answer the following question by writing a python code: 5- Create the “Testing” file that does the following: a) Use the constructor to create an object of Hourly_Based_Employee class. b) Use the constructor to create an object of Salary_Based_Employee class. c) Use the constructor to create object of Manager_Employee class. d) Call computeSalary and print methods using objects created in a, b, and c
answer the following question by writing a python code: 5- Create the “Testing” file that does the following: a) Use the constructor to create an object of Hourly_Based_Employee class. b) Use the constructor to create an object of Salary_Based_Employee class. c) Use the constructor to create object of Manager_Employee class. d) Call computeSalary and print methods using objects created in a, b, and c
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
answer the following question by writing a python code:
5- Create the “Testing” file that does the following:
a) Use the constructor to create an object of Hourly_Based_Employee class.
b) Use the constructor to create an object of Salary_Based_Employee class.
c) Use the constructor to create object of Manager_Employee class.
d) Call computeSalary and print methods using objects created in a, b, and c
use this code to do so
class Employee:
# constructor
def__init__(self, name, date_of_birth, starting_date, ssn, phone_number):
self.name = name
self.date_of_birth = date_of_birth
self.starting_date = starting_date
self.ssn = ssn
self.phone_number = phone_number
# getters
defget_name(self):
returnself.name
defget_date_of_birth(self):
returnself.date_of_birth
defget_starting_date(self):
returnself.starting_date
defget_ssn(self):
returnself.ssn
defget_phone_number(self):
returnself.phone_number
# setters
defset_name(self, name):
self.name = name
defset_date_of_birth(self, date_of_birth):
self.date_of_birth = date_of_birth
defset_starting_date(self, starting_date):
self.starting_date = starting_date
defset_ssn(self, ssn):
self.ssn = ssn
defset_phone_number(self, phone_number):
self.phone_number = phone_number
# print method
defprint(self):
print("Name: " + self.name)
print("Date of birth: " + self.date_of_birth)
print("Starting date: " + self.starting_date)
print("SSN: " + self.ssn)
print("Phone number: " + self.phone_number)
class Hourly_Based_Employee(Employee):
# constructor
def__init__(self, name, date_of_birth, starting_date, ssn, phone_number, per_hour, hours):
super().__init__(name, date_of_birth, starting_date, ssn, phone_number)
self.per_hour = per_hour
self.hours = hours
# getters
defget_per_hour(self):
returnself.per_hour
defget_hours(self):
returnself.hours
# setters
defset_per_hour(self, per_hour):
self.per_hour = per_hour
defset_hours(self, hours):
self.hours = hours
# compute_salary method
defcompute_salary(self):
returnself.per_hour * self.hours
# print method
defprint(self):
super().print()
print("Per hour: " + str(self.per_hour))
print("Hours: " + str(self.hours))
emp.print()
print("Salary: ", emp.compute_salary())
# call the main function
if __name__ == "__main__":
main()
class Salary_Based_Employee(Employee):
#Rest data members are dervied from the super class.
phone_number = 0
salary=0
#Constructor
def__init__(self, name, date_of_birth, starting_date, ssn, phone_number,salary):
super().__init__(name, date_of_birth, starting_date, ssn)
self.phone_number = phone_number
self.salary = salary
#Getter methods
defgetPhoneNumber(self):
returnself.phone_number
defgetSalary(self):
returnself.salary
#Setter methods
defsetPhoneNumber(self, phone_number):
self.phone_number = phone_number
defsetSalary(self, salary):
self.salary = salary
defcomputeSalary(self):
return salary
defprint(self):
super.print()
print("Phone Number: ", self.phone_number)
print("Salary: ", self.salary)
class Manager_Employee(Salary_Based_Employee):
#Rest data members are dervied from the super class.
bonus=0
#Constructor
def__init__(self, name, date_of_birth, starting_date, ssn, phone_number,salary,bonus):
super().__init__(name, date_of_birth, starting_date, ssn,phone_number,salary)
self.bonus = bonus
#Getter methods
defgetBonus(self):
returnself.bonus
#Setter methods
defsetBonus(self,bonus):
self.bonus = bonus
defcomputeSalary(self):
return (salary+bonus)
defprint(self):
super.print()
print("Bonus: ", self.bonus)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
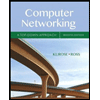
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
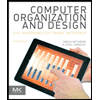
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
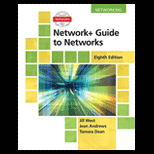
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
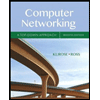
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
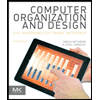
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
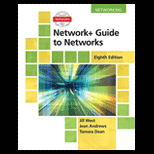
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
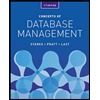
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
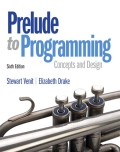
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
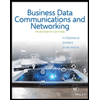
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY