add public method size() to the class Stack so the output will be pop is: 4 peek is: 8 size is: 2 false public class Stack { private LinkedList list; public Stack() { list = new LinkedList<>(); } public boolean isEmpty() { return list.isEmpty(); } public void push(T data) { list.prepend(data); } public T pop() { if (isEmpty()) { throw new EmptyStackException(); } return list.removeHead(); } public T peek() { if (isEmpty()) { throw new EmptyStackException(); } return list.getHead(); } } public class Main { public static void main(String[] args) { Stack stack = new Stack<>(); stack.push(6); stack.push(8); stack.push(4); System.out.println("pop is: " + stack.pop()); System.out.println("peek is: " + stack.peek()); System.out.println("size is: " + stack.size()); System.out.println(stack.isEmpty()); } } public class LinkedList { private Node head; private Node tail; private int size; public LinkedList() { head = null; tail = null; size = 0; } public boolean isEmpty() { return size == 0; } public void append(T data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { tail.next = newNode; } tail = newNode; size++; } public void prepend(T data) { Node newNode = new Node(data); if (head == null) { head = newNode; tail = newNode; } else { newNode.next = head; head = newNode; } size++; } public T getHead() { return head.data; } public T getTail() { return tail.data; } public int size() { return size; } public void removeByValue(T data) { Node current = head; while (current.next!= null && current.next.data!= data) { current = current.next; } if (current.next!= null) { current.next = current.next.next; size--; if (current.next == null) { tail = current; } } } public T removeHead() { T removedHead = head.data; head = head.next; size--; if (head == null) { tail = null; } return removedHead; } public T removeTail() { T removedTail = tail.data; if (head == tail) { head = null; tail = null; } else { Node current = head; while (current.next!= tail) { current = current.next; } tail = current; tail.next = null; } size--; return removedTail; } @Override public String toString() { StringBuilder builder = new StringBuilder(); Node current = head; while (current!= null) { builder.append(current.data); if (current.next!= null) { builder.append(" -> "); } current = current.next; } return builder.toString(); } private class Node { public Node next; public T data; public Node(T data) { this.data = data; } } }
add public method size() to the class Stack so the output will be
pop is: 4
peek is: 8
size is: 2
false
public class Stack<T> {
private LinkedList<T> list;
public Stack() {
list = new LinkedList<>();
}
public boolean isEmpty() {
return list.isEmpty();
}
public void push(T data) {
list.prepend(data);
}
public T pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
return list.removeHead();
}
public T peek() {
if (isEmpty()) {
throw new EmptyStackException();
}
return list.getHead();
}
}
public class Main {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
stack.push(6);
stack.push(8);
stack.push(4);
System.out.println("pop is: " + stack.pop());
System.out.println("peek is: " + stack.peek());
System.out.println("size is: " + stack.size());
System.out.println(stack.isEmpty());
}
}
public class LinkedList <T> {
private Node head;
private Node tail;
private int size;
public LinkedList() {
head = null;
tail = null;
size = 0;
}
public boolean isEmpty() {
return size == 0;
}
public void append(T data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
tail.next = newNode;
}
tail = newNode;
size++;
}
public void prepend(T data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
tail = newNode;
} else {
newNode.next = head;
head = newNode;
}
size++;
}
public T getHead() {
return head.data;
}
public T getTail() {
return tail.data;
}
public int size() {
return size;
}
public void removeByValue(T data) {
Node current = head;
while (current.next!= null && current.next.data!= data) {
current = current.next;
}
if (current.next!= null) {
current.next = current.next.next;
size--;
if (current.next == null) {
tail = current;
}
}
}
public T removeHead() {
T removedHead = head.data;
head = head.next;
size--;
if (head == null) {
tail = null;
}
return removedHead;
}
public T removeTail() {
T removedTail = tail.data;
if (head == tail) {
head = null;
tail = null;
} else {
Node current = head;
while (current.next!= tail) {
current = current.next;
}
tail = current;
tail.next = null;
}
size--;
return removedTail;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
Node current = head;
while (current!= null) {
builder.append(current.data);
if (current.next!= null) {
builder.append(" -> ");
}
current = current.next;
}
return builder.toString();
}
private class Node {
public Node next;
public T data;
public Node(T data) {
this.data = data;
}
}
}

Step by step
Solved in 4 steps with 2 images

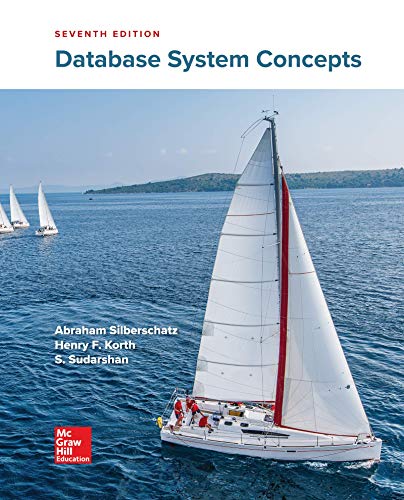
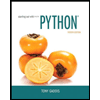
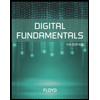
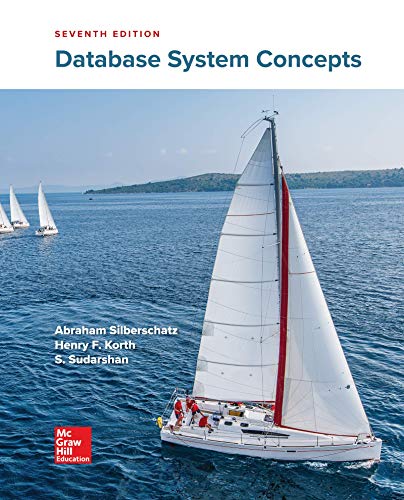
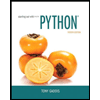
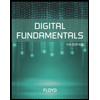
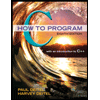
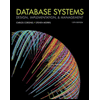
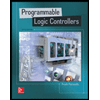