I need to format the date and time to read out month first then the day then the year but it is not working, what is the correct format? Also my hours are not updating when it runs, why? import java.time.format.DateTimeFormatter; import java.time.LocalDate; import java.util.Scanner; public class Volunteer { private String firstName; private String lastName; private static int startDate; private double volunteerHours; public static final String DEFAULT_FIRST_NAME = "first name not assigned"; public static final String DEFAULT_LAST_NAME = "last name not assigned"; public static final LocalDate DEFAULT_START_DATE = LocalDate.now(); public static final double DEFAULT_HOURS = 0; public Volunteer(String firstName, String lastName, int startDate2, double volunteerHours) { // TODO Auto-generated constructor stub } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { if (firstName != null && firstName.length() >0 ) this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastname(String lastName) { if (lastName != null && lastName.length() >0 ) this.lastName = lastName; } public int getStartDate() { return startDate; } public void setStartDate(int StartDate) { if (startDate != 0) Volunteer.startDate = StartDate; } public void setStartDate(int newYear, int newMonth, int newDay) { } public double getVolunteerHours(double hours) { return volunteerHours; } public void setVolunteerHours(double volunteerHours) { this.volunteerHours = volunteerHours; } public void updateVolunteerHours (double hours) { // this.volunteerHours=hours; this.volunteerHours = this.volunteerHours+hours; } public static String getDefaultFirstName() { return DEFAULT_FIRST_NAME; } public static String getDefaultLastName() { return DEFAULT_LAST_NAME; } public static LocalDate getDefaultStartDate() { return DEFAULT_START_DATE; } public static double getDefaultHours() { return DEFAULT_HOURS; } @Override public String toString() { return "Volunteers [firstName=" + firstName + ", lastName=" + lastName + ", startDate=" + startDate + ", volunteerHours=" + volunteerHours + "]"; } public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); System.out.print("Enter First Name: "); String firstName = keyboard.nextLine(); System.out.print("Enter Last Name: "); String lastName = keyboard.nextLine(); System.out.print("Enter the start date: (mm/dd/yyy)"); String userInput = keyboard.nextLine(); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy/d/M"); LocalDate date = LocalDate.parse(userInput, formatter); System.out.println(date); System.out.print("Enter Hours: ");{ double volunteerHours= keyboard.nextDouble(); Volunteer vol = new Volunteer(firstName,lastName,startDate,volunteerHours); System.out.println(vol); keyboard.close(); } } }
I need to format the date and time to read out month first then the day then the year but it is not working, what is the correct format? Also my hours are not updating when it runs, why?
import java.time.format.DateTimeFormatter;
import java.time.LocalDate;
import java.util.Scanner;
public class Volunteer {
private String firstName;
private String lastName;
private static int startDate;
private double volunteerHours;
public static final String DEFAULT_FIRST_NAME = "first name not assigned";
public static final String DEFAULT_LAST_NAME = "last name not assigned";
public static final LocalDate DEFAULT_START_DATE = LocalDate.now();
public static final double DEFAULT_HOURS = 0;
public Volunteer(String firstName, String lastName, int startDate2, double volunteerHours) {
// TODO Auto-generated constructor stub
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
if (firstName != null && firstName.length() >0 )
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastname(String lastName) {
if (lastName != null && lastName.length() >0 )
this.lastName = lastName;
}
public int getStartDate() {
return startDate;
}
public void setStartDate(int StartDate) {
if (startDate != 0)
Volunteer.startDate = StartDate;
}
public void setStartDate(int newYear, int newMonth, int newDay) {
}
public double getVolunteerHours(double hours) {
return volunteerHours;
}
public void setVolunteerHours(double volunteerHours) {
this.volunteerHours = volunteerHours;
}
public void updateVolunteerHours (double hours) {
// this.volunteerHours=hours;
this.volunteerHours = this.volunteerHours+hours;
}
public static String getDefaultFirstName() {
return DEFAULT_FIRST_NAME;
}
public static String getDefaultLastName() {
return DEFAULT_LAST_NAME;
}
public static LocalDate getDefaultStartDate() {
return DEFAULT_START_DATE;
}
public static double getDefaultHours() {
return DEFAULT_HOURS;
}
@Override
public String toString() {
return "Volunteers [firstName=" + firstName + ", lastName=" + lastName + ", startDate=" + startDate
+ ", volunteerHours=" + volunteerHours + "]";
}
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
System.out.print("Enter First Name: ");
String firstName = keyboard.nextLine();
System.out.print("Enter Last Name: ");
String lastName = keyboard.nextLine();
System.out.print("Enter the start date: (mm/dd/yyy)");
String userInput = keyboard.nextLine();
DateTimeFormatter formatter =
DateTimeFormatter.ofPattern("yyyy/d/M");
LocalDate date = LocalDate.parse(userInput, formatter);
System.out.println(date);
System.out.print("Enter Hours: ");{
double volunteerHours= keyboard.nextDouble();
Volunteer vol = new Volunteer(firstName,lastName,startDate,volunteerHours);
System.out.println(vol);
keyboard.close();
}
}
}

explanation:
you can use the simple date format like this:
SimpleDateFormat sdf = new SimpleDateFormat("mm/dd/yyyy");
Date date = sdf.parse(userInput);
sdf = new SimpleDateFormat("MM/dd/yyyy");
System.out.println(sdf.format(date));
below is modified code with output:
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

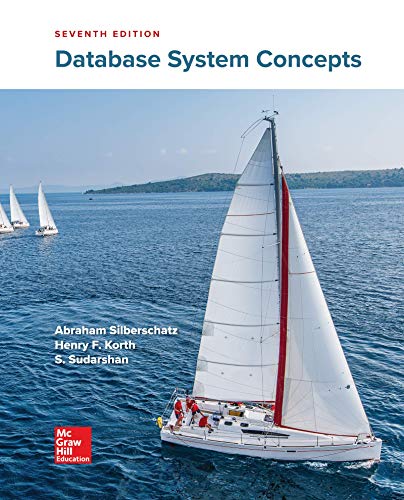
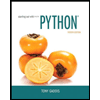
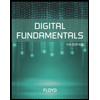
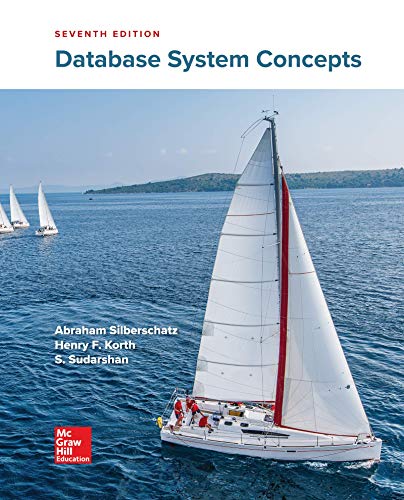
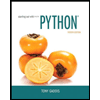
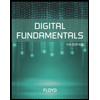
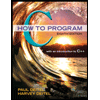
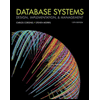
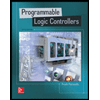