ANSWER ASAP. C++ LANGUAGE ONLY. (NOT C, NOT JAVA, NOT C#) CPP ONLY Using the double-ended linked list C language syntax code below, convert it into C++ and use it as the base. Modify the provided program into the ff: create a new class named student_queue. and then.. Implement the following methods for the class: front() - returns the pointer to the front element of the queue back() - returns the pointer to the back element of the queue push() - allocates memory for a new node and connects it to the existing queue pop() - retrieves the oldest node in the queue, copies the data to a structure, and frees up the memory from the queue empty() - returns 1 or 0 depending on whether the queue is empty or not size() - returns the number of elements in the queue Program code: #include #include typedef struct se_list_type { int num; struct se_list_type* prev; struct se_list_type* next; } se_list; int main() { // Start the list se_list* head = NULL; se_list* cur = NULL; // Create the first node head = (se_list*)malloc(sizeof(se_list)); head->num = 2; head->prev = NULL; head->next = NULL; cur = head; // Create the second node cur->next = (se_list*)malloc(sizeof(se_list)); cur->next->prev = cur; cur = cur->next; cur->num = 1; cur->prev = head; cur->next = NULL; // Create the third node cur->next = (se_list*)malloc(sizeof(se_list)); cur->next->prev = cur; cur = cur->next; cur->num = -5; cur->prev = head->next; cur->next = NULL; // Create 10 more random nodes srand(0); for (int i = 0; i < 10; i++) { cur->next = (se_list*)malloc(sizeof(se_list)); cur->next->prev = cur; cur = cur->next; cur->num = rand() % 20 - 10; cur->prev = cur->next; cur->next = NULL; } // Print the list forward cur = head; while (cur != NULL) { printf("%d ", cur->num); cur = cur->next; } printf("\n"); // Free the memory cur = head; while (cur != NULL) { se_list* next = cur->next; free(cur); cur = next; } return 0; } THANK YOU!
ANSWER ASAP.
C++ LANGUAGE ONLY. (NOT C, NOT JAVA, NOT C#) CPP ONLY
Using the double-ended linked list C language syntax code below, convert it into C++ and use it as the base.
Modify the provided program into the ff:
- create a new class named student_queue.
and then..
- Implement the following methods for the class:
- front() - returns the pointer to the front element of the queue
- back() - returns the pointer to the back element of the queue
- push() - allocates memory for a new node and connects it to the existing queue
- pop() - retrieves the oldest node in the queue, copies the data to a structure, and frees up the memory from the queue
- empty() - returns 1 or 0 depending on whether the queue is empty or not
- size() - returns the number of elements in the queue
Program code:
#include <stdio.h>
#include <stdlib.h>
typedef struct se_list_type {
int num;
struct se_list_type* prev;
struct se_list_type* next;
} se_list;
int main() {
// Start the list
se_list* head = NULL;
se_list* cur = NULL;
// Create the first node
head = (se_list*)malloc(sizeof(se_list));
head->num = 2;
head->prev = NULL;
head->next = NULL;
cur = head;
// Create the second node
cur->next = (se_list*)malloc(sizeof(se_list));
cur->next->prev = cur;
cur = cur->next;
cur->num = 1;
cur->prev = head;
cur->next = NULL;
// Create the third node
cur->next = (se_list*)malloc(sizeof(se_list));
cur->next->prev = cur;
cur = cur->next;
cur->num = -5;
cur->prev = head->next;
cur->next = NULL;
// Create 10 more random nodes
srand(0);
for (int i = 0; i < 10; i++) {
cur->next = (se_list*)malloc(sizeof(se_list));
cur->next->prev = cur;
cur = cur->next;
cur->num = rand() % 20 - 10;
cur->prev = cur->next;
cur->next = NULL;
}
// Print the list forward
cur = head;
while (cur != NULL) {
printf("%d ", cur->num);
cur = cur->next;
}
printf("\n");
// Free the memory
cur = head;
while (cur != NULL) {
se_list* next = cur->next;
free(cur);
cur = next;
}
return 0;
}
THANK YOU!

Step by step
Solved in 4 steps with 2 images

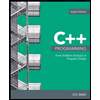
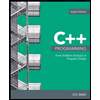