1. Write a program in C/C++ that receives N number of integer values from a user, stores the inputted values in a linked list, and performs some basic operations on the stored values. For simplicity, let’s assume that the user will always insert the values in ascending order. The program displays the user inputted values and prompt the user to provide an integer number (target value) to search in the linked list. If the target value does not appear in the list, the program inserts the value in an appropriate place according to the sorted (ascending) order. If the target value appears in the list, the program deletes the target value from the linked list. In both cases, the program displays the modified linked list. Your program must contain the following user-defined functions: - makeList – this function creates a linked list from the user inputted data. - printList – this function displays the entire linked list. - searchElement – this function receives the target value to search in the linked list and returns the location (address) of the target element in the linked list. - insertElement – this function inserts the target element in such a place so that the linked list will always be in ascending order. This function will only be executed if the target element does not appear in the linked list. - deleteElement – this function removes the target element from the linked list. This function will only be executed if the target element appears in the linked list.
1. Write a program in C/C++ that receives N number of integer values from a user, stores the inputted values in a linked list, and performs some basic operations on the stored values. For simplicity, let’s assume that the user will always insert the values in ascending order. The program displays the user inputted values and prompt the user to provide an integer number (target value) to search in the linked list. If the target value does not appear in the list, the program inserts the value in an appropriate place according to the sorted (ascending) order. If the target value appears in the list, the program deletes the target value from the linked list. In both cases, the program displays the modified linked list.
Your program must contain the following user-defined functions:
- makeList – this function creates a linked list from the user inputted data.
- printList – this function displays the entire linked list.
- searchElement – this function receives the target value to search in the linked list and returns the location (address) of the target element in the linked list.
- insertElement – this function inserts the target element in such a place so that the linked list will always be in ascending order. This function will only be executed if the target element does not appear in the linked list.
- deleteElement – this function removes the target element from the linked list. This function will only be executed if the target element appears in the linked list.

Step by step
Solved in 2 steps with 2 images

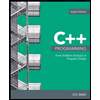
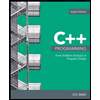