Assume that a gallon of paint covers about 350 square feet of wall space. Create a class called PaintCalculator with a main() method that prompts the user for the length, width, and height of a rectangular room, all three of type double. Pass these three values to a method called computeArea() that does the following: Calculates the wall area for a room Passes the calculated wall area to another method called computeGallons() that calculates and returns the number of gallons of paint needed Displays the number of gallons needed Computes the price based on a paint price of $32 per gallon, assuming that the painter can buy any fraction of a gallon of paint at the same price as a whole gallon Returns the price to the main() method The main() method displays the final price. For example, the cost to paint a 15-by-20-foot room with 10-foot ceilings is $64. An example of the program is shown below; Enter the room's length >> 13.2 Enter the room's width >> 10.5 Enter the room's height >> 9.0 You will need 1.2188571428571429 gallons The price to paint the room is $39.00342857142857 info> In order to complete the computeArea() method, you first need to implement the computeGallons() method. Task 01: Create the PaintCalculator class. Task 02: The computeGallons() method returns the gallons needed for an area. Task 03: The computeArea() method returns the price needed to paint a room. Task 04: The PaintCalculator program displays the number of gallons and the cost to paint a room.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Assume that a gallon of paint covers about 350 square feet of wall space. Create a class called PaintCalculator with a main() method that prompts the user for the length, width, and height of a rectangular room, all three of type double. Pass these three values to a method called computeArea() that does the following:
- Calculates the wall area for a room
- Passes the calculated wall area to another method called computeGallons() that calculates and returns the number of gallons of paint needed
- Displays the number of gallons needed
- Computes the price based on a paint price of $32 per gallon, assuming that the painter can buy any fraction of a gallon of paint at the same price as a whole gallon
- Returns the price to the main() method
The main() method displays the final price. For example, the cost to paint a 15-by-20-foot room with 10-foot ceilings is $64.
An example of the program is shown below;
Enter the room's length >> 13.2 Enter the room's width >> 10.5 Enter the room's height >> 9.0 You will need 1.2188571428571429 gallons The price to paint the room is $39.00342857142857
info> In order to complete the computeArea() method, you first need to implement the computeGallons() method.
![14
€03
C
> OUTLINE
> TIMELINE
Ö
> JAVA PROJECTS
* Codespaces: literate sniffle
Min X
EXPLORER
STUDENT [CODESPACES: LITERATE SNIFFLE]
J PaintCalculator.class
J PaintCalculator.java
Con X
template A0
187
108
https://literate-sniffle-x5wx9wg5x7w4fr5r.github.dev/?folder=/workspaces/9780357673423_java-programming-10e-5...
J PaintCalculator.java X
J PaintCalculator.java > PaintCalculator
104
105
106
109
110
111
112
113
114
Ans X
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129 }
Qinsu X
java X
double paintcost = calculateraintcoSE(CINSUTPAinty;
// Calculate VAT
double vat calculateVAT (paintCost);
// Calculate total cost
double totalCost = calculateTotalCost (paint Cost, vat);
// Prompt the user for payment
System.out.print("Enter the payment amount in dollars: ");
double payment = scanner.nextDouble();
// Calculate change
double change calculateChange (totalCost, payment);
form X
System.out.println("Paint Cost: $ + paintCost);
System.out.println("VAT: $" + vat);
System.out.println("Total Cost: $+ totalCost);
System.out.println("Change: $" + change);
scanner.close();
// Display the results
System.out.println("Wall Area: " + wallArea + " square meters");
System.out.println("Number of Tins of Paint: " + tinsOfPaint);
1 Java Lightweight Mode
New tab
chegg.co C chegg.co chegg.co debugthr C chegg.co
Q A" ☆ {} (D
B
student (Codespaces: literate sniffle)
T
"HELT WARRA
"THE
"UHCOMIN
www.a
"THE
MCHA
"THE
com
-
uns mom, men a
PUT
www.
en B
wa
T
D
LE
RE
m
= Companion X
You will need 1.2188571428571429 gallons
The price to paint the room is $39.00342857142857
Welcome
X
info> In order to complete the computeArea() method, you first need to implement
the computeGallons () method.
X
X
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL PORTS 1 COMMENTS
@juwen13663/2100231013463_java-pi ugi ammang-ave-20031JOL-LILO-4200-3026-313602363143/ L110plti 3/ CAD//SLUVCHIL (Lemplate) a
+
Task 01: Create the PaintCalculator class.
·[+]
Task 02: The computeGallons() method returns the gallons needed for an area.
Task 03: The computeArea() method returns the price needed to paint a room.
Task 04: The Paint Calculator program displays the number of gallons and the
cost to paint a room.
V
bash + I
用
•
*
0
08
...
X
Layout: US Q
0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F63639615-d990-48b7-9249-2331d859943f%2F6fef5cbe-8c75-490e-8df7-f426a1fbe0f0%2Fmmdfkws_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

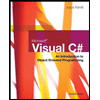
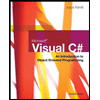