-Modify this program to ask the enter the employees' information in order of last name, first name, number of hours, hourly rate, and if they are a cyber sec or computer sci major or both -To ask the user to enter the info, ask "Enter the info of ten employees as shown: Last Name, First Name, # of hours worked, hourly rate and if they are a cyber sec or computer sci major or both" -Have the calculations and amount the same as shown in the program and have it printed to the terminal and in the employee.txt file -The calculations should be the hours x # of hours worked, plus 1000 if they are a cyber sec or computer sci major and 2000 if they are both. -An example of the output is in the images #include using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee*); void appendNode(Employee*&, Employee*); int main() { const int NumOfEmp = 10; Employee* head = nullptr; Employee* newNode; string firstNames[] = {"John", "Jane", "Mary", "Mike", "Sarah", "David", "Emily", "Alex", "Ethan", "Olivia"}; string lastNames[] = {"Smith", "Johnson", "Williams", "Jones", "Brown", "Garcia", "Miller", "Davis", "Rodriguez", "Martinez"}; int numOfHours[] = {40, 35, 42, 37, 39, 41, 38, 36, 43, 34}; float hourlyRate[] = {20.0, 22.5, 21.0, 22.0, 20.5, 23.0, 22.0, 21.5, 23.5, 20.0}; char comp_sci[] = {'Y', 'N', 'Y', 'Y', 'N', 'Y', 'N', 'Y', 'N', 'Y'}; char comp_sec[] = {'N', 'N', 'Y', 'Y', 'Y', 'N', 'Y', 'Y', 'N', 'N'}; cout<firstName = firstNames[i]; newNode->lastName = lastNames[i]; newNode->numOfHours = numOfHours[i]; newNode->hourlyRate = hourlyRate[i]; newNode->major[0] = comp_sci[i]; newNode->major[1] = comp_sec[i]; newNode->amount = newNode->numOfHours * newNode->hourlyRate; if (newNode->major[0] == 'Y' || newNode->major[1] == 'Y') { newNode->amount = newNode->amount + 1000; } if (newNode->major[0] == 'Y' && newNode->major[1] == 'Y') { newNode->amount = newNode->amount + 2000; } newNode->next = nullptr; appendNode(head, newNode); printRecord(newNode); } ofstream outfile("Employee.txt"); if (!outfile) { cerr << "Error: Output file could not be opened." << endl; return 1; } outfile<lastName << setw(15) << current->firstName << setw(12) << current->numOfHours << setw(20) << current->hourlyRate << setw(20) << current->amount << setw(20) << current->major[0]<major[1] << endl; current = current->next; } outfile.close(); return 0; } void printRecord(Employee* e) { cout << left << setw(15) << e->lastName << setw(15) << e->firstName << setw(12) <numOfHours<hourlyRate<amount << setw(10) << e->major[0] << setw(10) << e->major[1]<< endl; } void appendNode(Employee*& head, Employee* newNode) { if (head == nullptr) { head = newNode; } else { Employee* current = head; while (current->next != nullptr) { current = current->next; } current->next = newNode; } }
-Modify this program to ask the enter the employees' information in order of last name, first name, number of hours, hourly rate, and if they are a cyber sec or computer sci major or both -To ask the user to enter the info, ask "Enter the info of ten employees as shown: Last Name, First Name, # of hours worked, hourly rate and if they are a cyber sec or computer sci major or both" -Have the calculations and amount the same as shown in the program and have it printed to the terminal and in the employee.txt file -The calculations should be the hours x # of hours worked, plus 1000 if they are a cyber sec or computer sci major and 2000 if they are both. -An example of the output is in the images #include using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee*); void appendNode(Employee*&, Employee*); int main() { const int NumOfEmp = 10; Employee* head = nullptr; Employee* newNode; string firstNames[] = {"John", "Jane", "Mary", "Mike", "Sarah", "David", "Emily", "Alex", "Ethan", "Olivia"}; string lastNames[] = {"Smith", "Johnson", "Williams", "Jones", "Brown", "Garcia", "Miller", "Davis", "Rodriguez", "Martinez"}; int numOfHours[] = {40, 35, 42, 37, 39, 41, 38, 36, 43, 34}; float hourlyRate[] = {20.0, 22.5, 21.0, 22.0, 20.5, 23.0, 22.0, 21.5, 23.5, 20.0}; char comp_sci[] = {'Y', 'N', 'Y', 'Y', 'N', 'Y', 'N', 'Y', 'N', 'Y'}; char comp_sec[] = {'N', 'N', 'Y', 'Y', 'Y', 'N', 'Y', 'Y', 'N', 'N'}; cout<firstName = firstNames[i]; newNode->lastName = lastNames[i]; newNode->numOfHours = numOfHours[i]; newNode->hourlyRate = hourlyRate[i]; newNode->major[0] = comp_sci[i]; newNode->major[1] = comp_sec[i]; newNode->amount = newNode->numOfHours * newNode->hourlyRate; if (newNode->major[0] == 'Y' || newNode->major[1] == 'Y') { newNode->amount = newNode->amount + 1000; } if (newNode->major[0] == 'Y' && newNode->major[1] == 'Y') { newNode->amount = newNode->amount + 2000; } newNode->next = nullptr; appendNode(head, newNode); printRecord(newNode); } ofstream outfile("Employee.txt"); if (!outfile) { cerr << "Error: Output file could not be opened." << endl; return 1; } outfile<lastName << setw(15) << current->firstName << setw(12) << current->numOfHours << setw(20) << current->hourlyRate << setw(20) << current->amount << setw(20) << current->major[0]<major[1] << endl; current = current->next; } outfile.close(); return 0; } void printRecord(Employee* e) { cout << left << setw(15) << e->lastName << setw(15) << e->firstName << setw(12) <numOfHours<hourlyRate<amount << setw(10) << e->major[0] << setw(10) << e->major[1]<< endl; } void appendNode(Employee*& head, Employee* newNode) { if (head == nullptr) { head = newNode; } else { Employee* current = head; while (current->next != nullptr) { current = current->next; } current->next = newNode; } }
Fundamentals of Information Systems
9th Edition
ISBN:9781337097536
Author:Ralph Stair, George Reynolds
Publisher:Ralph Stair, George Reynolds
Chapter7: Knowledge Management And Specialized Information Systems
Section: Chapter Questions
Problem 7DQ
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
-Modify this program to ask the enter the employees' information in order of last name, first name, number of hours, hourly rate, and if they are a cyber sec or computer sci major or both
-To ask the user to enter the info, ask
"Enter the info of ten employees as shown: Last Name, First Name, # of hours worked, hourly rate and if they are a cyber sec or computer sci major or both"
-Have the calculations and amount the same as shown in the program and have it printed to the terminal and in the employee.txt file
-The calculations should be the hours x # of hours worked, plus 1000 if they are a cyber sec or computer sci major and 2000 if they are both.
-An example of the output is in the images
#include<bits/stdc++.h>
using namespace std;
struct Employee {
string firstName;
string lastName;
int numOfHours;
float hourlyRate;
char major[2];
float amount;
Employee* next;
};
void printRecord(Employee*);
void appendNode(Employee*&, Employee*);
int main() {
const int NumOfEmp = 10;
Employee* head = nullptr;
Employee* newNode;
string firstNames[] = {"John", "Jane", "Mary", "Mike", "Sarah", "David", "Emily", "Alex", "Ethan", "Olivia"};
string lastNames[] = {"Smith", "Johnson", "Williams", "Jones", "Brown", "Garcia", "Miller", "Davis", "Rodriguez", "Martinez"};
int numOfHours[] = {40, 35, 42, 37, 39, 41, 38, 36, 43, 34};
float hourlyRate[] = {20.0, 22.5, 21.0, 22.0, 20.5, 23.0, 22.0, 21.5, 23.5, 20.0};
char comp_sci[] = {'Y', 'N', 'Y', 'Y', 'N', 'Y', 'N', 'Y', 'N', 'Y'};
char comp_sec[] = {'N', 'N', 'Y', 'Y', 'Y', 'N', 'Y', 'Y', 'N', 'N'};
cout<<left<<setw(15) <<"Last Name"<<setw(15) <<"First Name"<<setw(12) <<"# of Hours"<<setw(12) <<"Hourly Rate"
<< setw(10) << "Amount"<< setw(10)<<"Comp Sci" << setw(20) << "Comp Sec" << endl;
for (int i = 0; i < NumOfEmp; i++) {
newNode = new Employee;
newNode->firstName = firstNames[i];
newNode->lastName = lastNames[i];
newNode->numOfHours = numOfHours[i];
newNode->hourlyRate = hourlyRate[i];
newNode->major[0] = comp_sci[i];
newNode->major[1] = comp_sec[i];
newNode->amount = newNode->numOfHours * newNode->hourlyRate;
if (newNode->major[0] == 'Y' || newNode->major[1] == 'Y') {
newNode->amount = newNode->amount + 1000;
}
if (newNode->major[0] == 'Y' && newNode->major[1] == 'Y') {
newNode->amount = newNode->amount + 2000;
}
newNode->next = nullptr;
appendNode(head, newNode);
printRecord(newNode);
}
ofstream outfile("Employee.txt");
if (!outfile) {
cerr << "Error: Output file could not be opened." << endl;
return 1;
}
outfile<<left<<setw(15) <<"Last Name"<<setw(15) <<"First Name"<<setw(12) <<"# of Hours"<<setw(20) <<"Hourly Rate"
<< setw(20) << "Amount"<< setw(20)<<"Comp Sci" << setw(20) << "Comp Sec" << endl;
Employee* current = head;
while (current != nullptr) {
outfile << left << setw(15) << current->lastName << setw(15) << current->firstName << setw(12) << current->numOfHours << setw(20)
<< current->hourlyRate << setw(20) << current->amount << setw(20) << current->major[0]<<setw(25)<< setw(25) << current->major[1] << endl;
current = current->next;
}
outfile.close();
return 0;
}
void printRecord(Employee* e) {
cout << left << setw(15) << e->lastName << setw(15) << e->firstName << setw(12)
<<e->numOfHours<<setw(12) <<e->hourlyRate<<setw(10) <<e->amount
<< setw(10) << e->major[0] << setw(10) << e->major[1]<< endl;
}
void appendNode(Employee*& head, Employee* newNode) {
if (head == nullptr) {
head = newNode;
}
else {
Employee* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
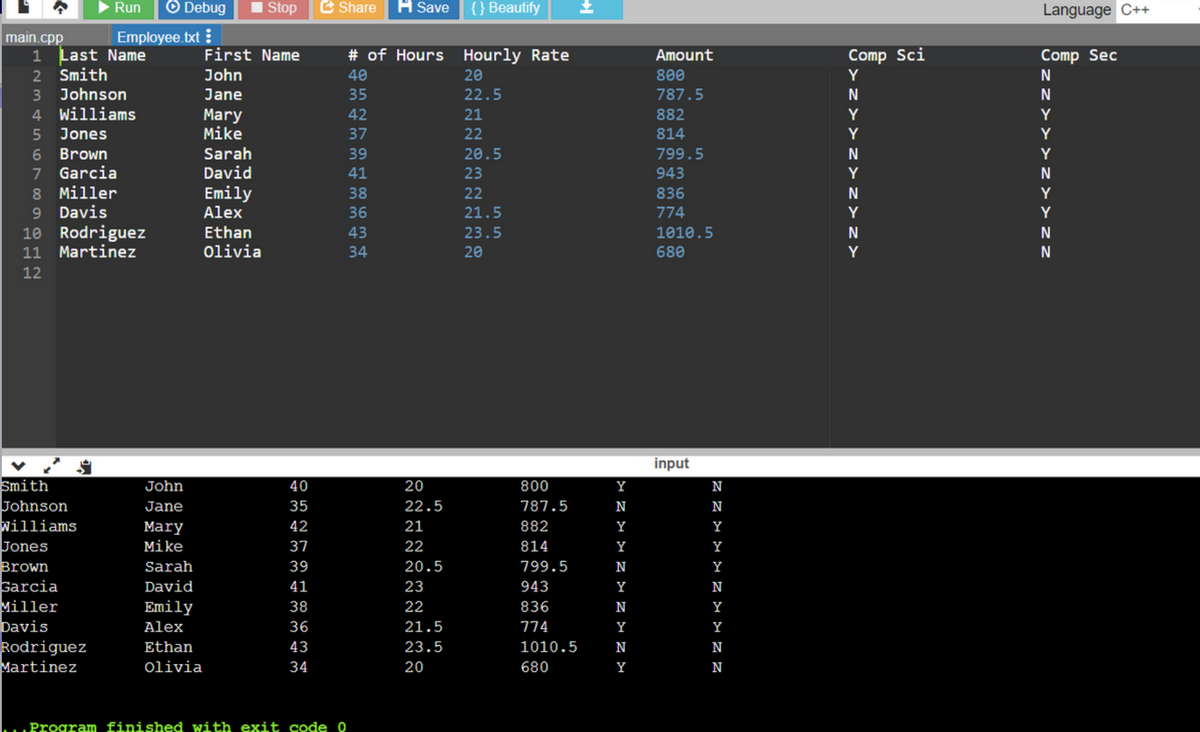
Transcribed Image Text:main.cpp
1 Last Name
2 Smith
3 Johnson
4 Williams
5 Jones
6 Brown
7 Garcia
8 Miller
9 Davis
10 Rodriguez
11 Martinez
12
Smith
Johnson
Williams
Run ✪ Debug
Employee.txt:
Jones
Brown
Garcia
Miller
Davis
Rodriguez
Martinez
John
Jane
Mary
Mike
Sarah
David
Emily
Alex
Mary
Mike
■ Stop
First Name
John
Jane
Ethan
olivia
Sarah
David
Emily
Alex
Ethan
Olivia
40
35
42
37
39
41
38
36
43
34
Share
. Program finished with exit code 0
39
41
H Save
# of Hours Hourly Rate
40
35
42
37
38
36
43
34
20
22.5
21
22
20.5
23
22
{} Beautify
21.5
23.5
20
20
22.5
21
22
20.5
23
22
21.5
23.5
20
800
787.5
882
814
799.5
943
836
774
1010.5
680
Y
MHMARHAMAD
N
Y
Y
N
Y
N
Y
N
Y
Amount
800
787.5
882
814
799.5
943
836
774
1010.5
680
input
NNNNNNNNNN
Y
Y
Y
Y
Y
Comp Sci
Y
> > > > > > > > > >
N
Y
Y
N
Y
N
Y
N
Y
Language C++
Comp Sec
N
N
Y
Y
Y
Y
Y
N
N
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
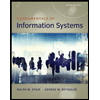
Fundamentals of Information Systems
Computer Science
ISBN:
9781337097536
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
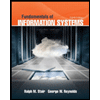
Fundamentals of Information Systems
Computer Science
ISBN:
9781305082168
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
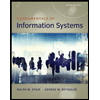
Fundamentals of Information Systems
Computer Science
ISBN:
9781337097536
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
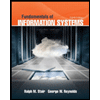
Fundamentals of Information Systems
Computer Science
ISBN:
9781305082168
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning