b) Write the method isBalanced, which returns true when the delimiters are balanced and returns false otherwise. The delimiters are balanced when both of the following conditions are satisfied; otherwise, they are not balanced. When traversing the ArrayList from the first element to the last element, there is no point at which there are more close delimiters than open delimiters at or before that point. The total number of open delimiters is equal to the total number of close delimiters. Consider a Delimiters object for which openDel is "" and closeDel is "". The examples below show different ArrayList objects that could be returned by calls to getDelimitersList and the value that would be returned by a call to isBalanced. Example 1 The following example shows an ArrayList for which isBalanced returns true. As tokens are examined from first to last, the number of open delimiters is always greater than or equal to the number of close delimiters. After examining all tokens, there are an equal number of open and close delimiters. "" "" "" "" "" "" Example 2 The following example shows an ArrayList for which isBalanced returns false. "" "" "" "" ↑ When starting from the left, at this point, condition 1 is violated. Example 3 The following example shows an ArrayList for which isBalanced returns false. "" ↑ At this point, condition 1 is violated. Example 4 The following example shows an ArrayList for which isBalanced returns false because the second condition is violated. After examining all tokens, there are not an equal number of open and close delimiters. "" "" "" Class information for this question public class Delimiters private String openDel private String closeDel public Delimiters(String open, String close) public ArrayList getDelimitersList(String[] tokens) public boolean isBalanced(ArrayList delimiters) Complete method isBalanced below. /** Returns true if the delimiters are balanced and false otherwise, as described in part (b). * Precondition: delimiters contains only valid open and close delimiters. */ public boolean isBalanced(ArrayList delimiters) ===================================================== import java.util.ArrayList; public class Delimiters { /** The open and close delimiters. */ private String openDel; private String closeDel; /** Constructs Delimiters object where open is the open delimiter and close is the * close delimiter. * Precondition: open and close are non-empty strings. */ public Delimiters(String open, String close) { openDel = open; closeDel = close; } /** Returns an ArrayList of delimiters from the array tokens, as described in part (a). */ public ArrayList getDelimitersList(String[] tokens){ /* to be implemented in part (a) */ } /** Returns true if the delimiters are balanced and false otherwise, as described in part (b). * Precondition: delimiters contains only valid open and close delimiters. */ public boolean isBalanced(ArrayList delimiters) { /* to be implemented in part (b) */ } } =============================== import java.util.ArrayList; public class DelimitersRunner { public static void main(String[] args) { //Test-1 Delimiters dl = new Delimiters("", ""); String[] arr = {"", "text", "", "in", "", "between", "the", "", "delimiters", "", ".", ""}; System.out.println(dl.isBalanced(dl.getDelimitersList(arr))); //Test-2 Delimiters dl2 = new Delimiters("<(>", "<)>"); String[] arr2 = {"<(>", "What", "is", "<)>", "this", "<)>", "<(>"}; System.out.println(dl2.isBalanced(dl2.getDelimitersList(arr2))); //Test-3 String[] arr3 = {""}; System.out.println(dl.isBalanced(dl.getDelimitersList(arr3))); //Test-4 String[] arr4 = {"", "", ""}; System.out.println(dl.isBalanced(dl.getDelimitersList(arr4))); } }
(b) Write the method isBalanced, which returns true when the delimiters are balanced and returns false otherwise. The delimiters are balanced when both of the following conditions are satisfied; otherwise, they are not balanced.
- When traversing the ArrayList from the first element to the last element, there is no point at which there are more close delimiters than open delimiters at or before that point.
- The total number of open delimiters is equal to the total number of close delimiters.
Consider a Delimiters object for which openDel is "<sup>" and closeDel is "</sup>".
The examples below show different ArrayList objects that could be returned by calls to
getDelimitersList and the value that would be returned by a call to isBalanced.
Example 1
The following example shows an ArrayList for which isBalanced returns true. As tokens are
examined from first to last, the number of open delimiters is always greater than or equal to the number of close delimiters. After examining all tokens, there are an equal number of open and close delimiters.
"<sup>" | "<sup>" | "</sup>" | "<sup>" | "</sup>" | "</sup>" |
Example 2
The following example shows an ArrayList for which isBalanced returns false.
"<sup>" | "</sup>" | "</sup>" | "<sup>" |
↑ |
When starting from the left, at this point, condition 1 is violated.
Example 3
The following example shows an ArrayList for which isBalanced returns false.
"</sup>" |
↑
At this point, condition 1 is violated.
Example 4
The following example shows an ArrayList for which isBalanced returns false because the
second condition is violated. After examining all tokens, there are not an equal number of open and close delimiters.
"<sup>" | "<sup>" | "</sup>" |
Class information for this question public class Delimiters private String openDel private String closeDel public Delimiters(String open, String close) public ArrayList<String> getDelimitersList(String[] tokens) public boolean isBalanced(ArrayList<String> delimiters) |
Complete method isBalanced below.
/** Returns true if the delimiters are balanced and false otherwise, as described in part (b). * Precondition: delimiters contains only valid open and close delimiters. */ public boolean isBalanced(ArrayList<String> delimiters)
=====================================================
import java.util.ArrayList;
public class Delimiters {
/** The open and close delimiters. */
private String openDel;
private String closeDel;
/** Constructs Delimiters object where open is the open delimiter and close is the
* close delimiter.
* Precondition: open and close are non-empty strings.
*/
public Delimiters(String open, String close) {
openDel = open;
closeDel = close;
}
/** Returns an ArrayList of delimiters from the array tokens, as described in part (a). */
public ArrayList<String> getDelimitersList(String[] tokens){
/* to be implemented in part (a) */
}
/** Returns true if the delimiters are balanced and false otherwise, as described in part (b).
* Precondition: delimiters contains only valid open and close delimiters.
*/
public boolean isBalanced(ArrayList<String> delimiters) {
/* to be implemented in part (b) */
}
}
===============================
import java.util.ArrayList;
public class DelimitersRunner {
public static void main(String[] args) {
//Test-1
Delimiters dl = new Delimiters("<p>", "</p>");
String[] arr = {"<p>", "text", "<p>", "in", "</p>", "between", "the", "<p>", "delimiters", "</p>", ".", "</p>"};
System.out.println(dl.isBalanced(dl.getDelimitersList(arr)));
//Test-2
Delimiters dl2 = new Delimiters("<(>", "<)>");
String[] arr2 = {"<(>", "What", "is", "<)>", "this", "<)>", "<(>"};
System.out.println(dl2.isBalanced(dl2.getDelimitersList(arr2)));
//Test-3
String[] arr3 = {"</p>"};
System.out.println(dl.isBalanced(dl.getDelimitersList(arr3)));
//Test-4
String[] arr4 = {"<p>", "<p>", "</p>"};
System.out.println(dl.isBalanced(dl.getDelimitersList(arr4)));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

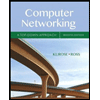
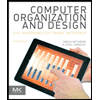
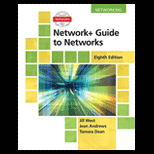
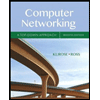
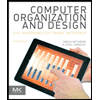
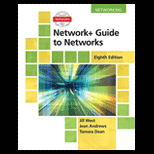
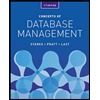
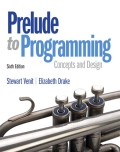
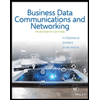