Given a string str and number n, write a program that recursively appends a copy of string str n times and returns the resulting string. For example: RecuriveCopy("a", 10) // -> "aaaaaaaaaaa" RecursiveCopy("blah", 5) // -> "blahblahblahblahblahblah" For this exercise, complete the following function. n is the number of copies to add to str: function RecursiveCopy(str, n) { // your code here } Part 2: Call RecursiveCopy Write another function that calls RecursiveCopy() and returns the following string output: "The resulting string [x] has [y] characters". [x] should be replaced by the result from RecursiveCopy) and [y] should be replaced by the number of characters in the resulting string. You may pick the arguments to RecursiveCopy in CountChars or have the user provide input. I will leave this up to you. function CountChars() { } // your code here // call RecursiveCopy(..) // returns a formatted string For example: console.log(CountChars()) // -> "The resulting string blahblahblahblah has 16 characters"
Given a string str and number n, write a program that recursively appends a copy of string str n times and returns the resulting string. For example: RecuriveCopy("a", 10) // -> "aaaaaaaaaaa" RecursiveCopy("blah", 5) // -> "blahblahblahblahblahblah" For this exercise, complete the following function. n is the number of copies to add to str: function RecursiveCopy(str, n) { // your code here } Part 2: Call RecursiveCopy Write another function that calls RecursiveCopy() and returns the following string output: "The resulting string [x] has [y] characters". [x] should be replaced by the result from RecursiveCopy) and [y] should be replaced by the number of characters in the resulting string. You may pick the arguments to RecursiveCopy in CountChars or have the user provide input. I will leave this up to you. function CountChars() { } // your code here // call RecursiveCopy(..) // returns a formatted string For example: console.log(CountChars()) // -> "The resulting string blahblahblahblah has 16 characters"
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 6PE
Related questions
Question
![Given a string str and number n, write a program that recursively appends a copy of string str n times and returns the resulting string. For example:
RecuriveCopy("a", 10)
// -> "aaaaaaaaaaa"
RecursiveCopy("blah", 5)
// -> "blahblahblahblahblahblah"
For this exercise, complete the following function. n is the number of copies to add to str:
function RecursiveCopy(str, n) {
// your code here
}
Part 2: Call RecursiveCopy
Write another function that calls RecursiveCopy() and returns the following string output: "The resulting string [x] has [y] characters". [x] should be replaced by the
result from RecursiveCopy) and [y] should be replaced by the number of characters in the resulting string. You may pick the arguments to RecursiveCopy in
CountChars or have the user provide input. I will leave this up to you.
function CountChars() {
}
// your code here
// call RecursiveCopy(..)
// returns a formatted string
For example:
console.log(CountChars())
// -> "The resulting string blahblahblahblah has 16 characters"](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34ad7f09-3af4-48a5-aeaa-19cf65627345%2F0b4fa10e-cbcc-4100-aed5-dc17cdc50e1a%2Ff4ggap2_processed.png&w=3840&q=75)
Transcribed Image Text:Given a string str and number n, write a program that recursively appends a copy of string str n times and returns the resulting string. For example:
RecuriveCopy("a", 10)
// -> "aaaaaaaaaaa"
RecursiveCopy("blah", 5)
// -> "blahblahblahblahblahblah"
For this exercise, complete the following function. n is the number of copies to add to str:
function RecursiveCopy(str, n) {
// your code here
}
Part 2: Call RecursiveCopy
Write another function that calls RecursiveCopy() and returns the following string output: "The resulting string [x] has [y] characters". [x] should be replaced by the
result from RecursiveCopy) and [y] should be replaced by the number of characters in the resulting string. You may pick the arguments to RecursiveCopy in
CountChars or have the user provide input. I will leave this up to you.
function CountChars() {
}
// your code here
// call RecursiveCopy(..)
// returns a formatted string
For example:
console.log(CountChars())
// -> "The resulting string blahblahblahblah has 16 characters"
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
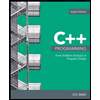
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
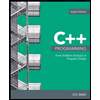
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning