A teacher has three exam grades for all the members of her class, and she worries that the grades are too low. You need to complete the method, named testAverage, in the class named Grades.java. There are two parameters to this method: the first is an integer representing the number of students in the class and, the second is a two-dimensional array of integers. In other words, each row represents the three grades for one student, while the columns represent the three sets of test grades. The return value should be the number of students with an average exam grade below seventy. The grades should be treated as double variables. For example, consider the grades for the four students in the following 2-D array, [ [88, 84, 89] [76, 64, 67] [85, 76, 79] [95, 90, 91] ] The first student has an exam average of 87.0 (= (88+84+89)/3 = 261/3), the second student has an exam average of 69.0 (= (77+64+67)/3 = 207/3), the third student has an exam average of 80.0 (= (85+76+79)/3 = 240/3), while the fourth student has an exam average of 92.0 (= (95+90+91)/3 = 276/3). This means that the number of students, in this group, that have an exam average less than 70.0 is only one. Complete the following file: public class Grades { /** This method examines the two-dimensional array of integers identified by the first parameter, whose length (number of rows, also the number of students) is the second parameter, while the three columns in the array represent the scores for three different tests. The test scores for each student (one row of three grades) are combined to find the average score for each student. The integer returned is the number of students with a test average less than 70. @param numStudents, the number of students who took the three tests @param theArray, a 2-D array of integer scores @return, the number of test averages below a score of 70 */ public static int testAverage(double[][] theArray, int numStudents) { int NUM_TESTS = 3; // your work here // loop though theArray, computing each student's average // your work here // return count of averages below 70.0 // your work here } }
A teacher has three exam grades for all the members of her class, and she worries that the grades are too low. You need to complete the method, named testAverage, in the class named Grades.java. There are two parameters to this method: the first is an integer representing the number of students in the class and, the second is a two-dimensional array of integers. In other words, each row represents the three grades for one student, while the columns represent the three sets of test grades. The return value should be the number of students with an average exam grade below seventy. The grades should be treated as double variables.
For example, consider the grades for the four students in the following 2-D array,
[ [88, 84, 89] [76, 64, 67] [85, 76, 79] [95, 90, 91] ]
The first student has an exam average of 87.0 (= (88+84+89)/3 = 261/3), the second student has an exam average of 69.0 (= (77+64+67)/3 = 207/3), the third student has an exam average of 80.0 (= (85+76+79)/3 = 240/3), while the fourth student has an exam average of 92.0 (= (95+90+91)/3 = 276/3). This means that the number of students, in this group, that have an exam average less than 70.0 is only one.
Complete the following file:
public class Grades
{
/**
This method examines the two-dimensional array of integers
identified by the first parameter, whose length (number of rows,
also the number of students) is the second parameter, while the
three columns in the array represent the scores for three
different tests.
The test scores for each student (one row of three grades) are
combined to find the average score for each student. The integer
returned is the number of students with a test average less than 70.
@param numStudents, the number of students who took the three tests
@param theArray, a 2-D array of integer scores
@return, the number of test averages below a score of 70
*/
public static int testAverage(double[][] theArray, int numStudents)
{
int NUM_TESTS = 3;
// your work here
// loop though theArray, computing each student's average
// your work here
// return count of averages below 70.0
// your work here
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

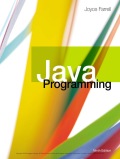
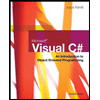
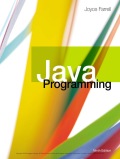
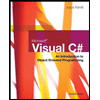