ass Company: A company object stores the company name, company payments as an array of Payab number of payments. o Include a constructor that receives a company name, constructs the payments array and a constant maximum array capacity of your choice. o Include a method addPayment that receives a string value representing a payment "invoice"), It then reads the appropriate payment information from the user (assume one and adds this payment to the payments array. o Include a method printDuePayments that prints the company name, the payments infe element and the total due payments, as illustrated in the given sample run. o No setters or getters are requested for this class.
ass Company: A company object stores the company name, company payments as an array of Payab number of payments. o Include a constructor that receives a company name, constructs the payments array and a constant maximum array capacity of your choice. o Include a method addPayment that receives a string value representing a payment "invoice"), It then reads the appropriate payment information from the user (assume one and adds this payment to the payments array. o Include a method printDuePayments that prints the company name, the payments infe element and the total due payments, as illustrated in the given sample run. o No setters or getters are requested for this class.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Solve by Java
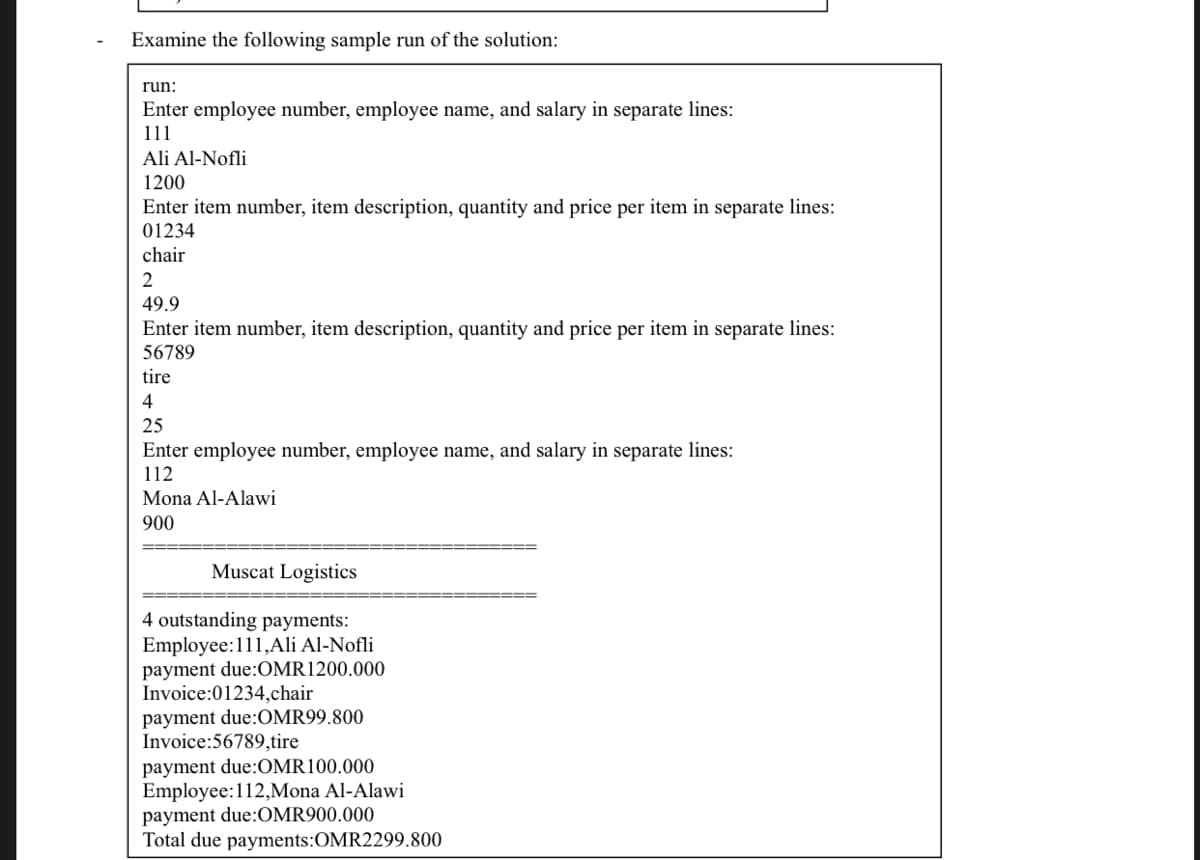
Transcribed Image Text:Examine the following sample run of the solution:
run:
Enter employee number, employee name, and salary in separate lines:
111
Ali Al-Nofli
1200
Enter item number, item description, quantity and price per item in separate lines:
01234
chair
2
49.9
Enter item number, item description, quantity and price per item in separate lines:
56789
tire
4
25
Enter employee number, employee name, and salary in separate lines:
112
Mona Al-Alawi
900
Muscat Logistics
4 outstanding payments:
Employee:111,Ali Al-Nofli
payment due:OMR1200.000
Invoice:01234.chair
payment due:OMR99.800
Invoice:56789,tire
payment due:OMR100.000
Employee:112,Mona Al-Alawi
payment due:OMR900.000
Total due payments:OMR2299.800
![Problem:
A company keeps track of its monthly payments that can include purchase invoices and employee salaries. You are requested to
develop an OOP solution that computes a company payments of invoices and employee salaries. Create a NetBeans project named
Labtest Yourld. Include the following interfaces and classes.
Interface Payable that includes two paramterless methods:
o getPaymentAmount: should return a double value.
o print: No return value
Class Employee:
o An employee has an employee number, name and salary.
o Include one method setEmployee that receives employee information as parameters and use it to set the Employee
object data.
Class Employee should implement the Payable interface, as follows:
• It returns the salary as the payment amount.
It prints on screen the employee number and name (e.g. "Employee: 111,Ali Al-Nofli"). See the provided
sample run.
No constructors, setters or getters are requested for this class.
Class Invoice:
An invoice has an item number, item description, quantity and price per item.
Include one method setInvoice that receives an invoice information as parameters and use it to set the Invoice
object data.
Class Invoice should implement the Payable interface, as follows:
It returns the invoice amount (i.e. quantity X price per item) as the payment amount.
It prints on screen the item number and item description (e.g. "Invoice: 01234, chair). See the provided
sample run.
o No constructors, setters or getters are requested for this class.
Class Company:
A company object stores the company name, company payments as an array of Payable objects, and the current
number of payments.
o Include a constructor that receives a company name, constructs the payments array and initializes class data. Use
a constant maximum array capacity of your choice.
Include a method addPayment that receives a string value representing a payment type (e.g. "employee", or
"invoice"), It then reads the appropriate payment information from the user (assume one value is entered per line),
and adds this payment to the payments array.
o Include a method printDuePayments that prints the company name, the payments information, the payment per
element and the total due payments, as illustrated in the given sample run.
No setters or getters are requested for this class.
Add (and run) the following tester code to the main class of your labtest project (Note: do not change the tester code):
Page 1 of 2
public static void main(String[] args) {
Company company = new Company("Muscat Logistics");
company.addPayment("employee");
company.addPayment("invoice");
company.addPayment("invoice");
company.addPayment("employee");
company.printDuePayments();
}//main
Examine the following sample run of the solution:
run:
Enter employee number, employee name, and salary in separate lines:
111
Ali Al-Nofli
1200
Enter item number, item description, quantity and price per item in separate lines:
01234
chair
2
49.9
Enter item number, item description, quantity and price per item in separate lines:
56789
tire
4
25
Enter employee number, employee name, and salary in separate lines:
112
Mona Al-Alawi](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8568a871-2ffa-45f0-9492-98ceb881074c%2F92dcd494-cb87-4e74-8b8a-75e29215b35e%2Fztpfoai_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem:
A company keeps track of its monthly payments that can include purchase invoices and employee salaries. You are requested to
develop an OOP solution that computes a company payments of invoices and employee salaries. Create a NetBeans project named
Labtest Yourld. Include the following interfaces and classes.
Interface Payable that includes two paramterless methods:
o getPaymentAmount: should return a double value.
o print: No return value
Class Employee:
o An employee has an employee number, name and salary.
o Include one method setEmployee that receives employee information as parameters and use it to set the Employee
object data.
Class Employee should implement the Payable interface, as follows:
• It returns the salary as the payment amount.
It prints on screen the employee number and name (e.g. "Employee: 111,Ali Al-Nofli"). See the provided
sample run.
No constructors, setters or getters are requested for this class.
Class Invoice:
An invoice has an item number, item description, quantity and price per item.
Include one method setInvoice that receives an invoice information as parameters and use it to set the Invoice
object data.
Class Invoice should implement the Payable interface, as follows:
It returns the invoice amount (i.e. quantity X price per item) as the payment amount.
It prints on screen the item number and item description (e.g. "Invoice: 01234, chair). See the provided
sample run.
o No constructors, setters or getters are requested for this class.
Class Company:
A company object stores the company name, company payments as an array of Payable objects, and the current
number of payments.
o Include a constructor that receives a company name, constructs the payments array and initializes class data. Use
a constant maximum array capacity of your choice.
Include a method addPayment that receives a string value representing a payment type (e.g. "employee", or
"invoice"), It then reads the appropriate payment information from the user (assume one value is entered per line),
and adds this payment to the payments array.
o Include a method printDuePayments that prints the company name, the payments information, the payment per
element and the total due payments, as illustrated in the given sample run.
No setters or getters are requested for this class.
Add (and run) the following tester code to the main class of your labtest project (Note: do not change the tester code):
Page 1 of 2
public static void main(String[] args) {
Company company = new Company("Muscat Logistics");
company.addPayment("employee");
company.addPayment("invoice");
company.addPayment("invoice");
company.addPayment("employee");
company.printDuePayments();
}//main
Examine the following sample run of the solution:
run:
Enter employee number, employee name, and salary in separate lines:
111
Ali Al-Nofli
1200
Enter item number, item description, quantity and price per item in separate lines:
01234
chair
2
49.9
Enter item number, item description, quantity and price per item in separate lines:
56789
tire
4
25
Enter employee number, employee name, and salary in separate lines:
112
Mona Al-Alawi
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
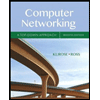
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
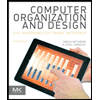
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
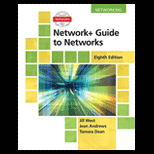
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
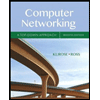
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
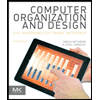
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
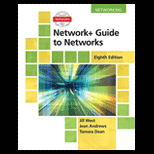
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
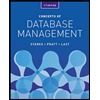
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
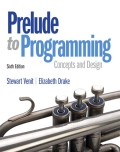
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
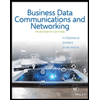
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY