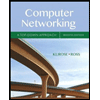
Draw a UML class diagram for the following code:
import java.util.LinkedList;
public class ListBasedQueue<E> {
private LinkedList<E> list = new LinkedList<E>();
public boolean isEmpty() {
return list.isEmpty();
}
public void enqueue(E item) {
list.addLast(item);
}
public E dequeue() {
if(isEmpty()) {
throw new IllegalStateException("Queue is empty. Cannot dequeue.");
}
return list.removeFirst();
}
public void dequeueAll() {
list.clear();
}
public E peek() {
if(isEmpty()) {
throw new IllegalStateException("Queue is empty. Cannot peek.");
}
return list.getFirst();
}
public static void main(String[] args) {
ListBasedQueue<Integer> queue = new ListBasedQueue<>();
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
System.out.println("Front of the queue: " + queue.peek());
System.out.println("Dequeue operation result: " + queue.dequeue());
System.out.println("Front of the queue post-dequeue: " + queue.peek());
queue.dequeueAll();
System.out.println("Is the queue empty after dequeueAll()? " + queue.isEmpty());
}
}

Step by stepSolved in 3 steps with 1 images

- I need a test harness for the following code: LinkedListImpl.java package linkedList; public abstract class LinkedListImpl implements LinkedList { private ListItem firstItem; public LinkedListImpl() { this.firstItem = null; } @Override public Boolean isItemInList(String thisItem) { ListItem currentItem = firstItem; while (currentItem != null) { if (currentItem.data.equals(thisItem)) { return true; } currentItem = currentItem.next; } return false; } @Override public Boolean addItem(String thisItem) { if (isItemInList(thisItem)) { return false; } else { ListItem newItem = new ListItem(thisItem); if (firstItem == null) { firstItem = newItem; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { currentItem = currentItem.next; } currentItem.next = newItem; } return true; } } @Override public Integer itemCount() { Integer count = 0; ListItem currentItem = firstItem; while (currentItem != null) { count++; currentItem = currentItem.next; } return count; }…arrow_forwardCould you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This…arrow_forwardadd public method size() to the class Stack so the output will be pop is: 4 peek is: 8 size is: 2 false public class Stack<T> { private LinkedList<T> list; public Stack() { list = new LinkedList<>(); } public boolean isEmpty() { return list.isEmpty(); } public void push(T data) { list.prepend(data); } public T pop() { if (isEmpty()) { throw new EmptyStackException(); } return list.removeHead(); } public T peek() { if (isEmpty()) { throw new EmptyStackException(); } return list.getHead(); } } public class Main { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(6); stack.push(8); stack.push(4); System.out.println("pop is: " + stack.pop()); System.out.println("peek is: " + stack.peek()); System.out.println("size is: " +…arrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardModify the Java class for the abstract stack type shown belowto use a linked list representation and test it with the same code thatappears in this chapter. class StackClass {private int [] stackRef;private int maxLen,topIndex;public StackClass() { // A constructorstackRef = new int [100];maxLen = 99;topIndex = -1;}public void push(int number) {if (topIndex == maxLen)System.out.println("Error in push–stack is full");else stackRef[++topIndex] = number;}public void pop() {if (empty())System.out.println("Error in pop–stack is empty"); else --topIndex;}public int top() {if (empty()) {System.out.println("Error in top–stack is empty");return 9999;}elsereturn (stackRef[topIndex]);}public boolean empty() {return (topIndex == -1);}}An example class that uses StackClass follows:public class TstStack {public static void main(String[] args) {StackClass myStack = new StackClass();myStack.push(42);myStack.push(29);System.out.println("29 is: " + myStack.top());myStack.pop();System.out.println("42 is:…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forward
- Given the tollowiıng class template detinition: template class linkedListType { public: const linked ListType& operator=(const linked ListType&); I/Overload the assignment operator. void initializeList(); /Initialize the list to an empty state. //Postcondition: first = nullptr, last = nullptr, count = 0; linked ListType(); Ildefault constructor //Initializes the list to an empty state. /Postcondition: first = nullptr, last = nullptr, count = 0; -linkedListīype(); Ildestructor /Deletes all the nodes from the list. //Postcondition: The list object is destroyed. protected: int count; //variable to store the number of elements in the list nodeType *first; //pointer to the first node of the list nodeType *last; //pointer to the last node of the list 1) Write initializeList() 2) Write linkedListType() 3) Write operator=0 4) Add the following function along with its definition: void rotate(); I/ Remove the first node of a linked list and put it at the end of the linked listarrow_forwardImplement the Linked List using head and tail pointer. Interface (.h file) of LinkedList class is given below. Your task is to provide implementation (.cpp file) for LinkedList class. Interface: template<class Type> class LinkedList { private: Node<Type>* head; Node<Type>* tail; <Type> data; public: LinkedList<Type>(); LinkedList<Type>(const LinkedList<Type> &obj); void sortedInsert(Type); Type deleteFromPosition(int position); //deletes the node at the particular position Type delete(int start,int end); //deletes the nodes from starting to ending position bool isEmpty()const; void print()const; ~LinkedList(); }; program in c++.arrow_forwardDraw a class diagram that shows how to use JCF class ArrayList, LinkedList, and Iterator using a UML drawing tool.arrow_forward
- Consider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forwardDraw a UML class diagram for the following code: import java.util.*; public class QueueOperationsDemo { public static void main(String[] args) { Queue<String> linkedListQueue = new LinkedList<>(); linkedListQueue.add("Apple"); linkedListQueue.add("Banana"); linkedListQueue.add("Cherry"); System.out.println("Is linkedListQueue empty? " + linkedListQueue.isEmpty()); System.out.println("Front element: " + linkedListQueue.peek()); System.out.println("Removed element: " + linkedListQueue.remove()); System.out.println("Front element after removal: " + linkedListQueue.peek()); Queue<Integer> arrayDequeQueue = new ArrayDeque<>(); arrayDequeQueue.add(10); arrayDequeQueue.add(20); arrayDequeQueue.add(30); System.out.println("Is arrayDequeQueue empty? " + arrayDequeQueue.isEmpty()); System.out.println("Front element: " + arrayDequeQueue.peek());…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
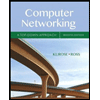
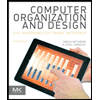
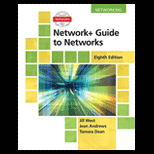
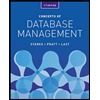
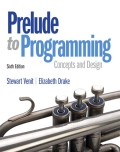
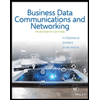