Add a method (call it spotterAnimalArea) to AnimalLab that takes two parameters — animalType and areaID — and returns a String containing the IDs of spotters that have seen the animal in that particular area. You should include only spotters who reported a sighting with count greater than zero. You should exclude duplicate spotter IDs.import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors;
Add a method (call it spotterAnimalArea) to AnimalLab that takes two parameters —
animalType and areaID — and returns a String containing the IDs of spotters that have
seen the animal in that particular area. You should include only spotters who reported a
sighting with count greater than zero. You should exclude duplicate spotter IDs.import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
*/
public class AnimalLab
{
private ArrayList<SightingRecord> records;
private ArrayList<String > dubID;
/**
* Create an AnimalLab.
*/
public AnimalLab()
{
records = new ArrayList<>();
addSightingRecords("records.csv");
}
/**
* Add the sightings recorded in the given filename to the current list.
* @param filename A CSV file of Sighting records.
*/
private void addSightingRecords(String filename)
{
SightingReader reader = new SightingReader();
records.addAll(reader.getSightings(filename));
}
/**
* Print details of all the sightings of the given animal.
* @param animal The type of animal.
*/
public void printSightingsOf(String animalType)
{
records.stream()
.filter(sighting -> animalType.equals(sighting.getAnimal()))
.forEach(sighting -> System.out.println(sighting.getDetails()));
}
/**
* Return a count of the specific animal reported in the given time period.
* @param animal The type of animal.
* @param timePeriod The time period.
* @return The sum of sightings.
*/
/**
* You should include only spotters who reported a
sighting with count greater than zero. You should exclude duplicate spotter IDs
returns a String containing the IDs of spotters that have
seen the animal in that particular area
*/
public int getSightingSum(String animal, int timePeriod)
{
{
return records.stream()
.filter(sighting -> animal.equals(sighting.getAnimal()))
.filter(sighting -> sighting.getPeriod() == timePeriod)
.filter(sighting -> sighting.getCount() > 0)
.map(sighting -> sighting.getSpotter() )
.reduce(0, (runningSum, count) -> runningSum + count);
}
}
public String spotterAnimalArea(String animalType, int areID)
{
return records.stream()
.filter(sighting -> animalType.equals(sighting.getAnimal()))
.filter(sighting -> sighting.getArea() == areID)
.map(sighting -> (sighting.getSpotter()))
.reduce("", (sum, b) -> sum + b);
}
}
++++++++++++++
/**
* Details of a sighting of a type of animal by an individual spotter.
*
public class SightingRecord
{
// The animal spotted.
private final String recordedAnimal;
// The ID of the spotter.
private final int spotterID;
// How many were seen.
private final int animalCount;
// The ID of the area in which they were seen.
private final int areaID;
// The reporting period.
private final int period;
/**
* Create a record of a sighting of a particular type of animal.
* @param animal The animal spotted.
* @param spotter The ID of the spotter.
* @param count How many were seen (>= 0).
* @param area The ID of the area in which they were seen.
* @param period The reporting period.
*/
public SightingRecord(String animal, int spotter, int count, int area, int period)
{
recordedAnimal = animal;
spotterID = spotter;
animalCount = count;
areaID = area;
this.period = period;
}
/**
* Return the type of animal spotted.
* @return The animal type.
*/
public String getAnimal()
{
return recordedAnimal;
}
/**
* Return the ID of the spotter.
* @return The spotter's ID.
*/
public int getSpotter()
{
return spotterID;
}
/**
* Return how many were spotted.
* @return The number seen.
*/
public int getCount()
{
return animalCount;
}
/**
* Return the ID of the area in which they were seen.
* @return Where they were seen.
*/
public int getArea()
{
return areaID;
}
/**
* Return the period in which they were seen.
* @return When they were seen.
*/
public int getPeriod()
{
return period;
}
/**
* Return a string containing details of the animal, the number seen,
* where they were seen, who spotted them and when.
* @return A string giving details of the sighting.
*/
public String getDetails()
{
return recordedAnimal +
", count = " + animalCount +
", area = " + areaID +
", spotter = " + spotterID +
", period = " + period;
}
}
JAVA

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

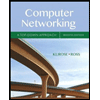
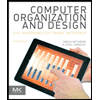
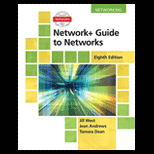
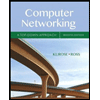
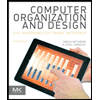
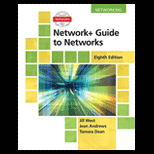
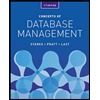
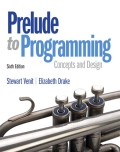
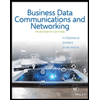