Answer the given question with a proper explanation and step-by-step solution. Write a method that takes in an array of Point2D objects, and then analyzes the dataset to find points that are close together. Be sure to review the Point2D API. In your method, if the distance between any pair of points is less than 10, display the distance and the (x,y)s of each point. For example, "The distance between (3,5) and (8,9) is 6.40312." The complete API for the Point2D ADT may be viewed at http://www.ime.usp.br/~pf/sedgewick-wayne/algs4/documentation/Point2D.htmlLinks to an external site.. Do not compare a point to itself! Try to write your program directly from the API - do not review the ADT's source code. (Don't worry too much about your answer compiling - focus on using an API from its documentation.) Your answer should include only the method you wrote, do not write a complete program.
Answer the given question with a proper explanation and step-by-step solution.
Write a method that takes in an array of Point2D objects, and then analyzes the dataset to find points that are close together. Be sure to review the Point2D API. In your method, if the distance between any pair of points is less than 10, display the distance and the (x,y)s of each point. For example, "The distance between (3,5) and (8,9) is 6.40312." The complete API for the Point2D ADT may be viewed at http://www.ime.usp.br/~pf/sedgewick-wayne/algs4/documentation/Point2D.htmlLinks to an external site.. Do not compare a point to itself! Try to write your program directly from the API - do not review the ADT's source code. (Don't worry too much about your answer compiling - focus on using an API from its documentation.)
Your answer should include only the method you wrote, do not write a complete program.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

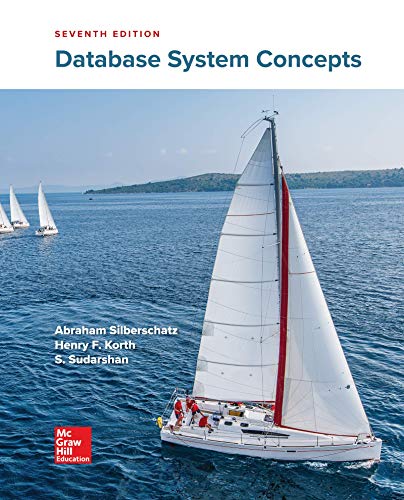
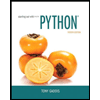
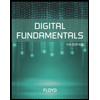
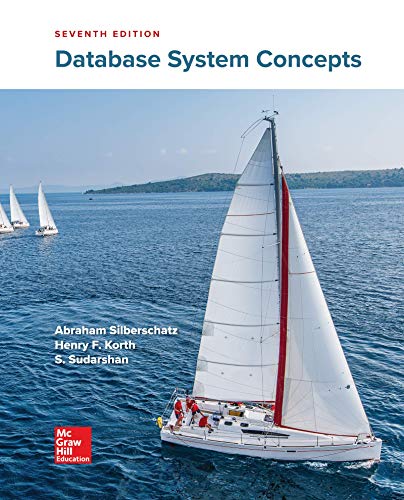
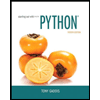
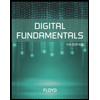
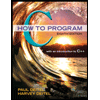
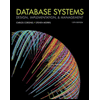
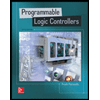