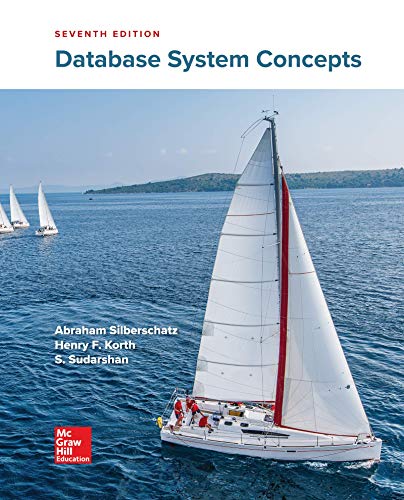
Write a Java program, called LinearSearch2, that contains a static method called countStrings() that accepts a 2D array of Strings and a String as its parameters. The method should return an integer value that is the total number of times the String being searched for is contained in the 2D String array. In the main method you should use user input to take the element you wish to search for in the array. There is code there for your array, DO NOT CHANGE THIS CODE. You should print an appropriate message to the screen if the element is found and how many times it exists in the array.
Note: The countStrings() method should work irrespective of case.
Sample Input 1
Duck
Sample Array 1
cat dog dUck rabbit hen duCk dUcK DucK goose
Sample Output 1
Duck is contained 4 times in the array
Sample Input 2
Opel
Sample Array 2
Volvo Fiat Suzuki Toyota fiat Suzuki Volvo Ferrari Ferrari Porsche Lamborghini Jag Jag Fiat Citroen Toyota
Sample Output 2
Opel was NOT found in the array

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Write a program named GradeStats.java then implement the following method: public static int[] gradeStats(int[] arr) It accepts int array representing test grades for CISY432 then calculates and returns the following grade statictics: highest, lowest, average, number of grades that are greater than or equal to 70, the number of grades that are less than 70 It returns these five numbers as an int array, and the 1st element is for highest, 2nd for lowest, 3rd for average 4th element for number of grades that are greater than or equal to 70 5th for the number of grades that are less than 70 For example. O Type here to search DELLarrow_forwardIn JAVAarrow_forwardIn java i have some code for a method that finds the index of an integer that is passed in thet int is (target) the method also has the array called (array) and the first and last element of the array. also for this method to work the array has to be in descending order "static int find(int [] array, int first, int last, int target)" thats the method with all the variables that are passed to it my guess is that the first if statement just will have a short line that is "return -1;" because since the array has to be in descending order the first element cant be greater then the last element in the array but im confused on the rest because i cant find a way to make them one line codes with the conditions that i have to meet in the comments any help would be aprreaciatedarrow_forward
- Write a program in java using two dimensional arrays. You should prompt the user toenter the number of rows and columns for your matrix. You should then prompt the userto enter values for each element of the matrix. Once the user has provided all the values for matrix, you should print the matrix and transpose of that matrix. A transpose of a matrix converts rows to columns and columns to rows.Example:Output: This program transposes a matrix.Output: Please enter the number of rows:User enters 2Output: Please enter the number of columns:User enters 3Enter value for row[0] column[0]: 9Enter value for row[0] column[1]: 1Enter value for row[0] column[2]: 2Enter value for row[1] column[0]: 72Enter value for row[1] column[1]: 3Enter value for row[1] column[2]: 6The matrix you entered is:9 1 272 3 6The transpose of this matrix has 3 rows and 2 columns and the transpose is:9 721 32 6arrow_forwardWrite a program called Range_array.java that has a method with one integer array and two integers position1 and position2 as parameters, where 0<=position1<=position2<=a.length-1. The method should construct and return an array that is identical to the given array, but with the values in position1 through position2 deleted. The output should look exactly like what is pictured below please. The code must be editable, and all variables defined within please.arrow_forwardIN JAVA Given an array, write a program to print the array elements one by one in a new line. If the array element is divisible by 4, print Four. If it is divisible by 6, print Six. If it is divisible by both 4 and 6, print Four Six. Use the following input array Int[] input_array = {2, 5, 10, 8, 18, 24, 3, 48, 30, 9, 12} Output should be 2 5 10 Four Six Four Six 3 Four Six Six 9 Four Sixarrow_forward
- For Java. Create a program that can read N integer numbers, store them in an array and then use a method to calculate and return the average of all numbers. Refer to photo.arrow_forwardWrite a program in java using two dimensional arrays. You should prompt the user toenter the number of rows and columns for your matrix. You should then prompt the userto enter values for each element of the matrix. Once the user has provided all the values for matrix, you should print the matrix and transpose of that matrix. A transpose of a matrix converts rows to columns and columns to rows.Example:Output: This program transposes a matrix.Output: Please enter the number of rows:User enters 2Output: Please enter the number of columns:User enters 3Enter value for row[0] column[0]: 9Enter value for row[0] column[1]: 1Enter value for row[0] column[2]: 2Enter value for row[1] column[0]: 72Enter value for row[1] column[1]: 3Enter value for row[1] column[2]: 6The matrix you entered is:9 1 272 3 6The transpose of this matrix has 3 rows and 2 columns and the transpose is:9 721 32 6arrow_forwardThis question is in javaYou are walking along a hiking trail. On this hiking trail, there is elevation marker at every kilometer. The elevation information is represented in an array of integers. For example, if the elevation array is [100, 50, 20, 30, 50, 40], that means at kilometer 0, the elevation is 100 meters; at kilometer 1, the elevation is 50 meters; at kilometer 2, the elevation is 20 meters; at kilometer 3, the elevation is 30 meters; at kilometer 4, the elevation is 50 meters; at kilometer 5, the elevation is 40 meters. a) Write a method called dangerousDescent that determines whether there is a downhill section in the hiking trail with a slope of more than -0.05. The slope is calculated by the rise in elevation over the run in distance. For example, the last kilometer section has a gradient of -0.01 because the rise in elevation is 40 - 50 = -10 meters. The distance covered in 1000 meters. -10 / 1000 = 0.01. Your method should return true if there exists a dangerous…arrow_forward
- In this project you will generate a poker hand containing five cards randomly selected from a deck of cards. The names of the cards are stored in a text string will be converted into an array. The array will be randomly sorted to "shuffle" the deck. Each time the user clicks a Deal button, the last five cards of the array will be removed, reducing the size of the deck size. When the size of the deck drops to zero, a new randomly sorted deck will be generated. A preview of the completed project with a randomly generated hand is shown in Figure 7-50.arrow_forwardThe programming language used is Javaarrow_forwardwrite the java programarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
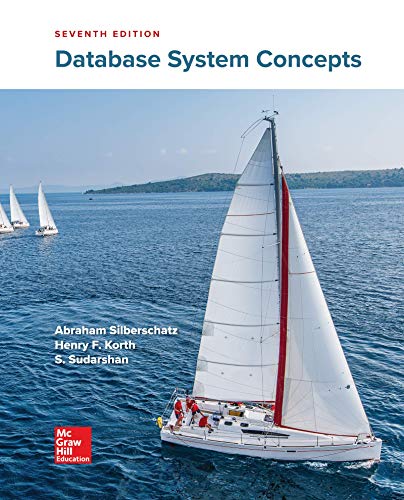
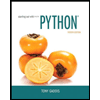
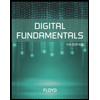
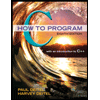
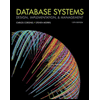
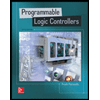