a) Create a class called BankAccount which represents a bank account having the following attributes: accountNumber, name, and balance b) Create a constructor for the class BankAccount having as parameter, accountNumber, name, balance. c) Create a method called Deposit() which manages the deposit of money to the account. d) Create a method called Withdrawal() which handles cash withdrawals. e) Create a method called agio() to apply interest to a percentage of 5% of the price. f) Create a method called display() method to display account details.
a) Create a class called BankAccount which represents a bank account having the following attributes: accountNumber, name, and balance b) Create a constructor for the class BankAccount having as parameter, accountNumber, name, balance. c) Create a method called Deposit() which manages the deposit of money to the account. d) Create a method called Withdrawal() which handles cash withdrawals. e) Create a method called agio() to apply interest to a percentage of 5% of the price. f) Create a method called display() method to display account details.
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 16RQ
Related questions
Question
100%
Solve by python exercise 3
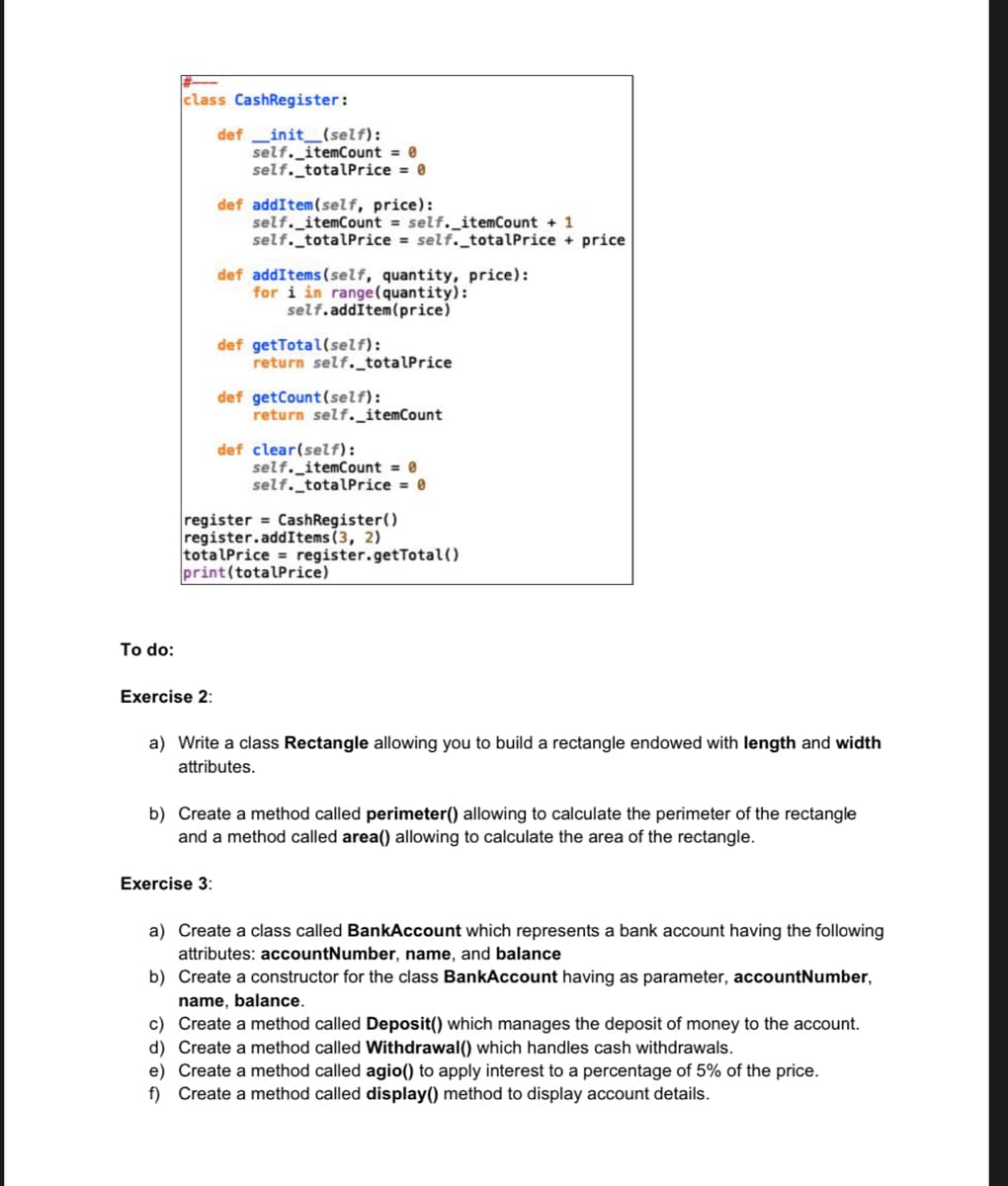
Transcribed Image Text:class CashRegister:
_init_(self):
self._itemCount = 0
self._totalPrice = 0
def
def addItem(self, price):
self._itemCount = self._itemCount + 1
self._totalPrice self._totalPrice + price
def addItems (self, quantity, price):
for i in range(quantity):
self.addItem(price)
def getTotal(self):
return self._totalPrice
def getCount(self):
return self._itemCount
def clear(self):
self._itemCount = 0
self._totalPrice = 0
register = CashRegister()
register.addItems(3, 2)
totalPrice = register.getTotal()
print(totalPrice)
To do:
Exercise 2:
a) Write a class Rectangle allowing you to build a rectangle endowed with length and width
attributes.
b) Create a method called perimeter() allowing to calculate the perimeter of the rectangle
and a method called area() allowing to calculate the area of the rectangle.
Exercise 3:
a) Create a class called BankAccount which represents a bank account having the following
attributes: accountNumber, name, and balance
b) Create a constructor for the class BankAccount having as parameter, accountNumber,
name, balance.
c) Create a method called Deposit() which manages the deposit of money to the account.
d) Create a method called Withdrawal() which handles cash withdrawals.
e) Create a method called agio() to apply interest to a percentage of 5% of the price.
f) Create a method called display() method to display account details.
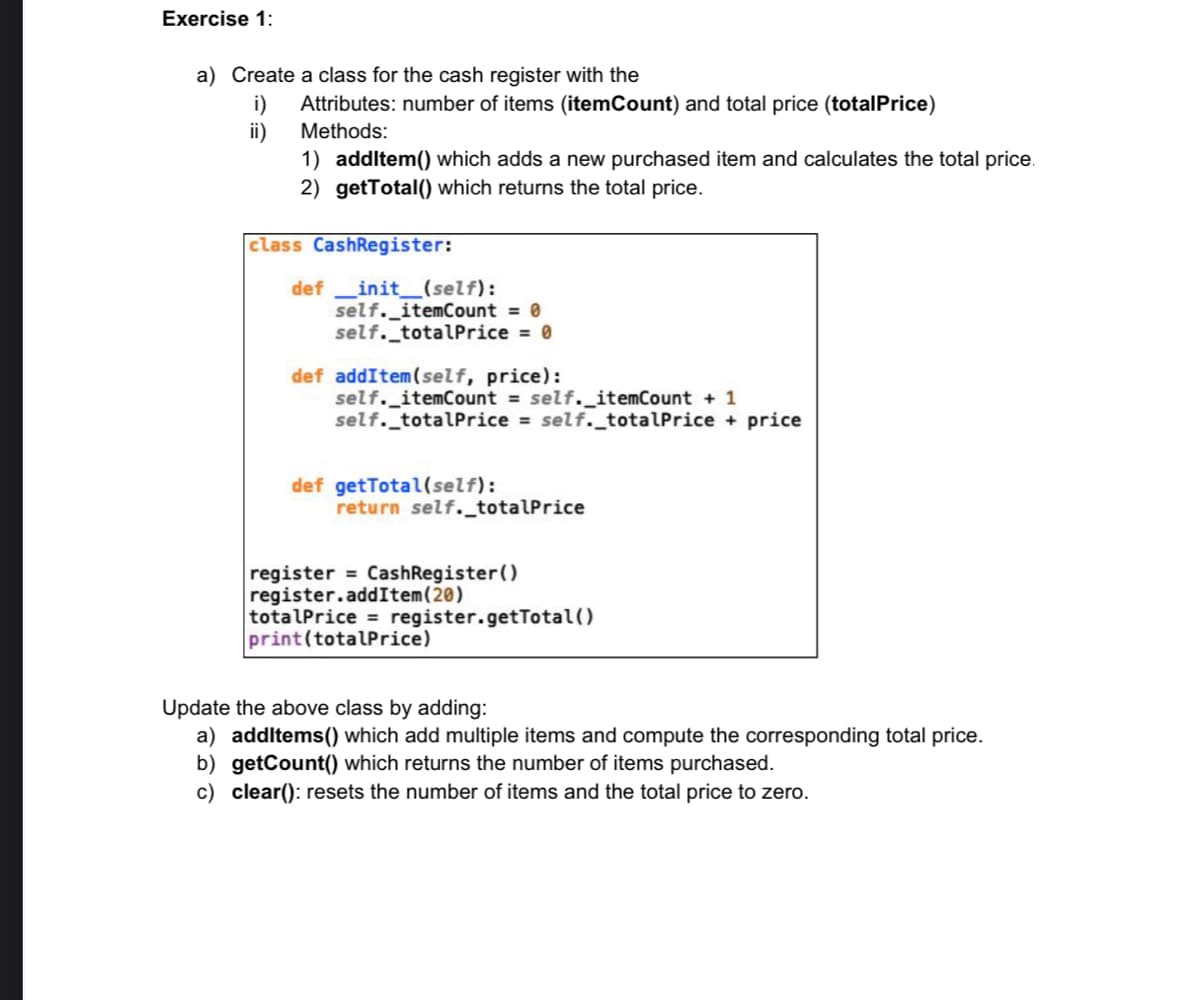
Transcribed Image Text:Exercise 1:
a) Create a class for the cash register with the
Attributes: number of items (itemCount) and total price (totalPrice)
ii)
1) addltem() which adds a new purchased item and calculates the total price.
2) getTotal() which returns the total price.
i)
Methods:
class CashRegister:
def
_init_(self):
self._itemCount = 0
self._totalPrice = 0
def addItem(self, price):
self._itemCount = self._itemCount + 1
self._totalPrice = self._totalPrice + price
def getTotal(self):
return self. totalPrice
register = CashRegister()
register.addItem(20)
totalPrice = register.getTotal()
print(totalPrice)
Update the above class by adding:
a) addltems() which add multiple items and compute the corresponding total price.
b) getCount() which returns the number of items purchased.
c) clear(): resets the number of items and the total price to zero.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
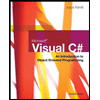
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
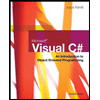
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,