in a java program. Imagine that you own a flower store and you need to display a menu of all the item categories, items and prices you sell for your customers. Display the prices for each product and category. The flowers are {"Rose", "Carnation", "Tulips", "Sunflowers"}, the price in order are {1.15, 1.5, 2.5, 2.75} the second category is vase sizes, {"Small", "Medium", "Large", "XLarge"}, the prices in order are {1.25, 2.5, 3.75, 4.5}. The last category is add ons, {"Teddy Bear" , "note", "ballon", "choclates"}. The prices are in order {10.0, 2.5, 5.75, 8.75} The list of products and the list of prices should be stores in 2 separate arrays. Create a method "display" that accepts 2 arrays as parameters and displays the product and the prices in receipt form. Create another method "sum" that displays the total for each category.
in a java program. Imagine that you own a flower store and you need to display a menu of all the item categories, items and prices you sell for your customers. Display the prices for each product and category. The flowers are {"Rose", "Carnation", "Tulips", "Sunflowers"}, the price in order are {1.15, 1.5, 2.5, 2.75}
the second category is vase sizes, {"Small", "Medium", "Large", "XLarge"}, the prices in order are {1.25, 2.5, 3.75, 4.5}.
The last category is add ons, {"Teddy Bear" , "note", "ballon", "choclates"}.
The prices are in order {10.0, 2.5, 5.75, 8.75}
The list of products and the list of prices should be stores in 2 separate arrays. Create a method "display" that accepts 2 arrays as parameters and displays the product and the prices in receipt form. Create another method "sum" that displays the total for each category.
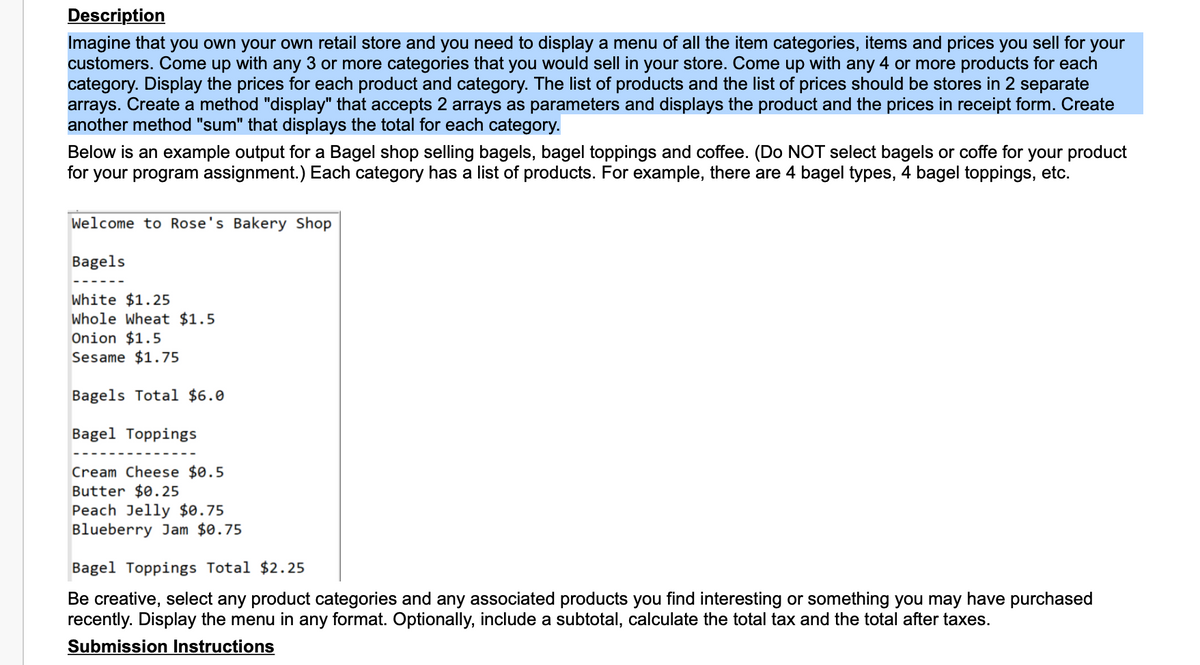

I have implemented the given requirement as per the specification. The code is as follows:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

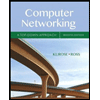
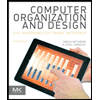
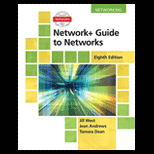
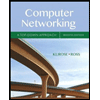
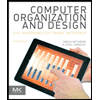
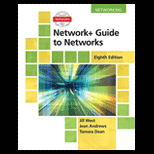
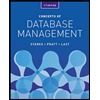
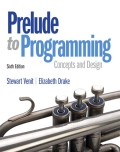
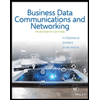