Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with my code import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin; } public void setVin(String vin) { this.vin = vin; } } public class Demo { public static void main(String[] args) throws FileNotFoundException { Scanner keyboard = new Scanner(System.in); System.out.println("Enter filename"); String input = keyboard.nextLine(); File file = new File(input); Scanner scanner = new Scanner(file); scanner.nextLine(); ArrayList list = new ArrayList<>(); while (scanner.hasNextLine()) { String[] arr = scanner.nextLine().split(" "); if (arr.length >= 4) { Car car = new Car(arr[0], arr[1], Integer.parseInt(arr[2]), arr[3]); list.add(car); } } for (int end = list.size() - 1; end >= 1; end--) { for (int current = 0; current <= end - 1; current++) { Car car1 = list.get(current); Car car2 = list.get(current + 1); int n = car1.manufacturer.toLowerCase().compareTo(car2.manufacturer.toLowerCase()); if (n == 0) { n = car1.year - car2.year; } if (n == 0) { n = car1.vin.compareTo(car2.vin); } if (n > 0) { Car temp = list.get(current); list.set(current, list.get(current + 1)); list.set(current + 1, temp); } } } // Find the oldest car for each manufacturer and print the results System.out.println("Oldest cars by make"); Car oldest = null; int count = 0; for (int i = 0; i < list.size() - 1; i++) { if (oldest == null) { oldest = list.get(i); } if (!list.get(i).manufacturer.equals(list.get(i + 1).manufacturer) || i == list.size() - 2) { if (i == list.size() - 2 && list.get(i).manufacturer.equals(list.get(i + 1).manufacturer)) { oldest = (oldest.year < list.get(i + 1).year) ? oldest : list.get(i + 1); } count++; System.out.println(String.format("%15s%25s%5s %s", oldest.manufacturer, oldest.model, oldest.year, oldest.vin)); oldest = null; } } System.out.println(count + " result(s)"); } } it is supposed to display Enter filename car-list-3.txtENTER Oldest cars by make Acura Legend 1988 YV1672MK8A2784103 Aptera Typ-1 2009 19UUA56952A698282 Aston Martin DB9 2006 JTDJTUD36ED662608 Audi 5000S 1985 JN1CV6EK1CM209730 Austin Mini Cooper 1964 1G6KD57Y86U095255 Bentley Continental GT 2006 19UUA655X4A245718 BMW 7 Series 1993 1FM5K7B80FG198521 Buick LeSabre 1986 5FRYD4H82EB970786 Cadillac Fleetwood 1992 WAUSVAFA0AN244068 Chevrolet Corvette 1957 WBA3V7C57F5095471 Chrysler Town & Country 1994 WBANU53558B610150 Daewoo Lanos 1999 2HNYD18412H169358 Daihatsu Charade 1992 JH4CL95958C112754 Dodge D150 1993 19XFA1F37BE391950 Eagle Talon 1993 WUAAU34289N943973 Ferrari F430 Spider 2006 5N1AR2MM5EC533806 Fiat 500 2012 1G6DL67A880617705 Ford Thunderbird 1965 1HGCR2F32DA757676 Geo Metro 1992 1G6KE54Y34U777569 GMC Suburban 1500 1992 19XFB2F59CE612826 Holden VS Commodore 1995 WAUBGBFC7CN714505 Honda Civic 1980 JTDKN3DU8A0870976 Hummer H1 1999 5FNRL5H20BB474763 Hyundai Sonata 2002 JA4AS2AW2DU975492 Infiniti Q 1994 WAUDH78E97A027816 Isuzu Impulse 1992 WBALM5C5XAE656665 Jaguar XJ Series 1994 1FMJK1HT9FE090336 Jeep Wrangler 1992 WA1AY94L38D656200 Kia Sephia 2001 3GYFNCEY3BS991891 ......... Volkswagen Beetle 1965 3GYFNKE6XBS962560 Volvo 740 1992 1G6AB5SX1F0577055 56 result(s) however, it is displaying Enter filename car-list-3.txtENTER Oldest cars by make 0 result(s) Can an expert help explain what error is in my code and fix it so that it works accordingly
Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN.
I am having trouble with my code
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin; } public void setVin(String vin) { this.vin = vin; } } public class Demo { public static void main(String[] args) throws FileNotFoundException { Scanner keyboard = new Scanner(System.in); System.out.println("Enter filename"); String input = keyboard.nextLine(); File file = new File(input); Scanner scanner = new Scanner(file); scanner.nextLine(); ArrayList<Car> list = new ArrayList<>(); while (scanner.hasNextLine()) { String[] arr = scanner.nextLine().split(" "); if (arr.length >= 4) { Car car = new Car(arr[0], arr[1], Integer.parseInt(arr[2]), arr[3]); list.add(car); } } for (int end = list.size() - 1; end >= 1; end--) { for (int current = 0; current <= end - 1; current++) { Car car1 = list.get(current); Car car2 = list.get(current + 1); int n = car1.manufacturer.toLowerCase().compareTo(car2.manufacturer.toLowerCase()); if (n == 0) { n = car1.year - car2.year; } if (n == 0) { n = car1.vin.compareTo(car2.vin); } if (n > 0) { Car temp = list.get(current); list.set(current, list.get(current + 1)); list.set(current + 1, temp); } } } // Find the oldest car for each manufacturer and print the results System.out.println("Oldest cars by make"); Car oldest = null; int count = 0; for (int i = 0; i < list.size() - 1; i++) { if (oldest == null) { oldest = list.get(i); } if (!list.get(i).manufacturer.equals(list.get(i + 1).manufacturer) || i == list.size() - 2) { if (i == list.size() - 2 && list.get(i).manufacturer.equals(list.get(i + 1).manufacturer)) { oldest = (oldest.year < list.get(i + 1).year) ? oldest : list.get(i + 1); } count++; System.out.println(String.format("%15s%25s%5s %s", oldest.manufacturer, oldest.model, oldest.year, oldest.vin)); oldest = null; } } System.out.println(count + " result(s)"); } }
it is supposed to display
Enter filename
car-list-3.txtENTER
Oldest cars by make
Acura Legend 1988 YV1672MK8A2784103
Aptera Typ-1 2009 19UUA56952A698282
Aston Martin DB9 2006 JTDJTUD36ED662608
Audi 5000S 1985 JN1CV6EK1CM209730
Austin Mini Cooper 1964 1G6KD57Y86U095255
Bentley Continental GT 2006 19UUA655X4A245718
BMW 7 Series 1993 1FM5K7B80FG198521
Buick LeSabre 1986 5FRYD4H82EB970786
Cadillac Fleetwood 1992 WAUSVAFA0AN244068
Chevrolet Corvette 1957 WBA3V7C57F5095471
Chrysler Town & Country 1994 WBANU53558B610150
Daewoo Lanos 1999 2HNYD18412H169358
Daihatsu Charade 1992 JH4CL95958C112754
Dodge D150 1993 19XFA1F37BE391950
Eagle Talon 1993 WUAAU34289N943973
Ferrari F430 Spider 2006 5N1AR2MM5EC533806
Fiat 500 2012 1G6DL67A880617705
Ford Thunderbird 1965 1HGCR2F32DA757676
Geo Metro 1992 1G6KE54Y34U777569
GMC Suburban 1500 1992 19XFB2F59CE612826
Holden VS Commodore 1995 WAUBGBFC7CN714505
Honda Civic 1980 JTDKN3DU8A0870976
Hummer H1 1999 5FNRL5H20BB474763
Hyundai Sonata 2002 JA4AS2AW2DU975492
Infiniti Q 1994 WAUDH78E97A027816
Isuzu Impulse 1992 WBALM5C5XAE656665
Jaguar XJ Series 1994 1FMJK1HT9FE090336
Jeep Wrangler 1992 WA1AY94L38D656200
Kia Sephia 2001 3GYFNCEY3BS991891
.........
Volkswagen Beetle 1965 3GYFNKE6XBS962560
Volvo 740 1992 1G6AB5SX1F0577055
56 result(s)
however, it is displaying
Enter filename
car-list-3.txtENTER
Oldest cars by make
0 result(s)
Can an expert help explain what error is in my code and fix it so that it works accordingly

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

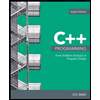
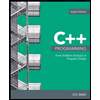