Hello, I am having some trouble with this C++homework question. 1. Implement the following: a. A template class named MyArray. 1) MyArray is a dynamic partially filled array for primitive types. 2) data members: - a pointer for the array - any associated variables needed to manage the array.
Hello, I am having some trouble with this C++homework question. 1. Implement the following: a. A template class named MyArray. 1) MyArray is a dynamic partially filled array for primitive types. 2) data members: - a pointer for the array - any associated variables needed to manage the array.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Test using following main function (DO NOT CHANGE the provided int main as any changes will not be accepted)
![Hello, I am having some trouble with this
C++homework question.
1. Implement the following:
a. A template class named MyArray.
1) MyArray is a dynamic partially filled array for
primitive types.
2) data members:
- a pointer for the array
- any associated variables needed to manage
the array.
3) Constructor must insure that specified capacity
is possible. Exit the program if
an illegal value is specified.
4) “The Big Three" are required to insure deep
copy.
5) Private grow function is used to automatically
increase the size of the array
when adding elements.
6) Add function to safely append elements to the
array.
7) getSize function that returns the current number
of elements.
8) Overloaded the [] operator to read and update
existing elements.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0262c52b-6ed5-4d62-a9e6-892d2b0b045b%2F3bf71234-0fcc-496c-8743-d4a45cc51404%2F66xiyqc_processed.png&w=3840&q=75)
Transcribed Image Text:Hello, I am having some trouble with this
C++homework question.
1. Implement the following:
a. A template class named MyArray.
1) MyArray is a dynamic partially filled array for
primitive types.
2) data members:
- a pointer for the array
- any associated variables needed to manage
the array.
3) Constructor must insure that specified capacity
is possible. Exit the program if
an illegal value is specified.
4) “The Big Three" are required to insure deep
copy.
5) Private grow function is used to automatically
increase the size of the array
when adding elements.
6) Add function to safely append elements to the
array.
7) getSize function that returns the current number
of elements.
8) Overloaded the [] operator to read and update
existing elements.
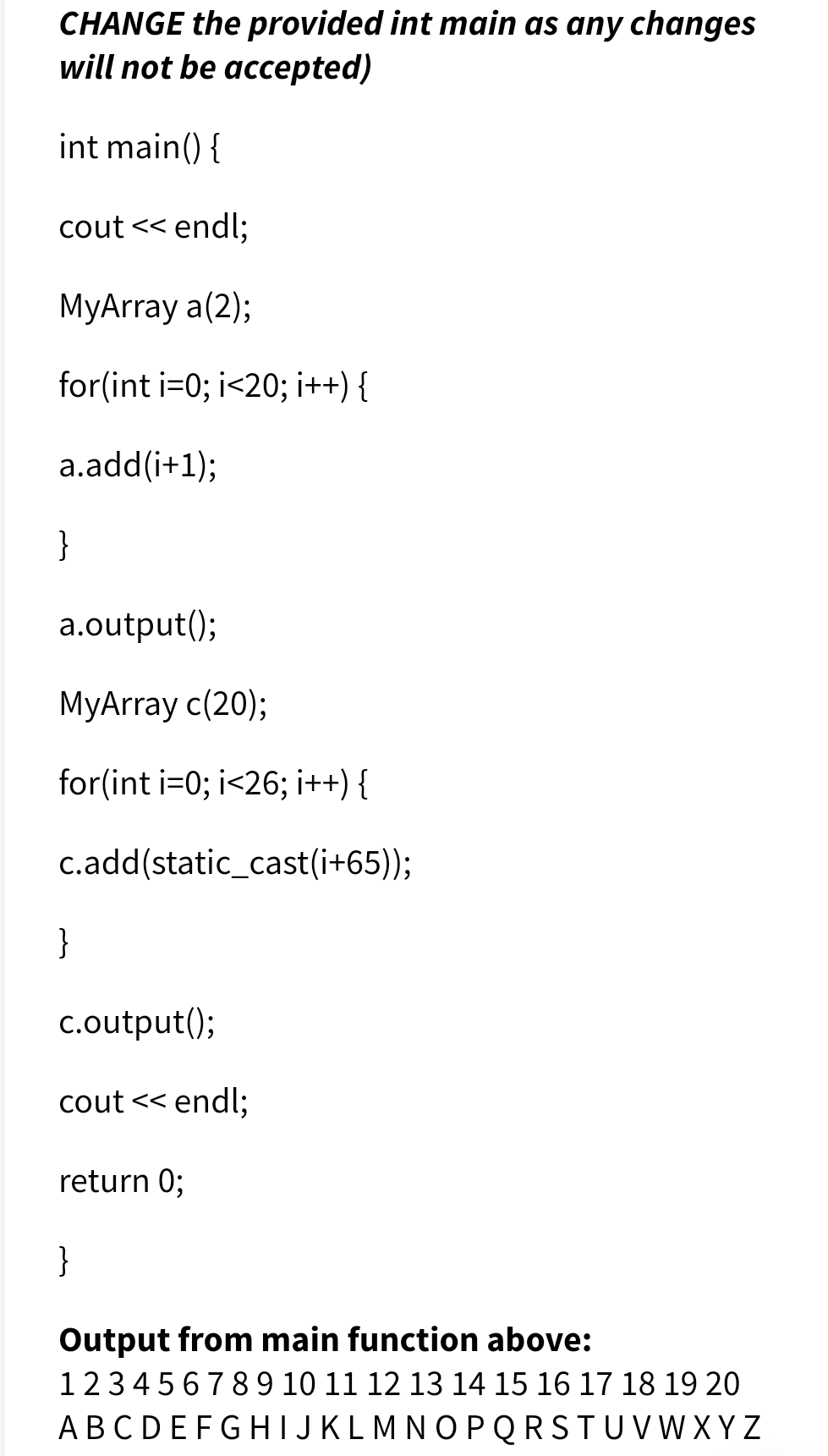
Transcribed Image Text:CHANGE the provided int main as any changes
will not be accepted)
int main() {
cout << endl;
MyArray a(2);
for(int i=0; i<20; i++) {
a.add(i+1);
}
a.output();
MyArray c(20);
for(int i=0; i<26; i++) {
c.add(static_cast(i+65));
}
c.output();
cout << endl;
return 0;
}
Output from main function above:
123456789 10 11 12 13 14 15 16 17 18 19 20
ABCDEFGHIJKLMNOPQRSTUVW XY Z
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
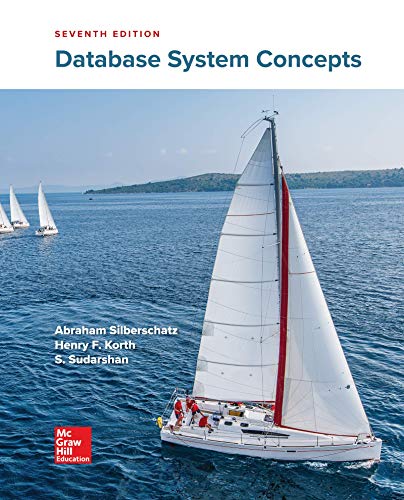
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
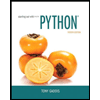
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
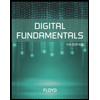
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
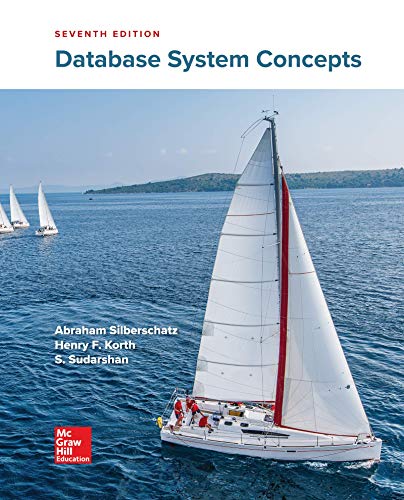
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
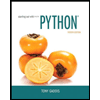
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
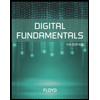
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
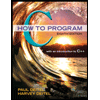
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
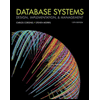
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
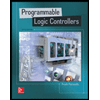
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education