Assignment: Check the Sample run first, then carefully read the instructions and write aprogram that prompts the user for first name, last name, then secret number. The output willgenerate a default email address and a default password. 1. The main method will do the following:• Asks the user for first name, last name, and secret number using Scanner. (first lettercan be in upper case)• Call defaultInfo(String, String) method to PRINT default Kean email address.• Call defaultInfo(String, int) method to PRINT default Kean password.• Note: You are using overloaded methods (i.e., same method name with differentparameter lists) 2. Write an overloaded method, defaultInfo, which does the following:• public static void defaultInfo(String firstName, String lastName) [Note:you can use your own variable name]‒ print default email address all in low caps: concatenate first letter offirstName, full lastName, and @gmail.com. [Hint: string.charAt(index),string.toLowerCase()]• public static void defaultInfo(String firstName, int secret) [Note: Youcan use your own variable name]‒ print default password all in low caps: concatenate the full firstName in reverseorder and the secret number). [Hint: use a loop, string.length(),string.charAt(index), string.toLowerCase()] Two Sample Runs:Enter first name: DaehanEnter last name: KwakEnter secret number: 2018Kean email: dkwak@gmail.comKean password: nahead2018 Enter first name: MichealEnter last name: JordanEnter secret number: 23Kean email: mjordan@gmail.comKean password: laehcim23 Useful Hints• Notes on toLowerCase (from ppt slide Ch4-2 and Ch4-3)s.toLowerCase() Returns a new string with all letters in string s lowercase.Character.toLowerCase(ch) Returns the lowercase of the specified character ch. • String Concatenation (from ppt slide Ch4-3)String s1 = "Mississippi";String s2 = "River";String s3 = s1.concat(s2);orString s3 = s1 + s2;System.out.println( s3 ); //will both print: MississippiRiver // Three strings are concatenatedString message = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2String s = "Chapter" + 2; // s becomes Chapter2 // String Supplement is concatenated with character BString s1 = "Supplement" + 'B'; // s1 becomes SupplementB • String Concatenation (from ppt slide Ch4-3)Recall that index starts from 0 up to (endIndex – 1)
Assignment: Check the Sample run first, then carefully read the instructions and write a
program that prompts the user for first name, last name, then secret number. The output will
generate a default email address and a default password.
1. The main method will do the following:
• Asks the user for first name, last name, and secret number using Scanner. (first letter
can be in upper case)
• Call defaultInfo(String, String) method to PRINT default Kean email address.
• Call defaultInfo(String, int) method to PRINT default Kean password.
• Note: You are using overloaded methods (i.e., same method name with different
parameter lists)
2. Write an overloaded method, defaultInfo, which does the following:
• public static void defaultInfo(String firstName, String lastName) [Note:
you can use your own variable name]
‒ print default email address all in low caps: concatenate first letter of
firstName, full lastName, and @gmail.com. [Hint: string.charAt(index),
string.toLowerCase()]
• public static void defaultInfo(String firstName, int secret) [Note: You
can use your own variable name]
‒ print default password all in low caps: concatenate the full firstName in reverse
order and the secret number). [Hint: use a loop, string.length(),
string.charAt(index), string.toLowerCase()]
Two Sample Runs:
Enter first name: Daehan
Enter last name: Kwak
Enter secret number: 2018
Kean email: dkwak@gmail.com
Kean password: nahead2018
Enter first name: Micheal
Enter last name: Jordan
Enter secret number: 23
Kean email: mjordan@gmail.com
Kean password: laehcim23
Useful Hints
• Notes on toLowerCase (from ppt slide Ch4-2 and Ch4-3)
s.toLowerCase() Returns a new string with all letters in string s lowercase.
Character.toLowerCase(ch) Returns the lowercase of the specified character ch.
• String Concatenation (from ppt slide Ch4-3)
String s1 = "Mississippi";
String s2 = "River";
String s3 = s1.concat(s2);
or
String s3 = s1 + s2;
System.out.println( s3 ); //will both print: MississippiRiver
// Three strings are concatenated
String message = "Welcome " + "to " + "Java";
// String Chapter is concatenated with number 2
String s = "Chapter" + 2; // s becomes Chapter2
// String Supplement is concatenated with character B
String s1 = "Supplement" + 'B'; // s1 becomes SupplementB
• String Concatenation (from ppt slide Ch4-3)
Recall that index starts from 0 up to (endIndex – 1)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

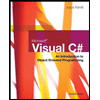
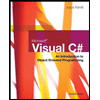