Class Gradebook1D Write an application that prints out the final grade of the students in a class and the average for the whole class. There are a total of 3 quizzes. You will need 4 arrays: 1. An array of type int to store all the ID's 2. An array of type double to store all scores for quiz 1 3. An array of type double to store all scores for quiz 2 4. An array of type double to store all scores for quiz 3 Example: Assume there are 10 students in the class, then the id array will have 10 indices. 2 3 132 451 854 523 153 588 351 856 142 445 Each quiz array will store in the index that corresponds to the student the score for the quiz. 3 100.0 90.0 85.0 92.0 75.0 93.0 98.0 100.0 95.0 87.0 90.0 87.0 92.0 95.0 78.0 84.0 88.0 96.0 100.0 95.0 3 100.0 98.0 85.0 98.0 87.0 89.0 92.0 88.0 92.0 97.0 Student with ID 153, at index 4, has taken all three quizzes with scores 75.0 (quiz 1), 78.0 (quiz 2) and 87.0 (quiz 3). How to proceed: 1. Ask the user how many students are in the class, so you can set the length of all the arrays. 2. Allocate 4 arrays that will store the data. 3. Use a FOR loop to retrieve and store all the data. 4. Use another FOR loop to a. Output the final score for each student. b. Keep track of all scores to later compute the average for the class. 5. Calculate and Output the average for the class. Format all floating-point numbers to 2 decimal places. Sample Output: How many students in the class? 4 Enter student ID: 111 Enter score for Quiz 1: 100 Enter score for Quiz 2: 95 Enter score for Quiz 3: 90 Enter student ID: 222 Enter score for Quiz 1: 90 Enter score for Quiz 2: 85 Enter score for Quiz 3: 80 Enter student ID: 333 Enter score for Quiz 1: 100 Enter score for Quiz 2: 100 Enter score for Quiz 3: 100 Enter student ID: 444 Enter score for Quiz 11 90 Enter score for Quiz 21 90 Enter score for Quiz 3: 90 ID 111 - Final grade: 95.00 ID 222 - Final grade: 85.00 ID 333 - Final grade: 100.00 ID 444 - Final grade: 90.00 Class average: 92.50 Your program MUST receive user input as shown in the sample output.
Class Gradebook1D Write an application that prints out the final grade of the students in a class and the average for the whole class. There are a total of 3 quizzes. You will need 4 arrays: 1. An array of type int to store all the ID's 2. An array of type double to store all scores for quiz 1 3. An array of type double to store all scores for quiz 2 4. An array of type double to store all scores for quiz 3 Example: Assume there are 10 students in the class, then the id array will have 10 indices. 2 3 132 451 854 523 153 588 351 856 142 445 Each quiz array will store in the index that corresponds to the student the score for the quiz. 3 100.0 90.0 85.0 92.0 75.0 93.0 98.0 100.0 95.0 87.0 90.0 87.0 92.0 95.0 78.0 84.0 88.0 96.0 100.0 95.0 3 100.0 98.0 85.0 98.0 87.0 89.0 92.0 88.0 92.0 97.0 Student with ID 153, at index 4, has taken all three quizzes with scores 75.0 (quiz 1), 78.0 (quiz 2) and 87.0 (quiz 3). How to proceed: 1. Ask the user how many students are in the class, so you can set the length of all the arrays. 2. Allocate 4 arrays that will store the data. 3. Use a FOR loop to retrieve and store all the data. 4. Use another FOR loop to a. Output the final score for each student. b. Keep track of all scores to later compute the average for the class. 5. Calculate and Output the average for the class. Format all floating-point numbers to 2 decimal places. Sample Output: How many students in the class? 4 Enter student ID: 111 Enter score for Quiz 1: 100 Enter score for Quiz 2: 95 Enter score for Quiz 3: 90 Enter student ID: 222 Enter score for Quiz 1: 90 Enter score for Quiz 2: 85 Enter score for Quiz 3: 80 Enter student ID: 333 Enter score for Quiz 1: 100 Enter score for Quiz 2: 100 Enter score for Quiz 3: 100 Enter student ID: 444 Enter score for Quiz 11 90 Enter score for Quiz 21 90 Enter score for Quiz 3: 90 ID 111 - Final grade: 95.00 ID 222 - Final grade: 85.00 ID 333 - Final grade: 100.00 ID 444 - Final grade: 90.00 Class average: 92.50 Your program MUST receive user input as shown in the sample output.
Chapter12: Exception Handling
Section: Chapter Questions
Problem 8PE
Related questions
Question
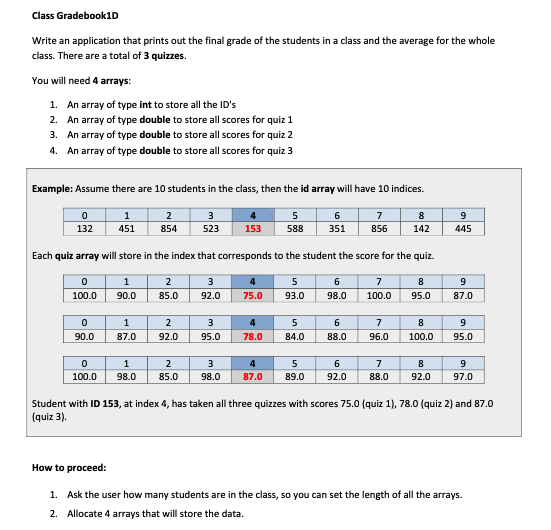
Transcribed Image Text:Class Gradebook1D
Write an application that prints out the final grade of the students in a class and the average for the whole
class. There are a total of 3 quizzes.
You will need 4 arrays:
1. An array of type int to store all the ID's
2. An array of type double to store all scores for quiz 1
3. An array of type double to store all scores for quiz 2
4. An array of type double to store all scores for quiz 3
Example: Assume there are 10 students in the class, then the id array will have 10 indices.
2
3
132
451
854
523
153
588
351
856
142
445
Each quiz array will store in the index that corresponds to the student the score for the quiz.
3
100.0
90.0
85.0
92.0
75.0
93.0
98.0
100.0
95.0
87.0
90.0
87.0
92.0
95.0
78.0
84.0
88.0
96.0
100.0
95.0
3
100.0
98.0
85.0
98.0
87.0
89.0
92.0
88.0
92.0
97.0
Student with ID 153, at index 4, has taken all three quizzes with scores 75.0 (quiz 1), 78.0 (quiz 2) and 87.0
(quiz 3).
How to proceed:
1. Ask the user how many students are in the class, so you can set the length of all the arrays.
2. Allocate 4 arrays that will store the data.
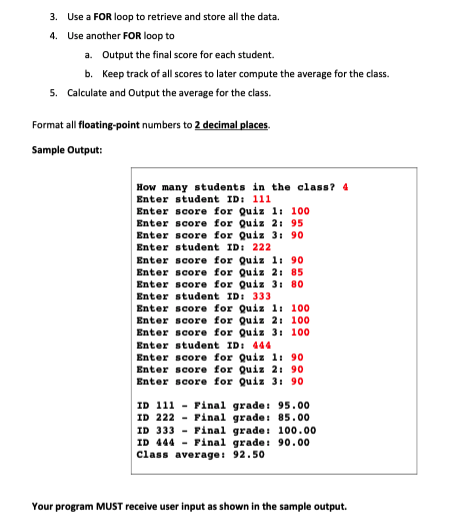
Transcribed Image Text:3. Use a FOR loop to retrieve and store all the data.
4. Use another FOR loop to
a. Output the final score for each student.
b. Keep track of all scores to later compute the average for the class.
5. Calculate and Output the average for the class.
Format all floating-point numbers to 2 decimal places.
Sample Output:
How many students in the class? 4
Enter student ID: 111
Enter score for Quiz 1: 100
Enter score for Quiz 2: 95
Enter score for Quiz 3: 90
Enter student ID: 222
Enter score for Quiz 1: 90
Enter score for Quiz 2: 85
Enter score for Quiz 3: 80
Enter student ID: 333
Enter score for Quiz 1: 100
Enter score for Quiz 2: 100
Enter score for Quiz 3: 100
Enter student ID: 444
Enter score for Quiz 11 90
Enter score for Quiz 21 90
Enter score for Quiz 3: 90
ID 111 - Final grade: 95.00
ID 222 - Final grade: 85.00
ID 333 - Final grade: 100.00
ID 444 - Final grade: 90.00
Class average: 92.50
Your program MUST receive user input as shown in the sample output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
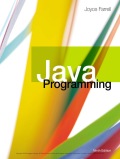
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
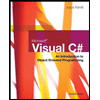
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
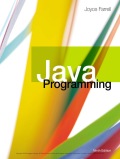
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
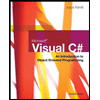
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,