A CNC router has a tool which can be moved along three axes to carve shapes out of metal or wood with very fine precision. These devices use G-code statements to describe the tool's path (https://en.wikipedia.org/wiki/G-code). Let's imagine a simplified machine which can move in the xy-plane in integer increments. Instead of G-code, our instructions will consist of the letters 'L', 'R' to move in the T-direction and 'U', 'D’in the y-direction. Implement a function which accepts a std::string and computes the tool's displace- ment from its starting point. Its exact declaration should be: Point2d displacement(std::string command); As an example, the following command: Point2d p displacement("RU"); %3D P.print (); would result in (1,-1) being printed. The function should ignore any characters which are not one of the four command characters 'L’, 'R', 'U', 'D'. Check Canvas for the skeleton file robot_origin_hint.cpp, which contains test cases. YoL are only required to implement the displacement function.
A CNC router has a tool which can be moved along three axes to carve shapes out of metal or wood with very fine precision. These devices use G-code statements to describe the tool's path (https://en.wikipedia.org/wiki/G-code). Let's imagine a simplified machine which can move in the xy-plane in integer increments. Instead of G-code, our instructions will consist of the letters 'L', 'R' to move in the T-direction and 'U', 'D’in the y-direction. Implement a function which accepts a std::string and computes the tool's displace- ment from its starting point. Its exact declaration should be: Point2d displacement(std::string command); As an example, the following command: Point2d p displacement("RU"); %3D P.print (); would result in (1,-1) being printed. The function should ignore any characters which are not one of the four command characters 'L’, 'R', 'U', 'D'. Check Canvas for the skeleton file robot_origin_hint.cpp, which contains test cases. YoL are only required to implement the displacement function.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
#include
#include
#include
struct Point2d
{
double x;
double y;
void print()
{
// example: (2.5,3.64)
std::cout << "(" << x << "," << y << ")" << std::endl;
}
double length() const
{
return std::sqrt(x * x + y * y);
}
// const Point2D & ==> function can't modify 'other'
// the second const ==> function can't modify x, y
Point2d add(const Point2d &other) const
{
Point2d result;
result.x = x + other.x;
result.y = y + other.y;
return result;
}
};
//
// do NOT modify above this line
//
Point2d discplacement(std::string commands)
{
// TODO: re-write this
Point2d p = {0, 0};
return p;
}
//
// do NOT modify below this line
//
void check(Point2d result, double x , double y)
{
if (result.x != x || result.y != y)
{
std::cout << "fail" << std::endl;
}
else
{
std::cout << "pass" << std::endl;
}
}
int main()
{
Point2d result = discplacement("");
check(result, 0, 0);
result = discplacement("R");
check(result, 1, 0);
result = discplacement("D");
check(result, 0, 1);
result = discplacement("UDDUUDD");
check(result, 0, 1);
result = discplacement("UDUDLRLR");
check(result, 0, 0);
result = discplacement("DURL");
check(result, 0, 0);
std::cout << "done" << std::endl;
return 0;
above is robot_origin_hint.cop
C++language
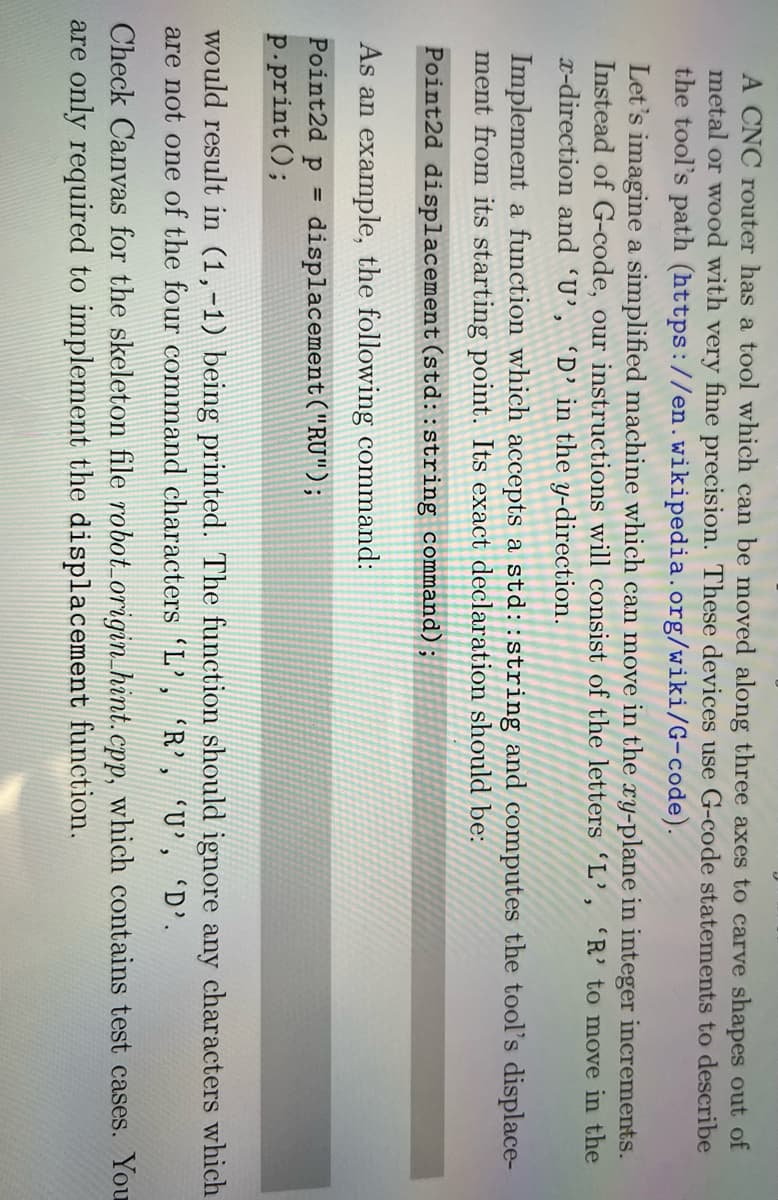
Transcribed Image Text:A CNC router has a tool which can be moved along three axes to carve shapes out of
metal or wood with very fine precision. These devices use G-code statements to describe
the tool's path (https://en.wikipedia.org/wiki/G-code).
Let's imagine a simplified machine which can move in the xy-plane in integer increments.
Instead of G-code, our instructions will consist of the letters 'L’, 'R' to move in the
T-direction and 'U', 'D’in the y-direction.
Implement a function which accepts a std::string and computes the tool's displace-
ment from its starting point. Its exact declaration should be:
Point2d displacement(std::string command);
As an example, the following command:
Point2d p
displacement("RU");
%3D
p.print ();
would result in (1,-1) being printed. The function should ignore any characters which
are not one of the four command characters 'L', 'R, U', 'D'.
Check Canvas for the skeleton file robot_origin_hint.cpp, which contains test cases. You
are only required to implement the displacement function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
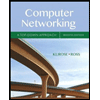
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
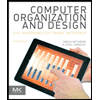
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
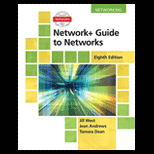
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
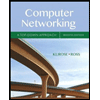
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
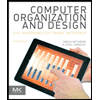
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
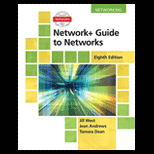
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
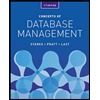
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
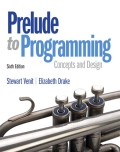
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
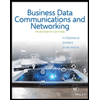
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY