Add a constructor initializer list to the overloaded constructor Professor(string newName, int newStudents) to initialize name with newName and students with a vector of size given by newStudents. Ex: If the input is Jan 2 Ava Ada, then the output is: Professor: Unknown, No students Professor: Jan, Students: Ava, Ada #include #include using namespace std; class Professor { public: Professor(); Professor(string newName, int newStudents); void ReadStudents(); void Print() const; private: string name; vector students; }; Professor::Professor() : name("Unknown"), students(0) { } Professor::Professor(string newName, int newStudents) /* Your code goes here */ { } void Professor::ReadStudents() { string studentName; int i; for (i = 0; i < students.size(); ++i) { cin >> studentName; students.at(i) = studentName; } } void Professor::Print() const { int i; cout << "Professor: " << name << ", "; if (students.size() == 0) { cout << "No students" << endl; } else { cout << "Students: "; for (i = 0; i < students.size() - 1; ++i) { cout << students.at(i) << ", "; } cout << students.at(students.size() - 1) << endl; } } int main() { string name; int newStudents; Professor myProfessor; myProfessor.Print(); cin >> name; cin >> newStudents; Professor yourProfessor(name, newStudents); yourProfessor.ReadStudents(); yourProfessor.Print(); return 0; } c++ and please please make it correct
Add a constructor initializer list to the overloaded constructor Professor(string newName, int newStudents) to initialize name with newName and students with a
Ex: If the input is Jan 2 Ava Ada, then the output is:
Professor: Unknown, No students Professor: Jan, Students: Ava, Ada
#include <iostream>
#include <vector>
using namespace std;
class Professor {
public:
Professor();
Professor(string newName, int newStudents);
void ReadStudents();
void Print() const;
private:
string name;
vector<string> students;
};
Professor::Professor() : name("Unknown"), students(0) {
}
Professor::Professor(string newName, int newStudents) /* Your code goes here */ {
}
void Professor::ReadStudents() {
string studentName;
int i;
for (i = 0; i < students.size(); ++i) {
cin >> studentName;
students.at(i) = studentName;
}
}
void Professor::Print() const {
int i;
cout << "Professor: " << name << ", ";
if (students.size() == 0) {
cout << "No students" << endl;
}
else {
cout << "Students: ";
for (i = 0; i < students.size() - 1; ++i) {
cout << students.at(i) << ", ";
}
cout << students.at(students.size() - 1) << endl;
}
}
int main() {
string name;
int newStudents;
Professor myProfessor;
myProfessor.Print();
cin >> name;
cin >> newStudents;
Professor yourProfessor(name, newStudents);
yourProfessor.ReadStudents();
yourProfessor.Print();
return 0;
}
c++ and please please make it correct

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

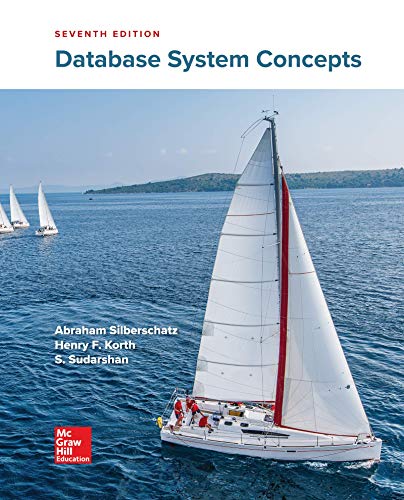
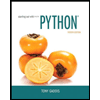
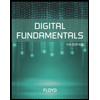
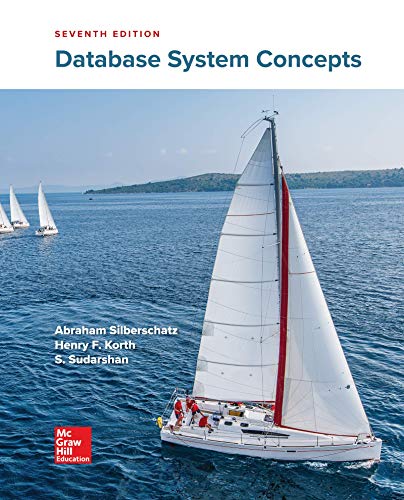
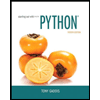
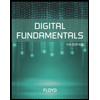
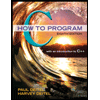
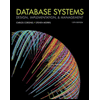
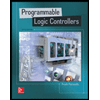