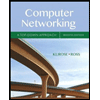
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
For this assignment, you need to implement link-based List and derivative ADTs in Java. To complete this, you will need the following:
- A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1.
- A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private.
- The attribute names for the Node and Linked List are the words in bold in #1 and #2.
- For the Linked List, implement the most common linked-list behaviors as explained in class -
- getters/setters/constructors/destructors for the attributes of the class,
- (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the list, (f) is list empty, (g) empty/destroy the list and (h) print all data items in a list. Note: create new list can be the constructor and destroy list can be the destructor or garbage collector. Note 2: It will be helpful if you define specific add/delete/find methods for the first and last position of the list.
- Any other methods you think would be useful in your program.
- A Stack class derived from the Linked List but with no additional attributes and the usual stack methods - (a) create stack, (b) push, (c) pop, (d) peek, and (e) destroy/empty the stack. Similar to above, create can be the constructor etc.
- A Queue class derived from the Linked List but with no additional attributes and the usual queue methods - (a) create queue, (b) enqueue, (c) dequeue, (d) peekFront, (e) peekRear, and (f) destroy/empty the queue. Similar to above, create can be the constructor etc.
- Ensure that all your classes are mimimal and cohesive with adequate walls around them. Try to reuse and not duplicate any code. You are allowed to define interfaces as needed, so you can reuse them in your project.
- Then write a main driver program that will demonstrate all the capabilities of your ADTs as follows -
- Create seven (7) Node objects of Money class to be used in the program.
- Demonstrate the use of your LinkedList with the seven USD Node objects inserted/deleted/searched in a random order. Remember, you will need to print the contents of the List several times to demonstrate the functionality.
- Demonstrate the use of your Stack with the same seven USD Node objects pushed/popped/peeked in a random order different from the one in LinkedList.
- Demonstrate the use of your Queue with the same seven USD Node objects enqueued/dequeued/peeked in a random order different from both the LinkedList and Stack.
- Demonstrate use of all the methods above by providing sufficient information to screen for actions being taken.
- Restrict all your input / output to the main driver program only.
- For submission, code your LinkNode and LinkedList in one file and Stack and Queue in a separate file. Remember to include the code file(s) for your Currency and Money classes. Also, your 'main' which is the driver program should be in a separate file of its own. Additionally, you will include your output text file as well as screenshots of the console run.
- Remember to include pre/post documentation and comment blocks as necessary in your code files.
The codes are stored in the following link, use them to complete the assignment: https://github.com/LunaMercer/Lab322C
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 1 images

Knowledge Booster
Similar questions
- For example: Programming generates and modifies linked lists: Typically, the software monitors two nodes: How to utilise the null reference in the node of a linked list in two common scenarios.arrow_forwardPart 1: autocomplete term. Write an immutable data type Term.java that represents an autocomplete term-a query string and an associated integer weight. You must implement the following API, which supports comparing terms by three different orders: lexicographic order by query string (the natural order); in descending order by weight (an alternate order); and lexicographic order by query string but using only the first r characters (a family of alternate orderings). The last order may seem a bit odd, but you will use it in Part 3 to find all query strings that start with a given prefix (of length r). public class Term implements Comparable { // Initializes a term with the given query string and weight. public Term (String query, long weight) // Compares the two terms in descending order by weight. public static Comparator byReverseWeight0rder () // Compares the two terms in lexicographic order, // but using only the first r characters of each query. public static Comparator…arrow_forwardFor the first two problems below, imagine the state of the linkedlist that would be created by the following code: ListNode asterisk = new ListNode('*') ; ListNode bang = new ListNode('!', asterisk); ListNode and = new ListNode('&', bang); ListNode percent = new ListNode('%', and); ListNode front = percent; Note that this ListNode class contains String values, but is otherwise identical to the ListNode class we have previously used that contained int values.arrow_forward
- Using java, write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference like (Java) or either a reference to the first node, as well as one to the last node. (Answer the following questions) 1) Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null. 2) Create a different method or function that iteratively explores the list, printing…arrow_forwardAssume that, you have a Linked List class "LL" with a function named "fun1". You can assume that "value" is the value of the nodes and "Next" is the next pointer of the node. You created an instance of the LL class, named "L1", where "head" is the head node. And you inserted the following numbers: 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91. What will be the output of the linked list L1 after the "fun1" operation to it?* 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 34 -> 57 31 -> 32 -> 43 -> 57 ->91 31 -> 32 -> 43 -> 34 -> 57 ->91arrow_forwardGiven the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student". typedef struct { char *name; char *major; } Student;typedef struct node { Student *data; struct node *next; struct node *prev; } Node;typedef struct { Node *header; int size; } LinkedList;LinkedList *roster; Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items). What TYPE of data is the following: *roster Group of answer choices int int * Node Node * Student Student * char char * This is legal code, but it does not match any of the types listed above. This is illegal code, and would produce a syntax error No hand written solution and no imagearrow_forward
- Design an implementation of an Abstract Data Type consisting of a set with the following operations: insert(S, x) Insert x into the set S. delete(S, x) Delete x fromthe set S. member(S, x) Return true if x ∈ S, false otherwise. position(S, x) Return the number of elements of S less than x. concatenate(S, T) Set S to the union of S and T, assuming every element in S is smaller than every element of T. All operations involving n-element sets are to take time O(log n).arrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forwardExplain the differences between a statically allocated array, a dynamically allocated array, and a linked list.arrow_forward
- Given the following specification of a front operation for queue:ItemType Front Function: Returns a copy of the front item on the queue. Precondition: Queue is not empty. Postcondition: Queue is not changed. 1. Write this operation as client code, using operations from the QueType class. (Remember,the client code has no access to the private variables of the class). 2. Write this function as a new member function of the QueType class. help me with complete codearrow_forwardIf you need to announce a Linked List Node structure for recording two data member with Name, Save Money, and a link pointer for next node in C language. The logical view is shown as attached figure. The requirement of data member record is following: Name is spelled by Character with maximal length 16; Save_Money is a positive integer number. Please filled the announcement statements for the Node of Linked List. struct Node { } node_Member; Save_Money; *next;arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
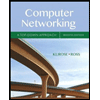
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
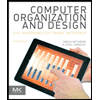
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
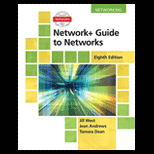
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
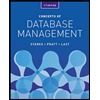
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
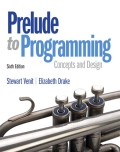
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
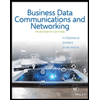
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY