Analyze the ChatGPT output by answering the following questions. Data types Which data types would be accepted by the function count_crossings_and_nestings for the argument arcs? Would a set work? What does the function return? What data type is it? Run the example suggested by ChatGPT. Is the function count_crossings_and_nestings working as expected? Try computing nestings and crossings with the smallest examples {}, {(0,1)}, {(0,1),(2,3)}, {(0,3),(1,2)} and {(0,2),(1,3}. Does the output work?
Analyze the ChatGPT output by answering the following questions. Data types Which data types would be accepted by the function count_crossings_and_nestings for the argument arcs? Would a set work? What does the function return? What data type is it? Run the example suggested by ChatGPT. Is the function count_crossings_and_nestings working as expected? Try computing nestings and crossings with the smallest examples {}, {(0,1)}, {(0,1),(2,3)}, {(0,3),(1,2)} and {(0,2),(1,3}. Does the output work?
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 24PE
Related questions
Question
100%
Analyze the ChatGPT output by answering the following questions.
Data types
- Which data types would be accepted by the function count_crossings_and_nestings for the argument arcs? Would a set work?
- What does the function return? What data type is it?
- Run the example suggested by ChatGPT. Is the function count_crossings_and_nestings working as expected?
- Try computing nestings and crossings with the smallest examples {}, {(0,1)}, {(0,1),(2,3)}, {(0,3),(1,2)} and {(0,2),(1,3}. Does the output work?
![When we asked ChatGPT 3.5 to "write Python code to count the number of crossings and nestings of an arc diagram on 2n points labelled by 0 to 2n-1 on the line" (we
had to add the labelling instruction to get the indexing right), it produced the following answer.
Sure, an arc diagram represents pairwise relationships between points. Here's a Python code snippet using a simple approach to count the number of
crossings and nestings in an arc diagram on 2n points:
def count_crossings_and_nestings(arcs):
n = len(arcs) // 2
crossings
nestings
= 0
0
for i in range(2 * n):
for j in range(i + 1, 2 * n):
if arcs[i] [0] < arcs[j][0] < arcs[i] [1] < arcs[j][1]:
crossings += 1
elif arcs [i] [0] > arcs [j] [0] > arcs [i] [1] > arcs [j] [1]:
crossings += 1
elif arcs [i] [0] < arcs [j] [0] < arcs[j] [1] < arcs[i] [1]:
nestings += 1
elif arcs [i] [0] > arcs [j] [0] > arcs [i] [1] > arcs [j] [1] :
nestings += 1
return crossings, nestings
# Example usage:
n = 4 # You can change this to the desired number of points (2n points)
arcs = [(0, 3), (1, 2), (4, 7), (5, 6), (8, 11), (9, 10), (12, 15), (13, 14)]
crossings, nestings = count_crossings_and_nestings(arcs)
print (f"Number of crossings: {crossings}")
print (f"Number of nestings: {nestings}")
This code defines a function count_crossings_and_nestings that takes a list of arcs as input, where each arc is represented as a tuple (start, end).
The function then iterates through all pairs of arcs and checks for crossings and nestings based on their start and end points. The example usage shows
how to use the function with a sample set of arcs.
You can modify the arcs variable with your specific arc diagram data for testing.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3688a312-1b6e-40d4-973f-537807921925%2F8ca9000c-435e-4018-8735-9c082b8322a0%2Fx461xz_processed.png&w=3840&q=75)
Transcribed Image Text:When we asked ChatGPT 3.5 to "write Python code to count the number of crossings and nestings of an arc diagram on 2n points labelled by 0 to 2n-1 on the line" (we
had to add the labelling instruction to get the indexing right), it produced the following answer.
Sure, an arc diagram represents pairwise relationships between points. Here's a Python code snippet using a simple approach to count the number of
crossings and nestings in an arc diagram on 2n points:
def count_crossings_and_nestings(arcs):
n = len(arcs) // 2
crossings
nestings
= 0
0
for i in range(2 * n):
for j in range(i + 1, 2 * n):
if arcs[i] [0] < arcs[j][0] < arcs[i] [1] < arcs[j][1]:
crossings += 1
elif arcs [i] [0] > arcs [j] [0] > arcs [i] [1] > arcs [j] [1]:
crossings += 1
elif arcs [i] [0] < arcs [j] [0] < arcs[j] [1] < arcs[i] [1]:
nestings += 1
elif arcs [i] [0] > arcs [j] [0] > arcs [i] [1] > arcs [j] [1] :
nestings += 1
return crossings, nestings
# Example usage:
n = 4 # You can change this to the desired number of points (2n points)
arcs = [(0, 3), (1, 2), (4, 7), (5, 6), (8, 11), (9, 10), (12, 15), (13, 14)]
crossings, nestings = count_crossings_and_nestings(arcs)
print (f"Number of crossings: {crossings}")
print (f"Number of nestings: {nestings}")
This code defines a function count_crossings_and_nestings that takes a list of arcs as input, where each arc is represented as a tuple (start, end).
The function then iterates through all pairs of arcs and checks for crossings and nestings based on their start and end points. The example usage shows
how to use the function with a sample set of arcs.
You can modify the arcs variable with your specific arc diagram data for testing.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
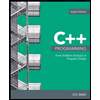
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
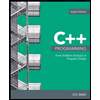
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning