Create a class Queue and a Main class. This queue will be implemented using the LinkedList class that has been provided. This queue will hold values of a generic type (). Your Queue should have the following public methods: public void enqueue(T data) public T dequeue() public T peek() public int size() public boolean isEmpty() public class LinkedList { private Node head; private Node tail; private int size; @Override public boolean isEmpty() { return size == 0; } @Override public void append(T data) { Node node = new Node<>(data); if (tail == null) { head = tail = node; } else { tail.next = node; node.prev = tail; tail = node; } size++; } @Override public void prepend(T data) { Node node = new Node<>(data); if (head == null) { head = tail = node; } else { head.prev = node; node.next = head; head = node; } size++; } @Override public T getHead() { return head.data; } @Override public T getTail() { return tail.data; } @Override public int size() { return size; } @Override public T removeHead() { if (head == null) { return null; } T data = head.data; if (head == tail) { head = tail = null; } else { head = head.next; head.prev = null; } size--; return data; } @Override public T removeTail() { if (tail == null) { return null; } T data = tail.data; if (head == tail) { head = tail = null; } else { tail = tail.prev; tail.next = null; } size--; return data; } @Override public void removeByValue(T data) { Node current = head; while (current != null) { if (current.data.equals(data)) { if (current == head) { removeHead(); } else if (current == tail) { removeTail(); } else { current.prev.next = current.next; current.next.prev = current.prev; size--; } return; } current = current.next; } } @Override public String toString() { StringBuilder sb = new StringBuilder(); Node current = head; while (current != null) { sb.append(current.data); if (current != tail) { sb.append(" -> "); } current = current.next; } return sb.toString(); } private static class Node { T data; Node prev; Node next; public Node(T data) { this.data = data; } } }
Create a class Queue and a Main class. This queue will be implemented using the LinkedList class that has been provided. This queue will hold values of a generic type (<T>).
Your Queue should have the following public methods:
- public void enqueue(T data)
- public T dequeue()
- public T peek()
- public int size()
- public boolean isEmpty()
public class LinkedList<T> {
private Node<T> head;
private Node<T> tail;
private int size;
@Override
public boolean isEmpty() {
return size == 0;
}
@Override
public void append(T data) {
Node<T> node = new Node<>(data);
if (tail == null) {
head = tail = node;
} else {
tail.next = node;
node.prev = tail;
tail = node;
}
size++;
}
@Override
public void prepend(T data) {
Node<T> node = new Node<>(data);
if (head == null) {
head = tail = node;
} else {
head.prev = node;
node.next = head;
head = node;
}
size++;
}
@Override
public T getHead() {
return head.data;
}
@Override
public T getTail() {
return tail.data;
}
@Override
public int size() {
return size;
}
@Override
public T removeHead() {
if (head == null) {
return null;
}
T data = head.data;
if (head == tail) {
head = tail = null;
} else {
head = head.next;
head.prev = null;
}
size--;
return data;
}
@Override
public T removeTail() {
if (tail == null) {
return null;
}
T data = tail.data;
if (head == tail) {
head = tail = null;
} else {
tail = tail.prev;
tail.next = null;
}
size--;
return data;
}
@Override
public void removeByValue(T data) {
Node<T> current = head;
while (current != null) {
if (current.data.equals(data)) {
if (current == head) {
removeHead();
} else if (current == tail) {
removeTail();
} else {
current.prev.next = current.next;
current.next.prev = current.prev;
size--;
}
return;
}
current = current.next;
}
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
Node<T> current = head;
while (current != null) {
sb.append(current.data);
if (current != tail) {
sb.append(" -> ");
}
current = current.next;
}
return sb.toString();
}
private static class Node<T> {
T data;
Node<T> prev;
Node<T> next;
public Node(T data) {
this.data = data;
}
}
}

Step by step
Solved in 3 steps with 1 images

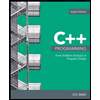
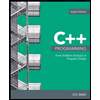