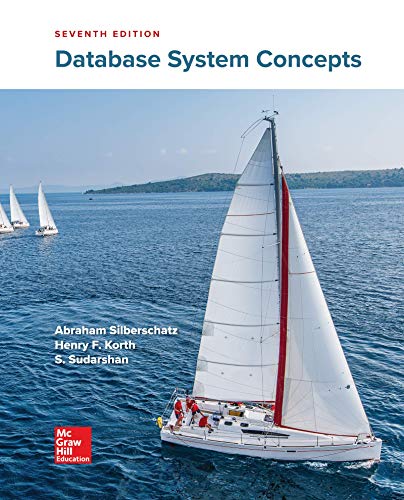

swap.h
void swap_by_value(int x,int y);
void swap_by_pointers(int *x,int *y);
void reverse_array(int x[],int n);
void cumulative_sum(int x[],int n);
swap.cpp
#include <iostream>
#include "swap.h"
void swap_by_value(int x,int y)
{
int temp=x;
x=y;
y=temp;
}
void swap_by_pointers(int *x,int *y)
{
int temp=*x;
*x=*y;
*y=temp;
}
void reverse_array(int x[],int n)
{
int i=0,j=n-1;
while(i<j)
{
int temp=x[i];
x[i]=x[j];
x[j]=temp;
i++;
j--;
}
}
void cumulative_sum(int x[],int n)
{
int sum=x[0];
for(int i=1;i<n;i++)
{
sum=sum+x[i];
x[i]=sum;
}
}
main.cpp
#include<iostream>
#include "swap.h"
using namespace std;
int main(int argc, char const *argv[])
{
int foo[5] = {1, 2, 3, 4, 5};
cout<<"Addresses of elements:"<<endl;
for(int i=0;i<5;i++)
{
cout<<&foo[i]<<" ";
}
cout<<endl;
cout<<"Elements:"<<endl;;
int *ptr=foo;
for(int i=0;i<5;i++)
{
cout<<*ptr<<" ";
ptr=ptr+1;
}
cout<<endl;
int a,b;
int f;
int flag = 1;
while(flag == 1)
{
cout<<"Enter indices of elements you want to swap?"<<endl;
cout<<"First index"<<endl;
cin>>a;
cout<<"Second index"<<endl;
cin>>b;
cout<<"Enter 0 for pass-by-value, 1 for pass-by-pointer"<<endl;
cin>>f;
switch (f)
{
case 0:
// Pass by Value
// Compare the resultant array with the array you get after passing by pointer
cout<<"Before swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
swap_by_value(foo[a],foo[b]);
cout<<"\nAfter swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
break;
case 1:
// Pass by pointer
cout<<"Before swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
swap_by_pointers(&foo[a],&foo[b]);
cout<<"\nAfter swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
break;
}
cout<<endl;
cout<<"Press 1 to continue else press 0"<<endl;
cin>>flag;
}
cout<<"Reversing the array";
// Reverse your array
reverse_array(foo,5);
cout<<"\nAfter reversing"<<endl;
for(int i = 0;i<5;i++){
cout<<foo[i]<<" ";
}
cout<<endl;
// GOLD problem
// Cumulative sum of the array
// TODO call this function cumulative_sum properly so that it passes the array 'foo'
// and updates 'foo' with cumulative summation.
// After this function call, you will get the updated array here
// TODO complete the function declaration in swap.h file
// TODO complete the function in swap.cpp file
cumulative_sum(foo,5);
cout<<"\nAfter cumulative summation"<<endl;
for(int i = 0;i<5;i++){
cout<<foo[i]<<" ";
}
cout<<endl;
return 0;
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- #include <iostream>using namespace std;double median(int *, int);int get_mode(int *, int);int *create_array(int);void getinfo(int *, int);void sort(int [], int);double average(int *, int);int getrange(int *,int);int main(){ int *dyn_array; int students; int mode,i,range;float avrg;do{cout << "How many students will you enter? ";cin >> students;}while ( students <= 0 );dyn_array = create_array( students );getinfo(dyn_array, students);cout<<"\nThe array is:\n";for(i=0;i<students;i++)cout<<"student "<<i+1<<" saw "<<*(dyn_array+i)<<" movies.\n";sort(dyn_array, students);cout << "\nthe median is "<<median(dyn_array, students) << endl;cout << "the average is "<<average(dyn_array, students) << endl;mode = get_mode(dyn_array, students);if (mode == -1)cout << "no mode.\n";elsecout << "The mode is " << mode << endl;cout<<"The range of movies seen is…arrow_forwardArrays write a C++ program that will declare two static arrays where each will hold the contents of a file (random.txt) of 200 integer values. Sorting Using the two arrays, call a private member function that uses the bubble sort algorithm to sort one of the arrays in ascending order. The function should count the number of exchanges it makes. Display this value. The program should then call a private member function that uses the selection sort algorithm to sort the other array. It should also count the number of exchanges it makes. Display this value. Searching Next, call a private member function that uses the linear search program to locate the value 869. The function should keep a count of the number of comparisons it makes until it finds the value. Display this value The program then should call a private member function that uses the binary search algorithm to locate the same value. It should also keep count of the numbers of comparisons it makes. Display this…arrow_forwardstruct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward
- Q# Describe what a data structure is for a char**? (e.g. char** argv) Group of answer choices A. It is a pointer to a single c-string. B. It is an array of c-strings C. It is the syntax for dereferencing a c-string and returning the value.arrow_forwardc++ language using addition, not get_sum function with this pseudocode: Function Main Declare Integer Array ages [ ] Declare integer total assign numbers = [ ] assign total = sum(ages) output "Sum: " & total end fucntion sum(Integer Array array) declare integer total declare integer index assign total = 0 for index = 0 to size(array) -1 assign total = total + array[ ] end return integer totalarrow_forward23. In the C++ language write a program to find the product of all the elements present in the array of integers given below. int numArr[5] = {16,4, 8, 7, 12};arrow_forward
- C++ languagearrow_forward#include <bits/stdc++.h>using namespace std;int main() { double matrix[4][3]={{2.5,3.2,6.0},{5.5, 7.5, 12.6},{11.25, 16.85, 13.45},{8.75, 35.65, 19.45}}; cout<<"Input no in first row of matrix"<<endl; for(int i=0;i<3;i++){ double t; cin>>t; matrix[0][i]=t; } cout<<"Contents of the last column in matrix"<<endl; for(int i=0;i<4;i++){ cout<<matrix[i][2]<<" "; } cout<<"Content of first row and last column element in matrix is: "<<matrix[0][3]<<endl; matrix[3][2]=13.6; cout<<"Updated matrix is :"<<endl; for(int i=0;i<4;i++){ for(int j=0;j<3;j++){ cout<<matrix[i][j]<<" "; }cout<<endl; } return 0;} Please explain this codearrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward
- C++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forwardStudent* func () { unique ptr arr[] make_unique ("CSC340") }; // #1 Insert Code int main () ( // #2 Insert Code [ #1 Insert Code]: Write code to keep all the object(s) which element(s) of array arr owns alive outside of the scope of func. [#2 Insert Code]: Write code to have a weak_ptr monitor the object which survived; Then test if it has any owner; Then properly destroy it; Then test again if has any owner; Then destroy the Control Block.arrow_forward#include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
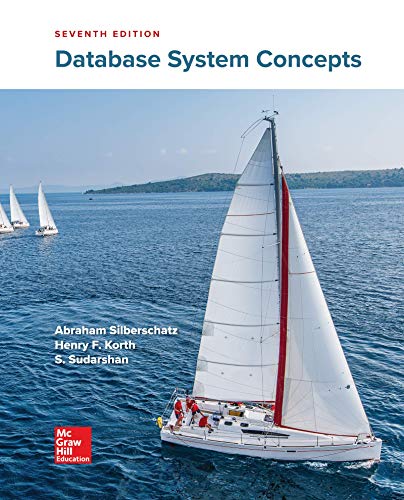
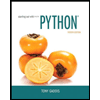
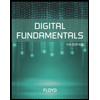
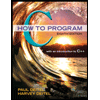
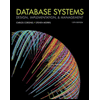
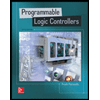