A client-server application for an Online-shopping Client side: (HTML, CSS) Write the code for the html. Ask the user to either login or create a new account: Login: Password Click here to create account -Create_account: If the user wants to create account , ask him/her to fill in registration form including his personal information , login ID, password and credit card number in order to create account for purchasing via online: Full Name: Address: Login Password Reype paword: Credit card Number: Create account Shopping.html must check (use javascript) that the full name can contain only alphabet and spaces, login can contain only alphabet, credit card number can be only digits. Assume initially, every credit card has a credit of 1000JD. When the user clicked on the "create account" button the data is sent to the shopping.php which in turn saves this information in the customer database. -Login_and_buy: when the user logged in using his/her login and password, he/she then can pick up (choose) as many products as he/she likes. When the user clicks on the button you must check if the user enters data or not, if either of the two fields is empty you must present an alert message and do not send the data to the server side. If the user entered login and password, then your program must call the shopping.php document. The user name must be autocomplete and the password must not be autocomplete You can choose the products you want in your project with at least five products. e.g. of products: "1kg suger pag", "tomato paste bottle", "tomato souce bottle", "sardine cans", " chilli sauce bottles", etc. When the customer finished selecting the products and pressed on the button buy, his/her request is sent to the server side shopping.php. e.g. Welcome to AlRadiadeh Shopping center Please choose the products you want and press buy * 2 1.2 JD Number of items 0.8 JD Number of items * 3 0.8 JD Number of items 0.75 JD Number of items Press to Buy In the above table the user bought 2 items of chilli sauce bottles (1.2 X 2 = 2.4 JD) and 3 items of sardine cans (0.8 X 3 = 2.4 JD). The total is 4.8. shopping.php will deduct 2.4 from his credit card balance. Server Side: Write the code of the server side shopping.php page such that. 1) The shopping.php checks using the regular expressions in PHP if the login is valid or not. A valid login must be exactly 5 characters and starts with a letter and followed by any one or more of the characters:_, $, 0-9, A-Z, a-z For instance, a12341, ab_$$1, and abc0Z_ are valid logins while _a1, b9993333*, and c23_@2 are invalid logins. But, there are no restrictions on the password; the user can use any character in the keyboard. 2) The shopping.php page reads the login and passwords from a database named "customers" to verify whether the customer is a valid user. The "customers" database contains two tables named "login_pssword" and “shopping cart”. The login_pssword table has three fields “customer_Id”,"login" and"password”, "credit balance". "credit balance" contains the remaining balance in the credit card. Initially, the "credit balance" for every user is 1000JD. Check if the user name and passwords exist. If it exists print the message "Thank you for logging in" and show him/her the products to start choosing. If the password or login is not in the database, display the alert message "wrong password and/or login please try again" and do not show him/her the products. The shopping_cart table has 3 fields: product_Id, product_name, product_price,
A client-server application for an
Online-shopping
- Client side: (HTML, CSS) Write the code for the html.
Ask the user to either login or create a new account:
Login:
Password
Click here to create account
-Create_account: If the user wants to create account , ask him/her to fill in registration form including his personal information , login ID, password and credit card number in order to create account for purchasing via online:
Full Name:
Address:
Login
Password
Reype paword:
Credit card Number:
|
Shopping.html must check (use javascript) that the full name can contain only alphabet and spaces, login can contain only alphabet, credit card number can be only digits. Assume initially, every credit card has a credit of 1000JD. When the user clicked on the "create account" button the data is sent to the shopping.php which in turn saves this information in the customer
-Login_and_buy: when the user logged in using his/her login and password, he/she then can pick up (choose) as many products as he/she likes. When the user clicks on the button you must check if the user enters data or not, if either of the two fields is empty you must present an alert message and do not send the data to the server side. If the user entered login and password, then your program must call the shopping.php document. The user name must be autocomplete and the password must not be autocomplete
You can choose the products you want in your project with at least five products. e.g. of products: "1kg suger pag", "tomato paste bottle", "tomato souce bottle", "sardine cans", " chilli sauce bottles", etc. When the customer finished selecting the products and pressed on the button buy, his/her request is
sent to the server side shopping.php. e.g.
Welcome to AlRadiadeh Shopping center Please choose the products you want and press buy |
||||||||||
1.2 JD Number of items |
0.8 JD Number of items |
0.8 JD Number of items |
||||||||
0.75 JD Number of items
|
|
|
||||||||
|
In the above table the user bought 2 items of chilli sauce bottles (1.2 X 2 = 2.4 JD) and 3 items of sardine cans (0.8 X 3 = 2.4 JD). The total is 4.8. shopping.php will deduct 2.4 from his credit card balance.
Server Side:
Write the code of the server side shopping.php page such that.
1) The shopping.php checks using the regular expressions in PHP if the login is valid or not. A valid login must be exactly 5 characters and starts with a letter and followed by any one or more of the characters:_, $, 0-9, A-Z, a-z For instance, a12341, ab_$$1, and abc0Z_ are valid logins
while _a1, b9993333*, and c23_@2 are invalid logins. But, there are no restrictions on the
password; the user can use any character in the keyboard.
2) The shopping.php page reads the login and passwords from a database named "customers" to verify whether the customer is a valid user. The "customers" database contains two tables named "login_pssword" and “shopping cart”. The login_pssword table has three fields “customer_Id”,"login" and"password”, "credit balance". "credit balance" contains the remaining balance in the credit card. Initially, the "credit balance" for every user is 1000JD.
Check if the user name and passwords exist. If it exists print the message "Thank you for logging in" and show him/her the products to start choosing. If the password or login is not in the database, display the alert message "wrong password and/or login please try again" and do not show him/her the products. The shopping_cart table has 3 fields: product_Id, product_name, product_price,

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

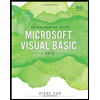
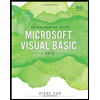