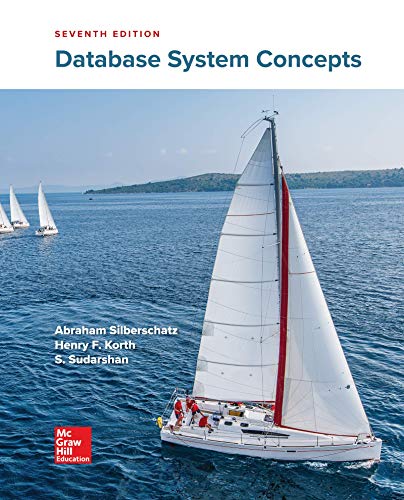
I am not able to get the ID, it jumps straight to GPA, where did i mess up? This is my code :
package com.company;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.Scanner;
class UniversityPersonnelManagement {
public static void main(String[] args) {
ArrayList<Student> studentList= new ArrayList<Student>();
ArrayList<Faculty> facultyList= new ArrayList<Faculty>();
System.out.println("Welcome to my Personal Management Program");
for(;;)
{
int input=0;
System.out.println(" Choose one of the options:");
System.out.println("Add a new Faculty member");
System.out.println("Add a new Student");
System.out.println("Print tuition invoice for a student");
System.out.println("Print information of a faculty");
System.out.println("Exit Program");
System.out.print(" Enter your selection: ");
Scanner s=new Scanner(System.in);
try{
input=s.nextInt();
}
catch (Exception e) {
System.out.println("Invalid entry- please try again");
}
if(input==1)
{
Faculty f=new Faculty();
System.out.println("Enter the faculty’s info:");
System.out.print("Name of the faculty:");
f.fullName= s.next();
System.out.print("ID:");
f.ID= s.next();
for(;;)
{
String rank;
System.out.print("Rank:");
rank=s.next();
if(rank.equalsIgnoreCase("professor")||rank.equalsIgnoreCase("adjunct"))
{
f.Rank=rank;
break;
}
else
{
System.out.print("Sorry entered rank (" +rank+") is invalid");
}
}
for(;;)
{
String department;
System.out.print("Department:");
department=s.next();
if(department.equalsIgnoreCase("mathematics")||department.equalsIgnoreCase("engineering")||department.equalsIgnoreCase("arts")||department.equalsIgnoreCase("science"))
{
f.Department=department;
break;
}
else
{
System.out.print("Sorry entered department (" +department+") is invalid");
}
}
System.out.println("Thanks!");
facultyList.add(f);
}
if(input==2)
{
Student st =new Student();
System.out.println("Enter the student’s info:");
System.out.print("Name of Student:");
st.fullName= s.next();
System.out.print("ID:");
st.ID= s.next();
System.out.print("Gpa:");
st.Gpa= s.nextDouble();
System.out.print("Credit hours:");
st.numberOfCreditHoursCurrentlyTaken= s.nextInt();
System.out.println("Thanks!");
studentList.add(st);
}
if(input==3)
{
System.out.print("Enter the student’s id:");
String id= s.next();
Student stnt=new Student();
Iterator<Student> si= studentList.iterator();
while (si.hasNext())
{
stnt= si.next();
if(stnt.ID==id)
{
break;
}
}
if(stnt.fullName==null)
{
System.out.print("Sorry-student not found!");
}
else
{
stnt.generateTuituonInvoice(stnt);
}
}
if(input==4)
{
System.out.print("Enter the faculty’s id: ");
String id= s.next();
Faculty ft=new Faculty();
Iterator<Faculty> si=facultyList.iterator();
while (si.hasNext())
{
ft= si.next();
if(ft.ID==id)
{
break;
}
}
if(ft.fullName==null)
{
System.out.print("Sorry-Faculty not found!");
}
else
{
System.out.println("Faculty found:");
System.out.println("--------------------------------------------------");
System.out.println(ft.fullName);
System.out.println(ft.Department+" Department, "+ft.Rank);
System.out.println("--------------------------------------------------");
}
}
if(input==5)
{
break;
}
}
}
}
class Faculty extends Person{
public String Department;
public String Rank;
public void add() {
// TODO Auto-generated method stub
}
public String getDepartment() {
return Department;
}
public void setDepartment(String department) {
Department = department;
}
public String getRank() {
return Rank;
}
public void setRank(String rank) {
Rank = rank;
}
}
class Student extends Person {
public double Gpa;
public int numberOfCreditHoursCurrentlyTaken;
public double getGpa() {
return Gpa;
}
public void setGpa(double gpa) {
Gpa = gpa;
}
public int getNumberOfCreditHoursCurrentlyTaken() {
return numberOfCreditHoursCurrentlyTaken;
}
public void setNumberOfCreditHoursCurrentlyTaken(int numberOfCreditHoursCurrentlyTaken) {
this.numberOfCreditHoursCurrentlyTaken = numberOfCreditHoursCurrentlyTaken;
}
public void add() {
}
public void generateTuituonInvoice(Student stnt)
{
System.out.println("Here is the tuition invoice for" +stnt.fullName+":");
System.out.println("--------------------------------------------------");
System.out.println(stnt.fullName+" "+ stnt.ID);
System.out.println("Credit Hours:"+stnt.numberOfCreditHoursCurrentlyTaken+"($236.45/credit hour)");
System.out.println("Fees: $52");
if(Gpa>=3.85)
{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.75;
double dis= (52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.25;
System.out.println("Total payment: $" +payment+"($"+dis+" discount applied)");
}
else{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45));
System.out.println("Total payment: $" +payment+"($0 discount applied)");
}
System.out.println("--------------------------------------------------");
}
}
abstract class Person {
public String fullName;
public String ID;
public abstract void add();
}

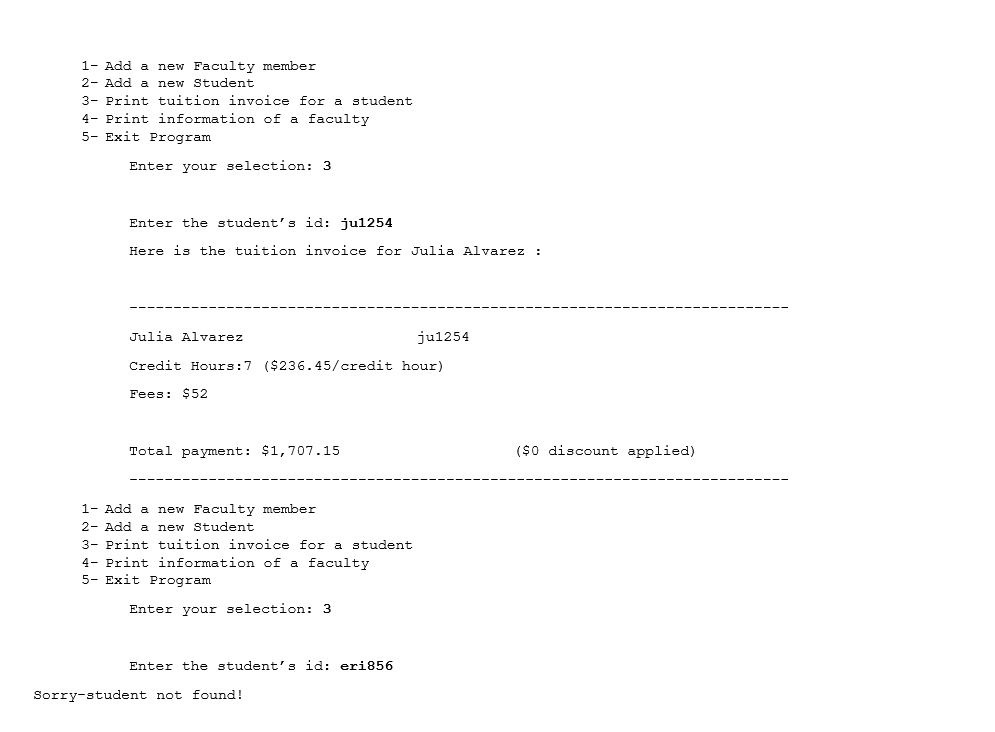

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Implement the Board class. Make sure to read through those comments so that you know what is required. import java.util.Arrays; import java.util.Random; public class Board { // You don't have to use these constants, but they do make your code easier to read public static final byte UR = 0; public static final byte R = 1; public static final byte DR = 2; public static final byte DL = 3; public static final byte L = 4; public static final byte UL = 5; // You need a random number generator in order to make random moves. Use rand below private static final Random rand = new Random(); private byte[][] board; /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. Note that making random moves * could result in the board being solved. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { // TODO } /** * Construct a puzzle board using a 2D array of bytes to indicate the contents *…arrow_forwardCreate the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forwardThis is in Java. The assignement is to create a class. I am confused on how to write de code for an array that is up to 5. Also I am confused regarding how to meet the requirements of the constructor. This is what I have. public class InventoryOnShelf { //fields private int[] itemOnShelfList []; private int size; public InventoryOnShelf() { }arrow_forward
- Use the Lottery class to the left as the superclass. Create the PowerBall class as a subclass of Lottery. Override the shuffle method so that it returns an ArrayList of six random integers up to but not including 100. CODE: import java.util.ArrayList;import java.util.Random; class Lottery { public ArrayList<Integer> shuffle() { Random r = new Random(); ArrayList<Integer> nums = new ArrayList<Integer>(); for (int i = 0; i < 5; i++) { int num = r.nextInt(20); nums.add(num); } return nums; }} //add class definitions below this line class PowerBall extends Lottery { public ArrayList<Integer> shuffle() { Random r = new Random(); ArrayList<Integer> nums = new ArrayList<Integer>(); for (int i = 0; i < 6; i++) { int num = r.nextInt(100); nums.add(num); } return nums; }} //add class definitions above this line public class Exercise1 { public static void main(String[] args) { //add code…arrow_forwardBuild a recursion tree for the following recurrence equation and then solve for T(n)arrow_forwardJava with screen shot pleasearrow_forward
- Please use the template provided below and make sure the output matches exactly. import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names. public static void printAllPermutations(ArrayList<String> permList, ArrayList<String> nameList) { } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ArrayList<String> nameList = new ArrayList<String>(); ArrayList<String> permList = new ArrayList<String>(); String name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardPRACTICE CODE import java.util.TimerTask;import org.firmata4j.ssd1306.MonochromeCanvas;import org.firmata4j.ssd1306.SSD1306;public class CountTask extends TimerTask {private int countValue = 10;private final SSD1306 theOledObject;public CountTask(SSD1306 aDisplayObject) {theOledObject = aDisplayObject;}@Overridepublic void run() {for (int j = 0; j <= 3; j++) {theOledObject.getCanvas().clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "Hello");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}theOledObject.clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "My name is ");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}while (true) {for (int i = 10; i >= 0; i--)…arrow_forward
- Write in Java What is this program's exact outputarrow_forwardneed help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
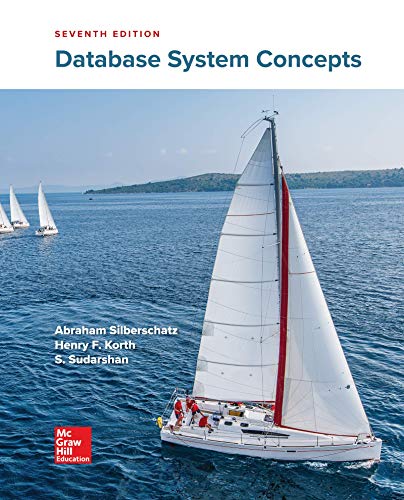
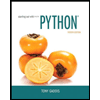
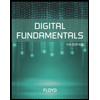
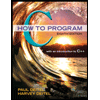
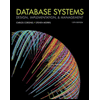
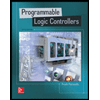