Write a static method called "flip4" that takes an ArrayList of integer values as a parameter and that rewrites successive sequences of four values in the list. For each sequence of four values, the method will swap the first element and the fourth element and then swap the second element with the third element. For example, suppose that a variable called list stores the following sequence of values: [3, 8, 19, 42, 7, 26, 19, -8, 193, 204, 6, -4] and we make the following call: flip4(list); Afterwards the list should store the following sequence of values: [42, 19, 8, 3, -8, 19, 26, 7, -4, 6, 204, 193] Each sequence of three elements will be rotated so that the 3rd value becomes the 1st value and the other two are moved to the next higher index; ie. the 1st becomes the 2nd and the 2nd becomes the 3rd. If the list has extra values that are not part of a sequence of three, those values are unchanged. For example, if the list had instead stored: [3, 8, 19, 42, 7, 26, 19, -8, 193, 204, 6, -4, 99, 2, 1] The result would have been: [42, 19, 8, 3, -8, 19, 26, 7, -4, 6, 204, 193, 99, 2, 1] Notice that the values (99, 2, 1) are unchanged in position because they were not part of a sequence of four values. You may use the following code to test your program: Expected output is listed to the right of the print statements. import java.util.*; public class Flipper { public static void main(String[] args) { ArrayList list = new ArrayList(); list.add(3); list.add(8); list.add(19); list.add(42); list.add(7); list.add(26); list.add(19); list.add(-8); list.add(193); list.add(99); list.add(2); System.out.println(list); // [3, 8, 19, 42, 7, 26, 19, -8, 193, 99, 2] flip4(list); System.out.println(list); // [42, 19, 8, 3, -8, 19, 26, 7, 193, 99, 2] } // *** Your method code goes here *** } // End of Flipper class
Write a static method called "flip4" that takes an ArrayList of integer values as a parameter and that rewrites successive sequences of four values in the list. For each sequence of four values, the method will swap the first element and the fourth element and then swap the second element with the third element. For example, suppose that a variable called list stores the following sequence of values:
[3, 8, 19, 42, 7, 26, 19, -8, 193, 204, 6, -4]
and we make the following call: flip4(list);
Afterwards the list should store the following sequence of values:
[42, 19, 8, 3, -8, 19, 26, 7, -4, 6, 204, 193]
Each sequence of three elements will be rotated so that the 3rd value becomes the 1st value and the other two are moved to the next higher index; ie. the 1st becomes the 2nd and the 2nd becomes the 3rd. If the list has extra values that are not part of a sequence of three, those values are unchanged. For example, if the list had instead stored:
[3, 8, 19, 42, 7, 26, 19, -8, 193, 204, 6, -4, 99, 2, 1]
The result would have been:
[42, 19, 8, 3, -8, 19, 26, 7, -4, 6, 204, 193, 99, 2, 1]
Notice that the values (99, 2, 1) are unchanged in position because they were not part of a sequence of four values.
You may use the following code to test your program:
Expected output is listed to the right of the print statements.
import java.util.*;
public class Flipper {
public static void main(String[] args) {
ArrayList<Integer> list = new ArrayList<Integer>();
list.add(3);
list.add(8);
list.add(19);
list.add(42);
list.add(7);
list.add(26);
list.add(19);
list.add(-8);
list.add(193);
list.add(99);
list.add(2);
System.out.println(list); // [3, 8, 19, 42, 7, 26, 19, -8, 193, 99, 2]
flip4(list);
System.out.println(list); // [42, 19, 8, 3, -8, 19, 26, 7, 193, 99, 2]
}
// *** Your method code goes here ***
} // End of Flipper class

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

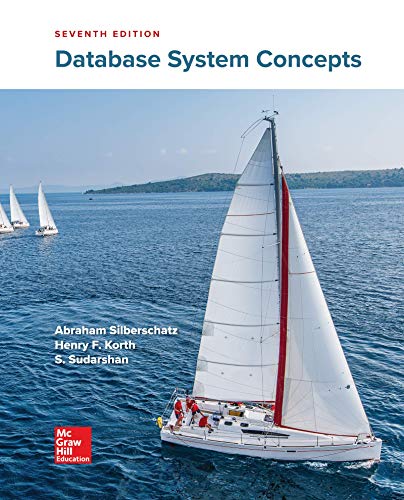
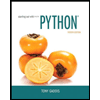
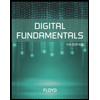
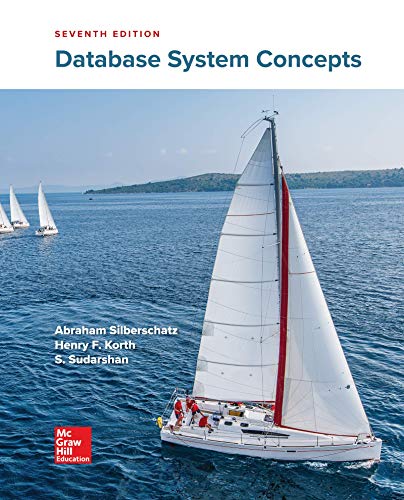
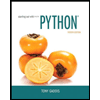
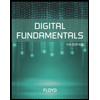
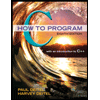
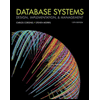
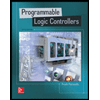