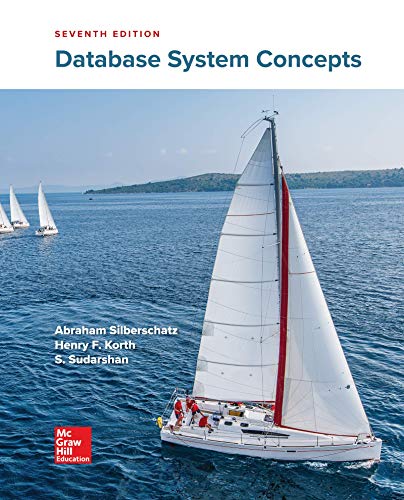
Concept explainers
2. Import Class
a) Implement a class Rectangle with two attributes, width and height. b) Implement an init method with an optional parameter type.
Set the default value of the attributes of width and height to 1.
c) Implement a display method to print the values of width and height.
d) Implement a setWidth method to assign width to the instance variable.
e) Implement a setHeight method to assign height to the instance variable.
f) Implement a getWidth method to return the value of the instance variable width.
g) Implement a getHeight method to return the value of the instance variable height.
h) Implement an area method to return the value of area of a rectangle.
i) Save Rectangle class as rectangle.py.
j) Import Rectangle class from rectangle.py.
k) Employs the Rectangle class methods above to set and get various measurements of a rectangle.
1) Instantiate two objects of type rectangle, one with arguments one without. r1 = Rectangle(4, 5)
r2 = Rectangle()
2) Call display() to print width and height.
3) Call area() in print() to display the area of r1 and r2.
4) Call setWidth() and setHeight() to update width and height to 6 of r2. 5) Call getWidth() in print() to display the updated width of r2.
6) Call getHeight() in print() to display the updated height of r2.
7) Call area() in print() to display the area of r2.

Step by stepSolved in 3 steps with 1 images

- a- Write a class definition for Student containing the following instance variables and methods: b- Create an object of this class c- Call the method to calculate and set average_test d- Print average test self. name self. test1 self. test2 self. average test A constructor to accept name, test1 and test2 as parameters and assign them to instant variables. A method to calculate and set averave test (each test worth 50%) A method to get average_testarrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forwardWrite a python code for both 1 and 2 use random inputs for each variablearrow_forward
- Make C# (Sharp): console project named MyPlayList. Create an Album class with 4 fields to keep track of title, artist, genre, and copies sold. Each field should have a get/set property. Your Album class should have a constructor to initialize the title (other 3 variables should default to null or 0). In addition, create a public method in the Album class to calculate amount sold for the album (copies sold * cost_of_album). You determine the cost of each album. Also create a method in the Album class to display the information. Print out of information should look something like this: The album Tattoo You by the Rolling Stones, a Rock group, made $25,000,000. In the main method, create 3 instances of the Album class with different albums, use setters to set values of the artist, genre, and copies sold. And then use getters to display information about each on the console.arrow_forward5. Declare a class called coordinate. This is to represent 3 dimensional Cartesian coordinates (x, y and z).Define the following methods cumulatively in Java cumulatively:a. Constructorb. Display, to print values of membersc. Add_coordinates, to add three such coordinate objects to produce a resultant coordinate object.Generate and handle exceptions if x, y and z coordinate of the result are zero.d. Main, to show use of the above methods.arrow_forward5. CODE IN PYTHON Create a BankAccount class which has an __init__ method which accepts an account name and starting balance to store as instance fields. The class should also have a method to deposit money, a method to withdraw money, and a method called "get_balance" which returns a string of the format "{account_name} has a balance of {balance}" (do not print this out from within the class, just return the string). In the same file, create an instance of the BankAccount class with a hardcoded name and starting balance. Deposit any amount to the account and withdraw any amount from the account (both can be hardcoded) and then print the result of calling the get_balance method on the instance.arrow_forward
- 1) Through BlueJ, the class must define a method called isSpace that allows the book club staff to determine whether there is enough capacity for a group to attend. This method must take a single integer parameter representing the size of the group, and return a boolean result. The method must work as follows: If the value of the parameter is less-than or equal-to 0 then the method must return false. This case has priority over those following. If the value of the parameter is less-than or equal-to the space left in the book club (use the capacity and occupancy values in the to work this out) then the method must return true. Otherwise (i.e., if there is not space in the book club for the whole group) then the method must return false. 2) This method must not change the state of the BookClub object. In other words, both the current number of occupants and the capacity of the club must be exactly the same after it is called as it was before (Note that the return type of this method…arrow_forward1)Create a complete Java class that can be used to create a Computer object as described below:A Computer has a:- Manufacturer- Disk Size- Manufacturing Date- Number of coresa) Add all instance variables (data encapsulation is expected).b) The class must have getters and setters for all instance variables.c) The class must have two constructors: a no-arg constructor and a constructor thatreceives input parameters for each instance variable.d) Implement a toString() method for your class. 2)Write a Java program TestComputer, which creates three Computer objects with the informationbelow:a) - Manufacturer: Dell- Disk Size: 1189160321024 bytes- Manufacturing Date: April 1, 2020- Number of cores: 2b) - Manufacturer: Apple Inc- Disk Size: 269283712040 bytes- Manufacturing Date: March 31, 2020- Number of cores: 4c) Create your own Object. You can mimic your actual personal computer or use a no-argsconstructor.Print the three objects using toString() method to show all data fieldsarrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- 1. Specify, design, and implement a "Note" class that can be used to hold information about a musical note. A programmer should be able to set and retrieve the length of the note and the value of the note. The length of a note may be a sixteenth note, eighth note, quarter note, half note, or whole note. A value is specified by indicating how far the note lies above or below the "A" note that orchestras use in tuning. In counting "how far," you should include both the white and black notes on a piano. For example, the note (value) numbers for the octave beginning at middle C are shown as integers here: -8 -6 C* D* Db Eb -3 -1 1 F* G* A* Gb Ab Bb CDEFGAB Write a complete Java program. The default constructor should set a note to a middle C quarter note. Include methods to set a note to a specified length and value. Write methods to retrieve information about a note, including methods to tell you: a) The letter of the note (A, B, C, ..., G) b) Whether the note is a natural (white key) or…arrow_forwardCreate an Account class that a bank might use to represent customers’ bank accounts. Include a data member to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it is greater than or equal to 0. If not, set the balance to 0. Provide three member functions:- credit($amount) should add amount to the current balance- debit($amount) should ensure that amount does not exceed the current balance and then reduce the current balance by amount. The balance should not change if amount exceeds the balance.- getBalance() should return the current balanceNote: Create a set of test data and then write a program to test the class.arrow_forwardg) Make a class Class (as in a class of students, not a class as in a class of objects) that stores several Student objects. Store a list of students in an attribute called students. The initializer method should initialize the class as an empty class (no students). Implement a method add_student() that takes an object of class Student and adds it to class. Implement method pass_rate() that determines the fraction of students that pass the class (have a grade of >= 6). python code!!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
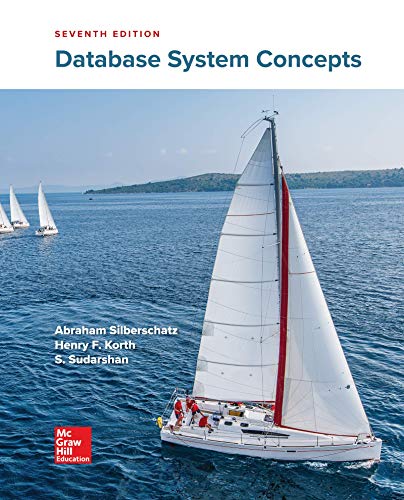
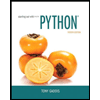
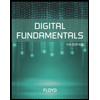
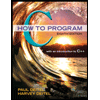
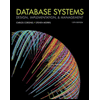
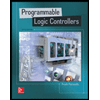