Write a program called Deleted_position.java that has a method with one int array as a parameter, and an integer position, where 0<=position<=a.length-1. The method should construct and return an array that is identical to the given array, but with the value in position deleted (Note that the resulting array should have length 1 less than the given array). The output is pictured below
Write a program called Deleted_position.java that has a method with one int array as a parameter, and an integer position, where 0<=position<=a.length-1. The method should construct and return an array that is identical to the given array, but with the value in position deleted (Note that the resulting array should have length 1 less than the given array). The output is pictured below
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a program called Deleted_position.java that has a method with one int array as a parameter, and an integer position, where 0<=position<=a.length-1. The method should construct and return an array that is identical to the given array, but with the value in position deleted (Note that the resulting array should have length 1 less than the given array).
The output is pictured below
![javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 0
Modified array is: [20, 30, 40, 50, 60, 100]
|→ javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 3
Modified array is: [10, 20, 30, 50, 60, 100]
|→ javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 4
Modified array is: [10, 20, 30, 40, 60, 100]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdb82eee3-c597-43b7-b98f-cffc18fbca72%2F73761a94-a030-4d97-adf2-bae1263383a8%2Fm9u50bh_processed.png&w=3840&q=75)
Transcribed Image Text:javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 0
Modified array is: [20, 30, 40, 50, 60, 100]
|→ javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 3
Modified array is: [10, 20, 30, 50, 60, 100]
|→ javalabs java Deleted_position
Given array:
[10, 20, 30, 40, 50, 60, 100]
Enter position of element to be deleted: 4
Modified array is: [10, 20, 30, 40, 60, 100]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
This code is incorrect. It does not match the output pictured in the original question. Please update the code to produce the exact output that is in the picture
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
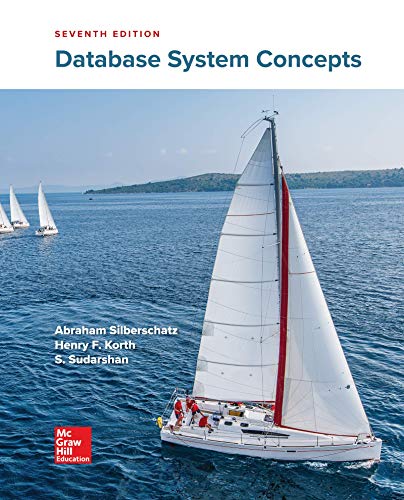
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
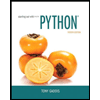
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
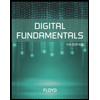
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
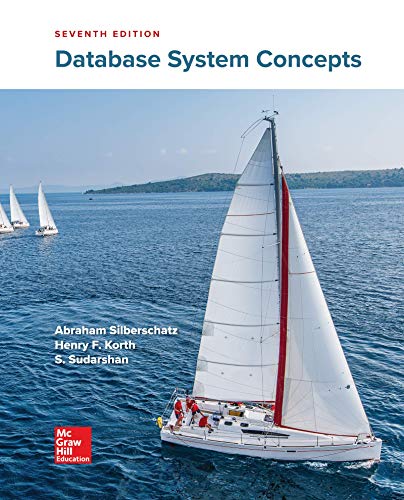
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
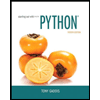
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
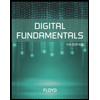
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
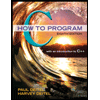
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
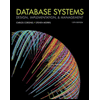
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
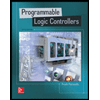
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education