Algorithm: 1. Prompt the user for number of hours. 2. Read the value for hours. 3. Prompt the user for hourly rate. 4. Read the hourly rate. 5. Prompt the user for amount of bonus. 6. Read the value for bonus. 7. Compute the pay. 8. Compute the totalPay by adding bonus to pay. 9. Compute the tax deduction by multiplying 30% times totalPay. 10. Compute the netPay by subtracting tax from totalPay. 11. Neatly print and label the following: a. totalPay b. tax c. netPay Put your program on one of the PCs using a stand alone compiler such as Dev C++, or online compiler such as C++ shell. Name your program salary.cpp and save it on your cloud drive or flash drive after it works. You may write your complete program by hand first and document it as done in the sample class programs. Compile and run your program. It is recommended that you print a copy of your program and put it in your 3-ring binder
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
The Salary Program:
Modify the program from the textbook (Program 1-1,see below) which
- reads the number of hours an employee works
- reads the pay rate per hour
- computes the pay for the employee.
Add the following steps:
a) Read an amount of bonus paid to the employee.
b) Compute the total pay as pay plus bonus.
c) Compute the tax at 30% of the total pay.
d) Compute the net pay as total pay minus tax, and
e) Print a report showing: total pay, tax deduction, and net pay.
1. Prompt the user for number of hours.
2. Read the value for hours.
3. Prompt the user for hourly rate.
4. Read the hourly rate.
5. Prompt the user for amount of bonus.
6. Read the value for bonus.
7. Compute the pay.
8. Compute the totalPay by adding bonus to pay.
9. Compute the tax deduction by multiplying 30% times totalPay.
10. Compute the netPay by subtracting tax from totalPay.
11. Neatly print and label the following:
a. totalPay
b. tax
c. netPay
Put your program on one of the PCs using a stand alone compiler such as Dev C++, or online compiler such as C++ shell. Name your program salary.cpp and save it on your cloud drive or flash drive after it works. You may write your complete program by hand first and document it as done in the sample class programs. Compile and run your program. It is recommended that you print a copy of your program and put it in your 3-ring binder.
Program 1-1
// This program calculates the user's pay.
#include <iostream>
using namespace std;
int main()
{
double hours, rate, pay;
// Get the number of hours worked.
cout << "How many hours did you work? ";
cin >> hours;
// Get the hourly pay rate.
cout << "How much do you get paid per hour? ";
cin >> rate;
// Calculate the pay.
pay = hours * rate;
// Display the pay.
cout << "You have earned " << pay << endl;
return 0;
}

Step by step
Solved in 3 steps with 1 images

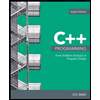
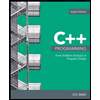