A chemistry teacher asked her class to answer the following question: Use java. If I put 10 bacteria on the classroom doorknob tonight, how many bacteria will be on the doorknob by the time we come to school tomorrow? In order to answer this question, you need to know a few things: Each bacterium (a single bacteria) can create 2 more bacteria every hour This means that after one hour, the doorknob will have 10 + 10 * 2 = 30 bacteria, since we started with 10 bacteria and each of those created 2 more. This means that after two hours, the doorknob will have 30 + 30 * 2 = 90, since there were 30 bacteria at the beginning of hour two, and during that hour each bacteria created 2 more bacteria. Continuing this pattern, the number of bacteria on the doorknob after N hours is numberOfBacteriaLastHour + numberOfBacteriaLastHour * 2 However, if we wanted to know how many bacteria are alive after 12 hours, we need to know how many bacteria were alive at hour 11. To find out how many bacteria are alive at hour 11, we need to figure out how many were alive at hour 10. Do you see how we can use recursion to solve this problem? Write a recursive function that computes the number of bacteria alive after N hours. The function signature is public int numBacteriaAlive(int hour)
A chemistry teacher asked her class to answer the following question: Use java. If I put 10 bacteria on the classroom doorknob tonight, how many bacteria will be on the doorknob by the time we come to school tomorrow? In order to answer this question, you need to know a few things: Each bacterium (a single bacteria) can create 2 more bacteria every hour This means that after one hour, the doorknob will have 10 + 10 * 2 = 30 bacteria, since we started with 10 bacteria and each of those created 2 more. This means that after two hours, the doorknob will have 30 + 30 * 2 = 90, since there were 30 bacteria at the beginning of hour two, and during that hour each bacteria created 2 more bacteria. Continuing this pattern, the number of bacteria on the doorknob after N hours is numberOfBacteriaLastHour + numberOfBacteriaLastHour * 2 However, if we wanted to know how many bacteria are alive after 12 hours, we need to know how many bacteria were alive at hour 11. To find out how many bacteria are alive at hour 11, we need to figure out how many were alive at hour 10. Do you see how we can use recursion to solve this problem? Write a recursive function that computes the number of bacteria alive after N hours. The function signature is public int numBacteriaAlive(int hour)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
A chemistry teacher asked her class to answer the following question: Use java.
If I put 10 bacteria on the classroom doorknob tonight, how many bacteria will be on the doorknob by the time we come to school tomorrow?
In order to answer this question, you need to know a few things:
- Each bacterium (a single bacteria) can create 2 more bacteria every hour
- This means that after one hour, the doorknob will have 10 + 10 * 2 = 30 bacteria, since we started with 10 bacteria and each of those created 2 more.
- This means that after two hours, the doorknob will have 30 + 30 * 2 = 90, since there were 30 bacteria at the beginning of hour two, and during that hour each bacteria created 2 more bacteria.
- Continuing this pattern, the number of bacteria on the doorknob after N hours is
numberOfBacteriaLastHour + numberOfBacteriaLastHour * 2 - However, if we wanted to know how many bacteria are alive after 12 hours, we need to know how many bacteria were alive at hour 11. To find out how many bacteria are alive at hour 11, we need to figure out how many were alive at hour 10. Do you see how we can use recursion to solve this problem?
Write a recursive function that computes the number of bacteria alive after N hours.
The function signature is
public int numBacteriaAlive(int hour)
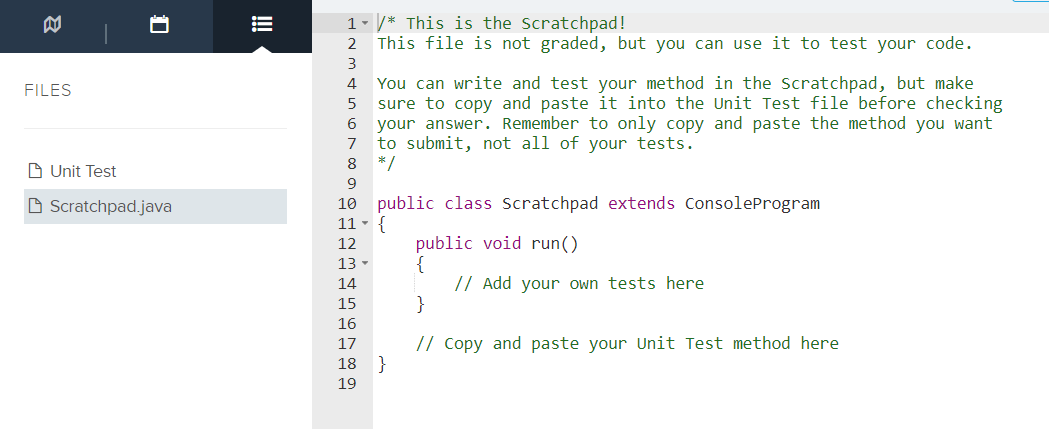
Transcribed Image Text:1 - /* This is the Scratchpad!
This file is not graded, but you can use it to test your code.
2
3
You can write and test your method in the Scratchpad, but make
sure to copy and paste it into the Unit Test file before checking
your answer. Remember to only copy and paste the method you want
to submit, not all of your tests.
*/
4
FILES
6
7
8
D Unit Test
9
O Scratchpad.java
10 public class Scratchpad extends ConsoleProgram
11 -
public void run()
{
// Add your own tests here
}
12
13 -
14
15
16
// Copy and paste your Unit Test method here
}
17
18
19
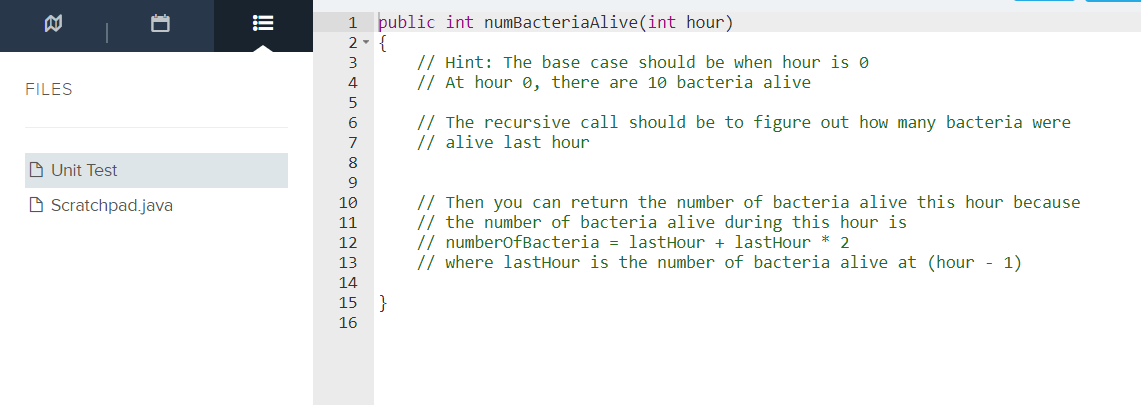
Transcribed Image Text:1 public int numBacteriaAlive(int hour)
2- {
// Hint: The base case should be when hour is 0
// At hour 0, there are 10 bacteria alive
4
FILES
5
// The recursive call should be to figure out how many bacteria were
// alive last hour
8
D Unit Test
9
// Then you can return the number of bacteria alive this hour because
// the number of bacteria alive during this hour is
// numberofBacteria = lastHour + lastHour * 2
// where lastHour is the number of bacteria alive at (hour - 1)
D Scratchpad.java
10
11
12
13
14
15
}
16
!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
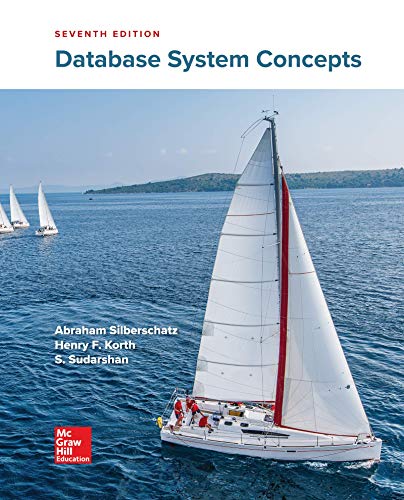
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
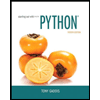
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
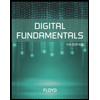
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
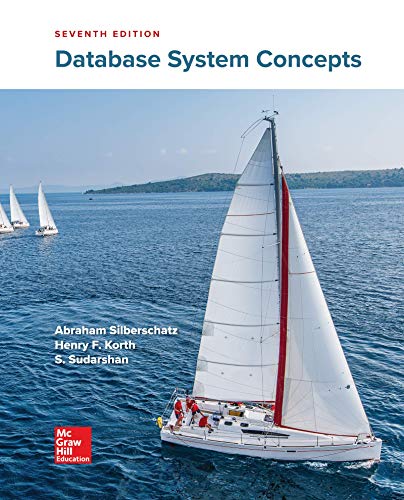
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
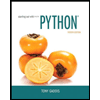
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
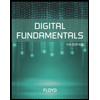
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
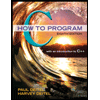
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
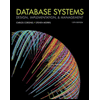
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
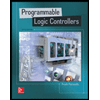
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education