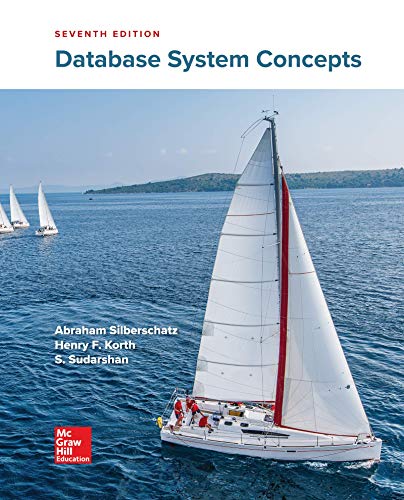
import java.util.Scanner;
public class Main {
static Scanner kb = new Scanner(System.in);
public static void displayMenu() {
System.out.println("a-Print Name and Tutor Name");
System.out.println("b-Largest of 3 Number");
System.out.println("c-Display Numbers in range of two numbers");
System.out.println("d-Check for Triangles");
System.out.println("e-Check for prime");
System.out.println("f-Arrays Example"); //adding statement
System.out.println("q-Quit");
System.out.print("Enter Your Choice (a,b,c,d,e,f,q) : ");
}
public static void findLargestOf3() {
double x, y, z, smallest, largest;
System.out.println("Enter 3 Numbers : ");
System.out.print("x : ");
x = Double.parseDouble(kb.nextLine());
System.out.print("y : ");
y = Double.parseDouble(kb.nextLine());
System.out.print("z : ");
z = Double.parseDouble(kb.nextLine());
smallest = (x < y && x < z) ? x : (y < x && y < z) ? y : z;
largest = (x > y && x > z) ? x : (y > x && y > z) ? y : z;
System.out.println("The Smallest Number is : " + smallest);
System.out.println("The Largest Number is : " + largest);
}
public static void displayNumbersinRange() {
int m, n, s = 0, t;
System.out.println("Enter 2 Numbers : ");
System.out.print("m : ");
m = Integer.parseInt(kb.nextLine());
System.out.print("n : ");
n = Integer.parseInt(kb.nextLine());
if (m > n) {
t = n;
n = m;
m = t;
}
for (int i = m, j = 1; i < n; i++, j++) {
System.out.print(i + " ");
if (j % 5 == 0)
System.out.println();
if (i % 2 != 0)
s += i;
}
System.out.println("\nThe Sum of all odd numbers between " + m + " and " + n + " is : " + s);
}
public static void checkTriangle() {
double s1, s2, s3;
System.out.println("Enter 3 Sides of Triangle : ");
System.out.print("s1 : ");
s1 = Double.parseDouble(kb.nextLine());
System.out.print("s2 : ");
s2 = Double.parseDouble(kb.nextLine());
System.out.print("s3 : ");
s3 = Double.parseDouble(kb.nextLine());
if (s1 > s2 + s3 || s2 > s1 + s3 || s3 > s1 + s2) {
System.out.println("The Given sides can from a Triangle");
} else
System.out.println("The Given sides can not from a Triangle");
}
public static void checkPrime() {
int m, c = 0;
System.out.print("Enter a Numbers : ");
m = Integer.parseInt(kb.nextLine());
for (int i = 1; i <= m; i++) {
if (m % i == 0)
c++;
}
if (c == 2)
System.out.println("The given number is Prime");
else
System.out.println("The given number is not a Prime");
}
public static void main(String[] args) {
char ch = 'x';
while (ch != 'q') {
displayMenu();
ch = kb.nextLine().toLowerCase().charAt(0);
switch (ch) {
case 'a':
System.out.println("My Name \n My Tutor Name");
break;
case 'b':
findLargestOf3();
break;
case 'c':
displayNumbersinRange();
break;
case 'd':
checkTriangle();
break;
case 'e':
checkPrime();
break;
case 'f': //adding another case
newFunction();
break;
case 'q':
break;
default:
System.out.println("Error : Invalid Input ");
break;
}
}
}
public static void newFunction() {
int[] array=new int[10]; //declare array
System.out.println("Enter 10 elements into array");
for(int i=0;i<10;i++) //i from 0 to 9
array[i] = Integer.parseInt(kb.nextLine()); //input element at index i
int min=array[0], max=array[0], sum=array[0]; //initialize all variables
for(int i=1;i<10;i++) { //i from 1 to 9
if(array[i]<min) //if element at index i less than min
min=array[i];
if(array[i]>max) //if element at index i more than max
max=array[i];
sum=sum+array[i]; //add element at index i to sum
}
//print all values
System.out.println("Average of 10 numbers is: "+(sum/10.0));
System.out.println("Highest number is: "+max);
System.out.println("Lowest number is: "+min);
}
}

Step by stepSolved in 3 steps with 1 images

- The method lower() returns a string with all the characters converted to lowercase. True or Falsearrow_forwardIn Programming When you work with text, sometimes you need to determine if a given string starts with or ends with certain characters. You can use two string methods to solve this problem: .startswith() and .endswith()?arrow_forwardMad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Write a program that takes a string and an integer as input, and outputs a sentence using the input values as shown in the example below. The program repeats until the input string is quit and disregards the integer input that follows. Ex: If the input is: apples 5 shoes 2 quit 0 the output is: Eating 5 apples a day keeps the doctor away. Eating 2 shoes a day keeps the doctor away.arrow_forward
- Baby btc program - python Budget: $75,000 Make a string based bitcoin menu which shows the price of a bitcoin (pseudo random price), able to buy bitcoin with $75,000 budget (say "buy 0.5" meaning buy half of a bitcoin or "buy 3000" meaning buy $3000 worth of bitcoin), able to sell their bitcoin currently owned (using same format), can print their balance (show their current USD, the number of bitcoins they have in their wallet, and USD value of the bitcoins they have), and able to print their buy/sell transaction history.arrow_forwardIn programming you work with text, sometimes you need to determine if a given string starts with or ends with certain characters. You can use two string methods to solve this problem: .startswith() and .endswith()?arrow_forwardWrite a complete program to generate a username based on a user's first and last name. Part One: Get a Valid First and Last Name ask the user for and read in their first and last name use a loop to validate the first name: it must be at least one character long and it must start with a letter use a loop to validate the last name: it must not contain any spaces Hint: use a nested loop. The outer loop will iterate until there is valid input. The inner loop will check each character in the String to see if any of them are whitespace. Part Two: Generate a Username generate a two-digit random number (i.e., a random number between 10 and 99, inclusive) create a username that is the following data concatenated: the first letter of their first name in lower case the first five characters of their last name in lower case; if the name is shorter than five characters, use the whole last name the two-digit number output the username to the user Additional Coding Requirements code…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
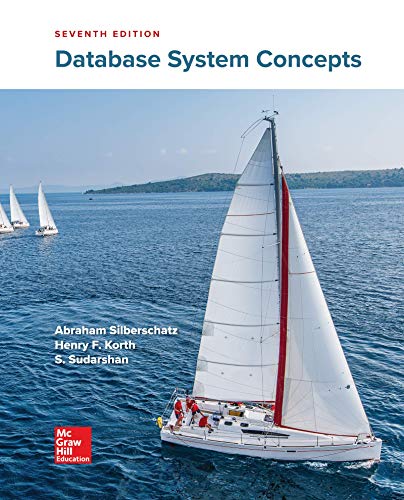
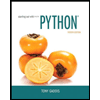
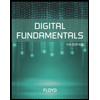
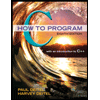
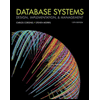
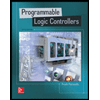