A. Write a Java Program that includes the following details You are tasked to develop a program to implement a simple Hospital Information System that will provide information about Hospitals such as their Doctors and Patient.
A. Write a Java Program that includes the following details
You are tasked to develop a program to implement a simple Hospital
provide information about Hospitals such as their Doctors and Patient.
1. Create a Hospital class and do the following:
a) Declare the following member variables: Hospital Id, and Hospital Name
b) Create a default constructor with no parameter.
c) Create a parameterized constructor to initialize all the member variables.
d) Create a method to display all the member variables.
2. Create a class Doctor and do the following:
a) The class should inherit the Hospital Class.
b) Declare a member variable Doctor id and Doctor salary.
c) Create a default constructor with no parameter
d) Create a parameterized constructor that will call the constructor of the parent class (super
class) and then initialize the member variables.
e) Create a method that will return a value to calculate the Doctor Salary by the Doctor using the
formula below:
Total Salary = Doctor Salary – (0.2 * Doctor Salary)
f) Create an overriding method to display all the member variables. The method should
invoke/call the super class display method and then display also all the member variables of
this class.
3. Create a class Patient and do the following:
a) The class should inherit the Hospital Class.
b) Declare a member variable Patient status and number of days
c) Create a default constructor with no parameter
d) Create a parameterized constructor that will call the constructor of the parent class (super
class) and then initialize the member variables.
e) Create a method that will return a value to determine the status of the patient based on the
criteria below:
When Patient status is equal to “in” and number of days is greater than or equal to 5
then the status of the patient is “Not good”.
Otherwise, the status of the patient is “OK”
The method should return the status of the patient.
f) Create an overriding method to display all the member variables. The method should
invoke/call the super class display method and then display also all the member variables of
this class.
4. Create a controlling class and do the following:
* Create an object for the two (2) subclasses
* Read the input, call the necessary methods, and display the required output as shown below.
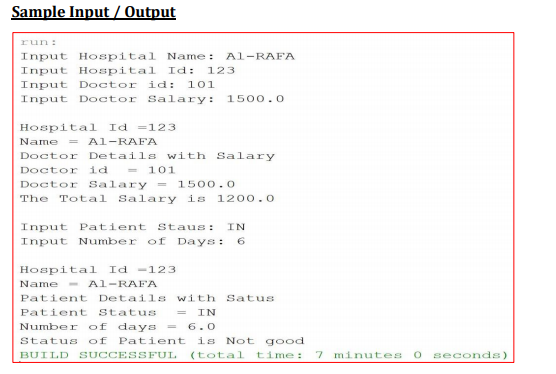

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

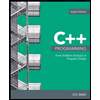
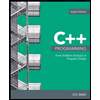