8.17 LAB: Inventory (linked lists: insert at the front of a list) C++ Given main(), define an InsertAtFront() member function in the InventoryNode class that inserts items at the front of a linked list (after the dummy head node). Ex. If the input is: 4 plates 100 spoons 200 cups 150 forks 200 the output is: 200 forks 150 cups 200 spoons 100 plates InventoryNode.h #include #include using namespace std; class InventoryNode { private: string item; int numberOfItems; InventoryNode *nextNodeRef; public: //Constructor InventoryNode() { this->item = ""; this->numberOfItems = 0; this->nextNodeRef = NULL; } //Constructor InventoryNode(string itemInit, int numberOfItemsInit) { this->item = itemInit; this->numberOfItems = numberOfItemsInit; this->nextNodeRef = NULL; } //Constructor InventoryNode(string itemInit, int numberOfItemsInit, InventoryNode nextLoc) { this->item = itemInit; this->numberOfItems = numberOfItemsInit; this->nextNodeRef = &nextLoc; } // TODO: Define an insertAtFront() method that inserts a node at the // front of the linked list (after the dummy head node) //Get the next node InventoryNode *GetNext() { return this->nextNodeRef; } //Print node data void PrintNodeData() { cout << this->numberOfItems << " " << this->item << endl; } }; main.ccp #include "InventoryNode.h" int main() { int count; int numItems; string item; InventoryNode *headNode = new InventoryNode(); InventoryNode *currNode; // Obtain number of items cin >> count; // Get each item and number of each for (int i = 0; i < count; i++) { cin >> item; cin >> numItems; currNode = new InventoryNode(item, numItems); currNode->InsertAtFront(headNode, currNode); } // Print linked list currNode = headNode->GetNext(); while (currNode != NULL) { currNode->PrintNodeData(); currNode = currNode->GetNext(); } return 0; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
8.17 LAB: Inventory (linked lists: insert at the front of a list) C++
Given main(), define an InsertAtFront() member function in the InventoryNode class that inserts items at the front of a linked list (after the dummy head node).
Ex. If the input is:
4the output is:
200 forks#include <iostream>
#include <string>
using namespace std;
class InventoryNode {
private:
string item;
int numberOfItems;
InventoryNode *nextNodeRef;
public:
//Constructor
InventoryNode() {
this->item = "";
this->numberOfItems = 0;
this->nextNodeRef = NULL;
}
//Constructor
InventoryNode(string itemInit, int numberOfItemsInit) {
this->item = itemInit;
this->numberOfItems = numberOfItemsInit;
this->nextNodeRef = NULL;
}
//Constructor
InventoryNode(string itemInit, int numberOfItemsInit, InventoryNode nextLoc) {
this->item = itemInit;
this->numberOfItems = numberOfItemsInit;
this->nextNodeRef = &nextLoc;
}
// TODO: Define an insertAtFront() method that inserts a node at the
// front of the linked list (after the dummy head node)
//Get the next node
InventoryNode *GetNext() {
return this->nextNodeRef;
}
//Print node data
void PrintNodeData() {
cout << this->numberOfItems << " " << this->item << endl;
}
};
main.ccp
#include "InventoryNode.h"
int main() {
int count;
int numItems;
string item;
InventoryNode *headNode = new InventoryNode();
InventoryNode *currNode;
// Obtain number of items
cin >> count;
// Get each item and number of each
for (int i = 0; i < count; i++) {
cin >> item;
cin >> numItems;
currNode = new InventoryNode(item, numItems);
currNode->InsertAtFront(headNode, currNode);
}
// Print linked list
currNode = headNode->GetNext();
while (currNode != NULL) {
currNode->PrintNodeData();
currNode = currNode->GetNext();
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

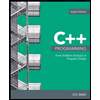
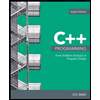