9.12: Element Shifter Write a function that accepts an int array and the array’s size as arguments. The function should create a new array that is one element larger than the argument array. The first element of the new array should be set to 0. Element 0 of the argument array should be copied to element 1 of the new array, element 1 of the argument array should be copied to element 2 of the new array, and so forth. The function should return a pointer to the new array. Demonstrate the function by using it in a main program that reads an integer N (that is not more than 50) from standard input and then reads N integers from a file named data into an array. The program then passes the array to your element shifter function, and prints the values of the new expanded and shifted array on standard output, one value per line. You may assume that the file data has at least N values. Prompts And Output Labels. There are no prompts for the integer and no labels for the reversed array that is printed out. Input Validation. If the integer read in from standard input exceeds 50 or is less than 0 the program terminates silently. I've typed up a code and it says it's correct from a "language point of view" but it has a "run-time error" and I'm not sure what this means. Can anyone help? I'll insert and image of the code I have type currently.
9.12: Element Shifter
Write a function that accepts an int array and the array’s size as arguments. The function should create a new array that is one element larger than the argument array. The first element of the new array should be set to 0. Element 0 of the argument array should be copied to element 1 of the new array, element 1 of the argument array should be copied to element 2 of the new array, and so forth. The function should return a pointer to the new array. Demonstrate the function by using it in a main
Prompts And Output Labels. There are no prompts for the integer and no labels for the reversed array that is printed out.
Input Validation. If the integer read in from standard input exceeds 50 or is less than 0 the program terminates silently.
I've typed up a code and it says it's correct from a "language point of view" but it has a "run-time error" and I'm not sure what this means. Can anyone help? I'll insert and image of the code I have type currently.
![WORK AREA
RESULTS
//Pass the array and size to function
58
int *s=elementShifter(array, count);
59
60
61
for (int i = 0; i <=count; i++)
62
{
63
64
65
cout << *s << endl;
s++;
67
68
69
70
}
return 0;
71
72 }
73
74 //Function definition
75
76 int *elementShifter(int arr[], int N)
77
78 {
79
80
int *newArr = new int[N + 1];
81
//First element set to zero
82
83
84
85
newArr[0] = 0;
for (int i
0; i < N; i++)
87
88
{
89
90
newArr[i+1]=arr[i];
91
92
93
94
95
96 }
}
return newArr;
LO O7c0 a O H NM S
O7 00 O 012 3456 r 00 o OHN M 4
n555 56664o 6666 6O77 7777 777 00 o co 0o 0o 0o co 00 co co aaaa oa
67 00 o 012 3456](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3f7b5fd3-a074-4788-8cbd-0dccf62c355e%2F6bc460b4-0e3d-489a-8123-4436786c4e3f%2F9aq8mut_processed.png&w=3840&q=75)
![WORK AREA
RESULTS
2 #include<iostream>
4 #include<fstream>
6 using namespace std;
7
8 int *elementShifter(int[], int);
9.
10 //main function
11
12 int main()
14 {
15
16
17
18
19
20
21
const int N = 50;
//Read N values from file
int array[N];
22
ifstream infile("data.txt");
23
if (!infile)
25
26
27
28
{
cout << "File not found....!!" << endl;
30
return -1;
31
32
}
33
34
35
int count
-1, data=0;
%3D
while (infile)
36
37
38
39
{
40
count++;
41
42
if (count < 0 || count >50)
return -1;
44
45
46
47
48
49
50
else
{
infile >> array[count];
51
}
53
54
55
56
}
//Pass the array and size to function
57
58
int *s=elementShifter(array, count);
59
345
C 00 O O123 4567 00
O H N 456 7 60
H H H - H 1 1 1122N NN 2 2222 233 m333 3333 4444444 44 n555 n555 55
N3456](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3f7b5fd3-a074-4788-8cbd-0dccf62c355e%2F6bc460b4-0e3d-489a-8123-4436786c4e3f%2F7xm59kp_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

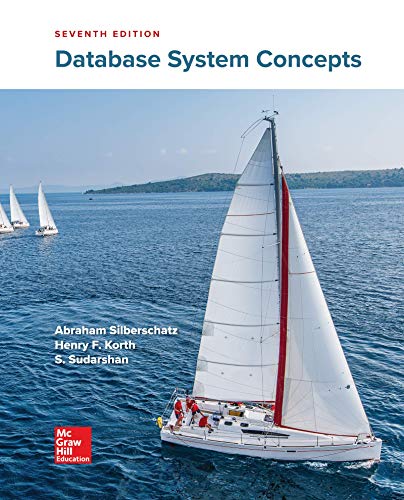
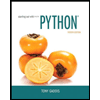
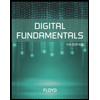
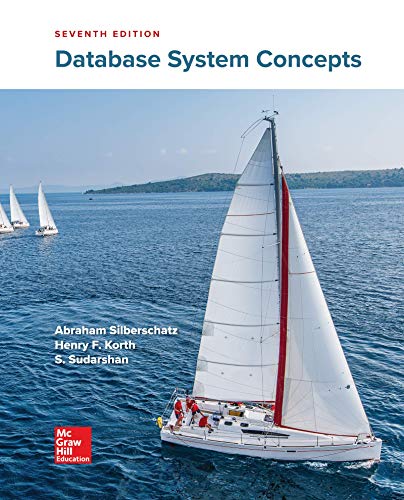
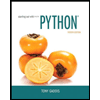
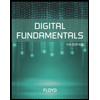
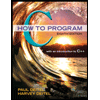
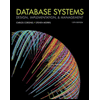
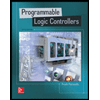