Add comments as appropriate. Be sure that your program output is neatly presented to the user. Add documentation comments to your functions. You are going to change the class you created in Program7 so that it has a constructor and some properties. In your GeoPoint class make the following changes (note your variable, parameter and method names may be different. Adjust as needed.): Add a constructor __init__(self, lat=0, lon=0,description = ‘TBD’) that will initialize the class variables __lat ,__lon and the __description. Notice that the constructor will also default lat and lon to zero and description to ‘TBD’ if they are not provided. Change the SetPoint method so that instead of individual coordinates SetPoint(self, lat, lon) it takes a single sequence. Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for points. Add another property: Description = property(GetDescription, SetDescription). Make sure GetDescription and SetDescription are the names you used for the get and set methods you already wrote. Change the distance method so that it takes a point instead of coordinates: Distance(self, toPoint) instead of Distance(self, lat, lon). You will put your class in a separate library file. You will use an #include statement from the main part of your program. In the main part of your program do the following: Include the class from the library file. Instantiate two points Instead of using SetPoint and SetDescription methods to set each of the points locations and descriptions do the following: Use the constructor to set point1 coordinates and description. It should look something like the following but with your own coordinates and description: point1 = GeoPoint(12.3456,-123.4567,'Loc1') Use a constructor without any arguments to instantiate the second point and use it’s properties to set it’s values. It should look something like the following but with your own coordinates and description: point2 = GeoPoint() point2.PointCoords = 23.4567, -213.456 point2.PointDescription = 'Loc2' Inside the “Do another (y/n)?” loop do the following: Ask the user for their location. Create a third point to represent the user’s location. You can use either the constructor or properties. With a constructor it will look like: pointUser = GeoPoint(lat,lon, 'User Location') Use point1 and point2’s Distance method to find the distance from each point to the user’s location. Notice we are passing in the users point rather than the separate coordinates: distanceToOne = point1.Distance(pointUser) distanceToTwo = point2.Distance(pointUser) Tell the user which point they are closest to in this format: You are closest to which is located at Ask “Do another (y/n)?” and loop if they respond with ‘y’
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
- Add comments as appropriate. Be sure that your program output is neatly presented to the user. Add documentation comments to your functions.
- You are going to change the class you created in Program7 so that it has a constructor and some properties.
- In your GeoPoint class make the following changes (note your variable, parameter and method names may be different. Adjust as needed.):
- Add a constructor __init__(self, lat=0, lon=0,description = ‘TBD’) that will initialize the class variables __lat ,__lon and the __description. Notice that the constructor will also default lat and lon to zero and description to ‘TBD’ if they are not provided.
- Change the SetPoint method so that instead of individual coordinates SetPoint(self, lat, lon) it takes a single sequence.
- Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for points.
- Add another property: Description = property(GetDescription, SetDescription). Make sure GetDescription and SetDescription are the names you used for the get and set methods you already wrote.
- Change the distance method so that it takes a point instead of coordinates: Distance(self, toPoint) instead of Distance(self, lat, lon).
- You will put your class in a separate library file. You will use an #include statement from the main part of your program.
- In the main part of your program do the following:
- Include the class from the library file.
- Instantiate two points
- Instead of using SetPoint and SetDescription methods to set each of the points locations and descriptions do the following:
- Use the constructor to set point1 coordinates and description. It should look something like the following but with your own coordinates and description:
point1 = GeoPoint(12.3456,-123.4567,'Loc1')
- Use a constructor without any arguments to instantiate the second point and use it’s properties to set it’s values. It should look something like the following but with your own coordinates and description:
point2 = GeoPoint()
point2.PointCoords = 23.4567, -213.456
point2.PointDescription = 'Loc2'
- Inside the “Do another (y/n)?” loop do the following:
- Ask the user for their location.
- Create a third point to represent the user’s location. You can use either the constructor or properties. With a constructor it will look like:
pointUser = GeoPoint(lat,lon, 'User Location')
- Use point1 and point2’s Distance method to find the distance from each point to the user’s location. Notice we are passing in the users point rather than the separate coordinates:
distanceToOne = point1.Distance(pointUser)
distanceToTwo = point2.Distance(pointUser)
- Tell the user which point they are closest to in this format:
You are closest to <description> which is located at <point’s x, y, z coordinates>
- Ask “Do another (y/n)?” and loop if they respond with ‘y’

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

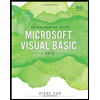
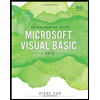