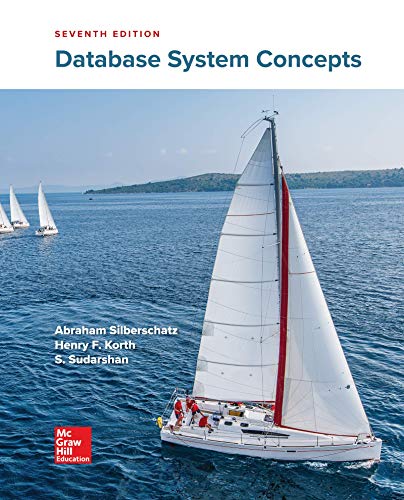
Write using beginner level JAVA!!!!
▪Create Sourdough subclass
- Unique Instance variable: lactic acid
- Constructors, getters, setters
- create 2 constructors – each utilizes the correct constructor
- for the default constructor, it is “1 tbs” of lactic acid and use “Sourdough” for breadName, the rest stay the same.
- create the getter and setter for the one unique attrib
- getUniqueIngredients returns a String showing the amount required for each unique attribute (hint: return this.lacticAcid + "something";)
- toString --- looks something like
Sourdough Bread Ingredients
5 cups of flour1.5 cups of water2.0 tbs of salt2.0 tbs of yeast // unique ingreds go after this line1 tbs lactic acid This bread is baked //This could be not baked
▪Create Tortilla subclass
–Unique Instance variables: masa
- Constructors, getters, setters
- create 2 constructors – each utilizes the correct constructor
- for the default constructor, it is “3.5 cups” of masa, use “Tortilla” for breadName, use “1 cup” for flour, the rest stay the same.
- create the getter and setter for the one unique attrib
- getUniqueIngredients returns a String showing the amount required for each unique attribute (hint: return this.masa + "something";)
- override the bake() method. Only change the baked value if masa is “3.5 cups”
- toString --- looks something like
Tortilla Ingredients
1 cup of flour
1.5 cups of water
2.0 tbs of salt
2.0 tbs of yeast
3.5 cups of masa
This Tortilla is not baked
You MUST utilize inheritance and reuse
Hint: attributes in superclasses should be protected, subclasses should be private
Hint: note where the keyword abstract is used.
I will supply a BreadTester – when you run it this is the results you should get:
Sourdough Ingredients
5 cups of flour
1.5 cups of water
2.0 tbs of salt
2.0 tbs of yeast
1 tbs of lactic acid
This Sourdough is baked ßNOTE – the bake method I called in line 25 in the Tester should have executed the bake method in Sourdough. There is no override in Sourdough, so the bake method in Bread code should run and should change this one from false to true!!
Tortilla Ingredients
1 cup of flour
1.5 cups of water
2.0 tbs of salt
2.0 tbs of yeast
3.5 cups of masa
This Tortilla is baked ßNOTE – the bake method override in Tortilla should change this one!!
Abuela's Tortillas Ingredients
4 cups of flour
2.5 cups of water
1 tsp of salt
3 tsp of yeast
2.75 cups of masa
This Abuela's Tortillas is not baked
Low Lactic Sourdough Ingredients
2 cups of flour
1.5 cups of water
3 tsp of salt
1 tsp of yeast
.05 tsp of lactic acid
This Low Lactic Sourdough is baked
Mama's Sourdough Ingredients
2 cups of flour
1.5 cups of water
3 tsp of salt
1 tsp of yeast
2 tbs of lactic acid
This Mama's Sourdough is baked ßNOTE – the bake method in Bread should change this one from false to true because Sourdough does not override the method!!
Low Salt Tortilla Ingredients
.5 cups of flour
1.5 cups of water
.05 tsp of salt
1 tsp of yeast
6.3 cups of masa
This Low Salt Tortilla is baked

Trending nowThis is a popular solution!
Step by stepSolved in 1 steps

- 1. Create the main method using pythone to test the classes. #Create the class personTypefrom matplotlib.pyplot import phase_spectrum class personType: #create the class constructor def __init__(self,fName,lName): #Initialize the data members self.fName = fName self.lName = lName #Method to access def getFName(self): return self.fName def getLName(self): return self.lName #Method to manipulate the data members def setFName(self,fName): self.fName = fName def setLName(self,lName): self.lName = lName #Create the class Doctor Type inherit from personTypeclass doctorType(personType): #Create the constructor for the doctorType class def __init__(self, fName, lName,speciality="unknown"): super().__init__(fName, lName) self.speciality = speciality #Methods to access def getSpeciality(self): return self.speciality #Methods to manipulate def setSpeciality(self,spc):…arrow_forward1 using static System.Console; 2 class ShapesDemo 3 { static void Main() { abstract class GeometricFigure Create an application named ShapesDemo that 4 creates several objects 5 that descend from an abstract class called 7 { GeometricFigure . Each 8 public int Height { 10 11 9 GeometricFigure get includes a height , a { 12 13 width , and an area. return height; } 14 15 Provide get and set set { 16 17 accessors for each field height = value; except area ; the area } 18 is computed and is read- } only. Include an abstract 19 public int Width 20 { 21 22 method called get ComputeArea() that { computes the area of the 23 24 return width; GeometricFigure . 25 26 27 28 29 set Next you will create three { width = value; additional classes derived from the } 30 } 31 GeometricFigure class. Name these derived classes: Rectange , 32 public int Area 33 { 34 Square , and Triangle. get 35 { 36 37 return area; } 38 } 39 40 private void CalArea() 41 { 42 43 } 44 area = Height * Width; 45 } 46arrow_forwardT/F 3. Any Java class must have a main method, which is the first method that is called when the Java class is invoked.arrow_forward
- Which of the following statements are true? Group of answer choices 1. A superclass reimplements the functionality of its subclasses. 2. A class that implements an interface reuses the functionality of the interface. 3. A derived class reuses the functionality instead of reimplements it. 4. If a class has no bugs, we can inherit from it without fear of having any bugs in our class.arrow_forwardTrue or False ___(1) An abstract class can have fields. ___(2) You can create an object from an abstract class _ _(3) An abstract class can have both abstract methods and methods that have method bodyarrow_forwardTrue or False ___(25) Java’s constructor name is exactly the same as the class name. ___(26) Java allows multiple constructors to be defined within a class. ___(27) is-a relationship is similar to has-a relationship in Java.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
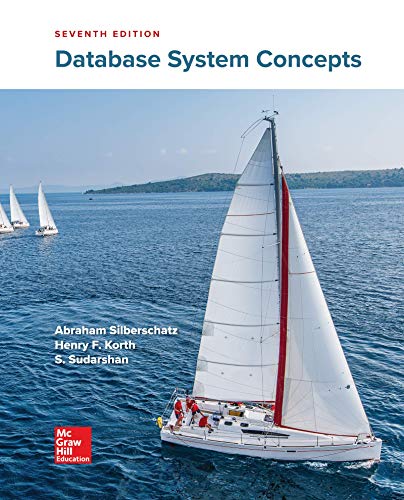
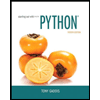
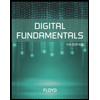
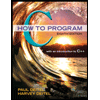
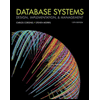
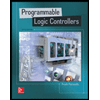