2- Add a new Student 3- Print tuition invoice for a student 4- Print information of a faculty 5- Exit Program Enter your selection: Enter the student's id: jul254 Here is the tuition invoice for Jones: Jones ma0258 Credit Hours:0($236.45/credit hour) Fees: $52 Total payment: $52.0($0 discount applied) Choose one of the options: 1- Add a new Faculty member 2- Add a new Student 3- Print tuition invoice for a student 4- Print information of a faculty 5- Exit Program Enter your selection: * 5: Debug un-to-date (3 200) E 6: TODO E Terminal Q Event 72.24 CRLE UTE.9 Aenarer 1- Add a new Faculty member 2- Add a new Student 3- Print tuition invoice for a student 4- Print information of a faculty 5- Exit Program Enter your selection: 3 Enter the student's id: jul254 Here is the tuition invoice for Julia Alvarez : Julia Alvarez jul254 Credit Hours:7 ($236.45/credit hour) Fees: $52 Total payment: $1,707.15 ($0 discount applied) 1- Add a new Faculty member 2- Add a new Student 3- Print tuition invoice for a student 4- Print information of a faculty 5- Exit Program Enter your selection: 3 Enter the student's id: eri856 Sorry-student not found!
Is there a particular reason why I do not get the right answer after I choose my option? Entering student ID ( that was previously entered ) and then displayed something different? This is the code :
import java.util.ArrayList;
import java.util.Iterator;
import java.util.Scanner;
class UniversityPersonnelManagement {
public static void main(String[] args) {
ArrayList<Student> studentList= new ArrayList<Student>();
ArrayList<Faculty> facultyList= new ArrayList<Faculty>();
System.out.println("Welcome to my Personal Management Program");
for(;;)
{
int input=0;
System.out.println(" Choose one of the options:");
System.out.println("1- Add a new Faculty member");
System.out.println("2- Add a new Student");
System.out.println("3- Print tuition invoice for a student");
System.out.println("4- Print information of a faculty");
System.out.println("5- Exit Program");
System.out.print(" Enter your selection: ");
Scanner s=new Scanner(System.in);
try{
input=s.nextInt();
}
catch (Exception e) {
System.out.println("Invalid entry- please try again");
}
if(input==1)
{
Faculty f=new Faculty();
System.out.println("Enter the faculty’s info:");
System.out.print("Name of the faculty:");
f.fullName= s.next();
System.out.print("ID:");
f.ID= s.next();
s.next();
for(;;)
{
String rank;
System.out.print("Rank:");
rank=s.next();
if(rank.equalsIgnoreCase("professor")||rank.equalsIgnoreCase("adjunct"))
{
f.Rank=rank;
break;
}
else
{
System.out.print("Sorry entered rank (" +rank+") is invalid");
}
}
for(;;)
{
String department;
System.out.print("Department:");
department=s.next();
if(department.equalsIgnoreCase("mathematics")||department.equalsIgnoreCase("engineering")||department.equalsIgnoreCase("arts")||department.equalsIgnoreCase("science"))
{
f.Department=department;
break;
}
else
{
System.out.print("Sorry entered department (" +department+") is invalid");
}
}
System.out.println("Thanks!");
facultyList.add(f);
}
if(input==2)
{
Student st =new Student();
System.out.println("Enter the student’s info:");
System.out.print("Name of Student:");
s.next(); // this is to clear the keyboard buffer
st.fullName= s.nextLine();
System.out.print("ID:");
st.ID= s.nextLine();
System.out.print("Gpa:");
st.Gpa= s.nextDouble();
System.out.print("Credit hours:");
st.numberOfCreditHoursCurrentlyTaken= s.nextInt();
System.out.println("Thanks!");
studentList.add(st);
}
if(input==3)
{
System.out.print("Enter the student’s id:");
String id= s.next();
Student stnt=new Student();
Iterator<Student> si= studentList.iterator();
while (si.hasNext())
{
stnt= si.next();
if(stnt.ID==id)
{
break;
}
}
if(stnt.fullName==null)
{
System.out.print("Sorry-student not found!");
}
else
{
stnt.generateTuituonInvoice(stnt);
}
}
if(input==4)
{
System.out.print("Enter the faculty’s id: ");
String id= s.next();
Faculty ft=new Faculty();
Iterator<Faculty> si=facultyList.iterator();
while (si.hasNext())
{
ft= si.next();
if(ft.ID==id)
{
break;
}
}
if(ft.fullName==null)
{
System.out.print("Sorry-Faculty not found!");
}
else
{
System.out.println("Faculty found:");
System.out.println("--------------------------------------------------");
System.out.println(ft.fullName);
System.out.println(ft.Department+" Department, "+ft.Rank);
System.out.println("--------------------------------------------------");
}
}
if(input==5)
{
break;
}
}
}
}
class Faculty extends Person{
public String Department;
public String Rank;
public void add() {
// TODO Auto-generated method stub
}
public String getDepartment() {
return Department;
}
public void setDepartment(String department) {
Department = department;
}
public String getRank() {
return Rank;
}
public void setRank(String rank) {
Rank = rank;
}
}
class Student extends Person {
public double Gpa;
public int numberOfCreditHoursCurrentlyTaken;
public double getGpa() {
return Gpa;
}
public void setGpa(double gpa) {
Gpa = gpa;
}
public int getNumberOfCreditHoursCurrentlyTaken() {
return numberOfCreditHoursCurrentlyTaken;
}
public void setNumberOfCreditHoursCurrentlyTaken(int numberOfCreditHoursCurrentlyTaken) {
this.numberOfCreditHoursCurrentlyTaken = numberOfCreditHoursCurrentlyTaken;
}
public void add() {
}
public void generateTuituonInvoice(Student stnt)
{
System.out.println("Here is the tuition invoice for" +stnt.fullName+":");
System.out.println("--------------------------------------------------");
System.out.println(stnt.fullName+" "+ stnt.ID);
System.out.println("Credit Hours:"+stnt.numberOfCreditHoursCurrentlyTaken+"($236.45/credit hour)");
System.out.println("Fees: $52");
if(Gpa>=3.85)
{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.75;
double dis= (52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.25;
System.out.println("Total payment: $" +payment+"($"+dis+" discount applied)");
}
else{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45));
System.out.println("Total payment: $" +payment+"($0 discount applied)");
}
System.out.println("--------------------------------------------------");
}
}
abstract class Person {
public String fullName;
public String ID;
public abstract void add();
}
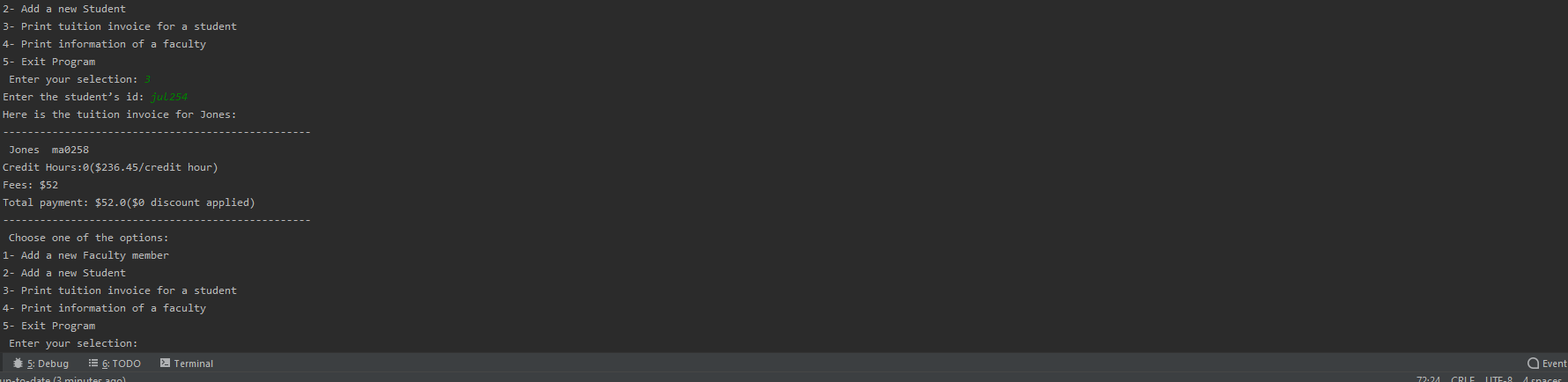
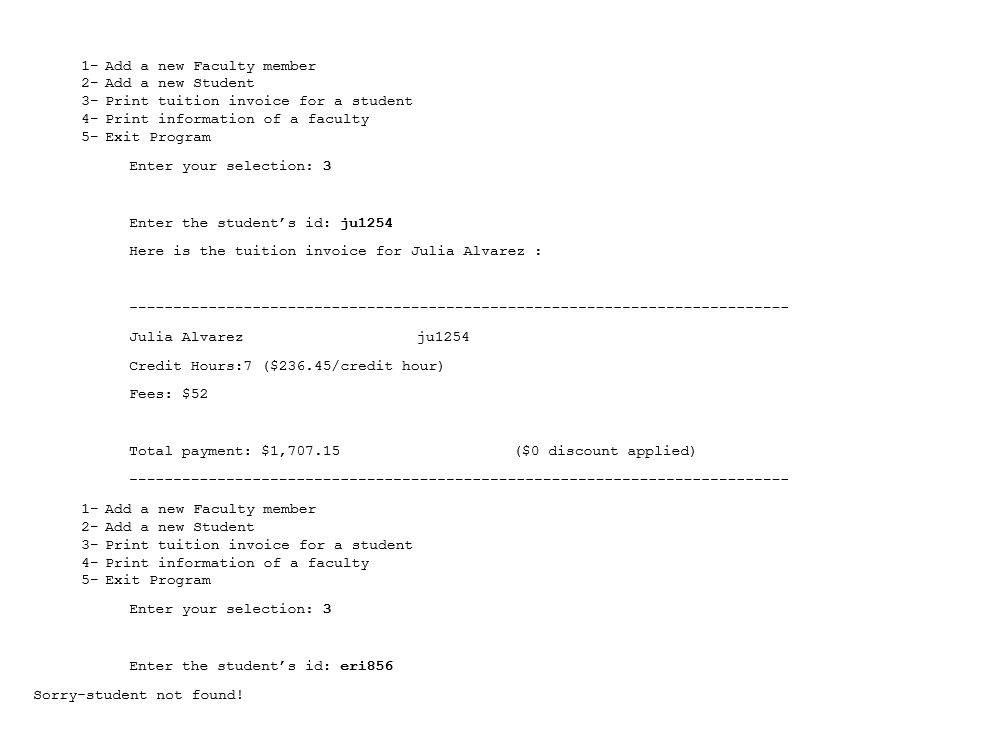

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

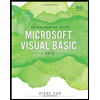
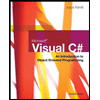
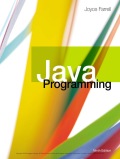
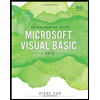
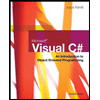
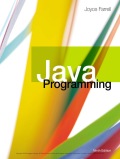