Please Use java only. Inthisassignment,youwillimplementaclasscalledCustomIntegerArrayList. Thisclass represents a fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods. For example, the CustomIntegerArrayList class has a “splice” method which removes a specified number of elements from the CustomIntegerArrayList, starting at a given index. For a CustomIntegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items). The Cu stomIntegerArrayList class has 2 different (overloaded) constructors. (Remember, an overloaded constructor is a constructor that has the same name, but a different number, type, or sequence of parameters, as another constructor in the class.) Having 2 different constructors means you can create an instance of the CustomIntegerArrayList class in 2 different ways, depending on which constructor you call. Both constructors have been implemented for you. (See below) Internally, the CustomIntegerArrayList class uses a private ArrayList variable named “arr” to store its integer elements. The “CustomIntegerArrayList()” constructor initializes the ArrayList variable “arr” as an empty ArrayList and the “CustomIntegerArrayList(ArrayList arr)” constructor initializes the ArrayList variable “arr” with the elements in the given ArrayList. (Again, see below) /** * Fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods. */ Introduction to Java & Object Oriented Programming public class CustomIntegerArrayList { // instance variables /** * Internal ArrayList of elements. */ private ArrayList arr; // constructors /** * Creates a new empty CustomIntegerArrayList. */ public CustomIntegerArrayList() { this.arr = new ArrayList(); } /** * Creates a new CustomIntegerArrayList with the elements in the given ArrayList. * @param arr with elements for the CustomIntegerArrayList */ public CustomIntegerArrayList(ArrayList arr) { this.arr = new ArrayList(arr); } There are 8 (mostly overloaded) methods that need to be implemented in the CustomIntegerArrayList c lass. (Again, an overloaded method is a method that has the same name, but a different number, type, or sequence of parameters, as another method in the same class.) ● getArrayList() - Returns the elements. ● get(int index) - Returns the element at the specified index from the elements. ● add(int element) - Appends the given element to the end of the elements. ● add(int index, int element) - Inserts the given element at the specified index. ● remove(int index) - Removes the element at the specified index. ● remove(int num, int element) - Removes the specified number of the given element from all elements. ● splice(int index, int num) - Removes the specified number of elements from all elements, starting at the given index. ● splice(int index, int num, int[] otherArray) - Removes the specified number of elements from all elements, starting at the given index, and inserts new elements in the given array at the given index. Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the method is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints and example method calls to help you get started. For example, we have defined a “remove” method for you (see below) which removes a specified number of a given element from the CustomIntegerArrayList. Read the javadoc, which explains what the method is supposed to do. Then write your code where it says “// TODO” to implement the method. You’ll do this for each method in the program. /** * Removes the specified number (num) of the given element from the internal ArrayList of elements. * If num lst1 = new ArrayList(); lst1.add(0, 2); lst1.add(0, 3); lst1.add(0, 4); lst1.remove(0); lst1.remove(1); assertEquals(arr1.get(0), lst1.get(0)); // TODO write at least 3 additional test cases } The main method calls have been provided for you (see example below). They are designed purely to call the other methods in the program, and to help you run the program. Nothing needs to be completed in the main method. public static void main(String args[]) { //create new empty CustomIntegerArrayList CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); //add element arr1.add(2); //get internal arraylist of elements System.out.println(arr1.getArrayList()); //[2] } You have been provided with CustomIntegerArrayList.java and CustomIntegerArrayListTest.java. To complete the assignment, implement the methods in CustomIntegerArrayList.java, making sure you pass all the tests in CustomIntegerArrayListTest.java. Then write at least 3 additional and distinct test cases for each unit test method in CustomIntegerArrayListTest.java. Do not modify the name of the methods in C ustomIntegerArrayList.java or the automated testing will not recognize it.
Please Use java only. Inthisassignment,youwillimplementaclasscalledCustomIntegerArrayList. Thisclass represents a fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods. For example, the CustomIntegerArrayList class has a “splice” method which removes a specified number of elements from the CustomIntegerArrayList, starting at a given index. For a CustomIntegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items). The Cu stomIntegerArrayList class has 2 different (overloaded) constructors. (Remember, an overloaded constructor is a constructor that has the same name, but a different number, type, or sequence of parameters, as another constructor in the class.) Having 2 different constructors means you can create an instance of the CustomIntegerArrayList class in 2 different ways, depending on which constructor you call. Both constructors have been implemented for you. (See below) Internally, the CustomIntegerArrayList class uses a private ArrayList variable named “arr” to store its integer elements. The “CustomIntegerArrayList()” constructor initializes the ArrayList variable “arr” as an empty ArrayList and the “CustomIntegerArrayList(ArrayList arr)” constructor initializes the ArrayList variable “arr” with the elements in the given ArrayList. (Again, see below) /** * Fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods. */ Introduction to Java & Object Oriented Programming public class CustomIntegerArrayList { // instance variables /** * Internal ArrayList of elements. */ private ArrayList arr; // constructors /** * Creates a new empty CustomIntegerArrayList. */ public CustomIntegerArrayList() { this.arr = new ArrayList(); } /** * Creates a new CustomIntegerArrayList with the elements in the given ArrayList. * @param arr with elements for the CustomIntegerArrayList */ public CustomIntegerArrayList(ArrayList arr) { this.arr = new ArrayList(arr); } There are 8 (mostly overloaded) methods that need to be implemented in the CustomIntegerArrayList c lass. (Again, an overloaded method is a method that has the same name, but a different number, type, or sequence of parameters, as another method in the same class.) ● getArrayList() - Returns the elements. ● get(int index) - Returns the element at the specified index from the elements. ● add(int element) - Appends the given element to the end of the elements. ● add(int index, int element) - Inserts the given element at the specified index. ● remove(int index) - Removes the element at the specified index. ● remove(int num, int element) - Removes the specified number of the given element from all elements. ● splice(int index, int num) - Removes the specified number of elements from all elements, starting at the given index. ● splice(int index, int num, int[] otherArray) - Removes the specified number of elements from all elements, starting at the given index, and inserts new elements in the given array at the given index. Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the method is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints and example method calls to help you get started. For example, we have defined a “remove” method for you (see below) which removes a specified number of a given element from the CustomIntegerArrayList. Read the javadoc, which explains what the method is supposed to do. Then write your code where it says “// TODO” to implement the method. You’ll do this for each method in the program. /** * Removes the specified number (num) of the given element from the internal ArrayList of elements. * If num lst1 = new ArrayList(); lst1.add(0, 2); lst1.add(0, 3); lst1.add(0, 4); lst1.remove(0); lst1.remove(1); assertEquals(arr1.get(0), lst1.get(0)); // TODO write at least 3 additional test cases } The main method calls have been provided for you (see example below). They are designed purely to call the other methods in the program, and to help you run the program. Nothing needs to be completed in the main method. public static void main(String args[]) { //create new empty CustomIntegerArrayList CustomIntegerArrayList arr1 = new CustomIntegerArrayList(); //add element arr1.add(2); //get internal arraylist of elements System.out.println(arr1.getArrayList()); //[2] } You have been provided with CustomIntegerArrayList.java and CustomIntegerArrayListTest.java. To complete the assignment, implement the methods in CustomIntegerArrayList.java, making sure you pass all the tests in CustomIntegerArrayListTest.java. Then write at least 3 additional and distinct test cases for each unit test method in CustomIntegerArrayListTest.java. Do not modify the name of the methods in C ustomIntegerArrayList.java or the automated testing will not recognize it.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please Use java only.
Inthisassignment,youwillimplementaclasscalledCustomIntegerArrayList. Thisclass represents a fancy ArrayList that stores integers and supports additional operations not included in Java's built-in ArrayList methods.
For example, the CustomIntegerArrayList class has a “splice” method which removes a specified number of elements from the CustomIntegerArrayList, starting at a given index. For a CustomIntegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items).
The Cu stomIntegerArrayList class has 2 different (overloaded) constructors. (Remember, an overloaded constructor is a constructor that has the same name, but a different number, type, or sequence of parameters, as another constructor in the class.) Having 2 different constructors means you can create an instance of the CustomIntegerArrayList class in 2 different ways, depending on which constructor you call. Both constructors have been implemented for you. (See below)
Internally, the CustomIntegerArrayList class uses a private ArrayList variable named “arr” to store its integer elements. The “CustomIntegerArrayList()” constructor initializes the ArrayList variable “arr” as an empty ArrayList and the “CustomIntegerArrayList(ArrayList arr)” constructor initializes the ArrayList variable “arr” with the elements in the given ArrayList. (Again, see below)
/**
* Fancy ArrayList that stores integers and supports additional
operations not included in Java's built-in ArrayList methods. */
Introduction to Java & Object Oriented Programming
public class CustomIntegerArrayList { // instance variables
/**
* Internal ArrayList of elements. */
private ArrayList arr;
// constructors
/**
* Creates a new empty CustomIntegerArrayList. */
public CustomIntegerArrayList() { this.arr = new ArrayList();
}
/**
* Creates a new CustomIntegerArrayList with the elements in
the given ArrayList.
* @param arr with elements for the CustomIntegerArrayList */
public CustomIntegerArrayList(ArrayList arr) {
this.arr = new ArrayList(arr); }
There are 8 (mostly overloaded) methods that need to be implemented in the CustomIntegerArrayList c lass. (Again, an overloaded method is a method that has the same name, but a different number, type, or sequence of parameters, as another method in the same class.)
● getArrayList() - Returns the elements.
● get(int index) - Returns the element at the specified index from the elements.
● add(int element) - Appends the given element to the end of the elements.
● add(int index, int element) - Inserts the given element at the specified index.
● remove(int index) - Removes the element at the specified index.
● remove(int num, int element) - Removes the specified number of the given element
from all elements.
● splice(int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
● splice(int index, int num, int[] otherArray) - Removes the specified number of elements
from all elements, starting at the given index, and inserts new elements in the given array at the given index.
Each method has been defined for you, but without the code. See the javadoc for each method for instructions on what the method is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints and example method calls to help you get started.
For example, we have defined a “remove” method for you (see below) which removes a specified number of a given element from the CustomIntegerArrayList. Read the javadoc, which explains what the method is supposed to do. Then write your code where it says “// TODO” to implement the method. You’ll do this for each method in the program.
/**
* Removes the specified number (num) of the given element from the
internal ArrayList of elements.
* If num lst1 = new ArrayList(); lst1.add(0, 2);
lst1.add(0, 3);
lst1.add(0, 4);
lst1.remove(0);
lst1.remove(1);
assertEquals(arr1.get(0), lst1.get(0));
// TODO write at least 3 additional test cases }
The main method calls have been provided for you (see example below). They are designed purely to call the other methods in the program, and to help you run the program. Nothing needs to be completed in the main method.
public static void main(String args[]) {
//create new empty CustomIntegerArrayList CustomIntegerArrayList arr1 = new CustomIntegerArrayList();
//add element arr1.add(2);
//get internal arraylist of elements
System.out.println(arr1.getArrayList()); //[2] }
You have been provided with CustomIntegerArrayList.java and CustomIntegerArrayListTest.java. To complete the assignment, implement the methods in CustomIntegerArrayList.java, making sure you pass all the tests in CustomIntegerArrayListTest.java. Then write at least 3 additional and distinct test cases for each unit test method in CustomIntegerArrayListTest.java. Do not modify the name of the methods in C ustomIntegerArrayList.java or the automated testing will not recognize it.
![• Unit Testing
The Assignment
In this assignment, you will implement a class called CustomintegerArrayList. This class
represents a fancy ArrayList that stores integers and supports additional operations not
included in Java's built-in ArrayList methods.
For example, the CustomintegerArrayList class has a "splice" method which removes a
specified number of elements from the CustomintegerArrayList, starting at a given index. For a
CustomintegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items
starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items).
The CustomintegerArrayList class has 2 different (overloaded) constructors. (Remember, an
overloaded constructor is a constructor that has the same name, but a different number, type,
or sequence of parameters, as another constructor in the class.) Having 2 different
constructors means you can create an instance of the CustomintegerArrayList class in 2
different ways, depending on which constructor you call. Both constructors have been
implemented for you. (See below)
Internally, the CustomintegerArrayList class uses a private ArrayList variable named "arr" to
store its integer elements. The "CustomlntegerArrayList()" constructor initializes the ArrayList
variable "arr" as an empty ArrayList and the "CustomintegerArrayList(ArrayList<Integer> arr)"
constructor initializes the ArrayList variable "arr" with the elements in the given ArrayList.
(Again, see below)
/**
* Fancy ArrayList that stores integers and supports additional
operations not included in Java's built-in ArrayList methods.
APenn
Engineering
ONLINE LEARNNG
Introduction to Java & Object Oriented Programming
public class CustomIntegerArrayList {
// instance variables
/**
* Internal ArrayList of elements.
*/
private ArrayList<Integer> arr;
// constructors
* Creates a new empty CustomintegerArrayList.
public CustomIntegerArrayList () {
this.arr - new ArrayList<Integer> ():
/**
* Creates a new CustomIntegerArrayList with the elements in
the given ArrayList.
* eparam arr with elements for the CustomIntegerArrayList
*/
public CustomIntegerArrayList (ArrayList<Integer> arr) {
this.arr - new ArrayList<Integer> (arr);
There are 8 (mostly overloaded) methods that need to be implemented in the
CustomintegerArrayList class. (Again, an overloaded method is a method that has the same
name, but a different number, type, or sequence of parameters, as another method in the same
class.)
• getArrayList() - Returns the elements.
• getlint index) - Returns the element at the specified index from the elements.
• addſint element) - Appends the given element to the end of the elements.
• addſint index, int element) - Inserts the given element at the specified index.
• remove(int index) - Removes the element at the specified index.
• remove(int num, int element) - Removes the specified number of the given element
from all elements.
• splice(int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
• splice(int index, int num, int] otherArray) - Removes the specified number of elements
from all elements, starting at the given index, and inserts new elements in the given
array at the given index.
Penn](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4946c40-85e7-4bdb-afce-1b8e9a9b2811%2F1158387a-d9b7-4761-952f-793c4701c939%2F2xj87uo_processed.jpeg&w=3840&q=75)
Transcribed Image Text:• Unit Testing
The Assignment
In this assignment, you will implement a class called CustomintegerArrayList. This class
represents a fancy ArrayList that stores integers and supports additional operations not
included in Java's built-in ArrayList methods.
For example, the CustomintegerArrayList class has a "splice" method which removes a
specified number of elements from the CustomintegerArrayList, starting at a given index. For a
CustomintegerArrayList that includes: 1, 2, 3, 4, and 5, calling splice(1, 2) will remove 2 items
starting at index 1. This will remove 2 and 3 (the 2nd and 3rd items).
The CustomintegerArrayList class has 2 different (overloaded) constructors. (Remember, an
overloaded constructor is a constructor that has the same name, but a different number, type,
or sequence of parameters, as another constructor in the class.) Having 2 different
constructors means you can create an instance of the CustomintegerArrayList class in 2
different ways, depending on which constructor you call. Both constructors have been
implemented for you. (See below)
Internally, the CustomintegerArrayList class uses a private ArrayList variable named "arr" to
store its integer elements. The "CustomlntegerArrayList()" constructor initializes the ArrayList
variable "arr" as an empty ArrayList and the "CustomintegerArrayList(ArrayList<Integer> arr)"
constructor initializes the ArrayList variable "arr" with the elements in the given ArrayList.
(Again, see below)
/**
* Fancy ArrayList that stores integers and supports additional
operations not included in Java's built-in ArrayList methods.
APenn
Engineering
ONLINE LEARNNG
Introduction to Java & Object Oriented Programming
public class CustomIntegerArrayList {
// instance variables
/**
* Internal ArrayList of elements.
*/
private ArrayList<Integer> arr;
// constructors
* Creates a new empty CustomintegerArrayList.
public CustomIntegerArrayList () {
this.arr - new ArrayList<Integer> ():
/**
* Creates a new CustomIntegerArrayList with the elements in
the given ArrayList.
* eparam arr with elements for the CustomIntegerArrayList
*/
public CustomIntegerArrayList (ArrayList<Integer> arr) {
this.arr - new ArrayList<Integer> (arr);
There are 8 (mostly overloaded) methods that need to be implemented in the
CustomintegerArrayList class. (Again, an overloaded method is a method that has the same
name, but a different number, type, or sequence of parameters, as another method in the same
class.)
• getArrayList() - Returns the elements.
• getlint index) - Returns the element at the specified index from the elements.
• addſint element) - Appends the given element to the end of the elements.
• addſint index, int element) - Inserts the given element at the specified index.
• remove(int index) - Removes the element at the specified index.
• remove(int num, int element) - Removes the specified number of the given element
from all elements.
• splice(int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
• splice(int index, int num, int] otherArray) - Removes the specified number of elements
from all elements, starting at the given index, and inserts new elements in the given
array at the given index.
Penn
![ONLINE LEARNING
Introduction to Java & Object Oriented Programming
Each method has been defined for you, but without the code. See the javadoc for each method
for instructions on what the method is supposed to do and how to write the code. It should be
clear enough. In some cases, we have provided hints and example method calls to help you
get started.
For example, we have defined a "remove" method for you (see below) which removes a
specified number of a given element from the CustomlntegerArrayList. Read the javadoc,
which explains what the method is supposed to do. Then write your code where it says ""
TODO" to implement the method. You'll do this for each method in the program.
/**
* Removes the specified number (num) of the given element from the
internal ArrayList of elements.
* If num <= 0, don't remove anything.
* If num is too large, remove all instances of the given element
from the internal ArrayList of elements.
* @param num number of instances of element to remove
* @param element to remove
*/
public void remove (int num, int element) {
// TODO Implement method
In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testRemovelnt" method for you (see below) which tests the
"remove" method. Pass the tests provided then write additional tests where it says "// TODO".
You'll do this for each unit test method in the program.
eTest
void testRemoveInt () {
CustomIntegerArrayList arrl - new CustomIntegerArrayList ();
arrl.add (0, 2):
arrl.add (0, 3):
arrl.add (0, 4);
arrl.remove (0):
arrl.remove (1):
Penn
Engineering
TONLINE LEARNING
Introduction to Java & Object Oriented Programming
ArrayList<Integer> 1stl = new ArrayList<Integer> () :
1stl.add (0, 2):
1st1.add (0, 3):
1st1.add (0, 4):
1stl.remove (0) :
1st1.remove (1):
assertEquals (arrl.get (0), 1stl.get (0)):
// TODO write at least 3 additional test cases
The main method calls have been provided for you (see example below). They are designed
purely to call the other methods in the program, and to help you run the program. Nothing
needs to be completed in the main method.
public static void main (String args(]) {
//create new empty CustomIntegerArrayList
CustomIntegerArrayList arrl ="new CustomIntegerArrayList ();
//add element
arrl.add (2):
//get internal arraylist of elements
System.out.printin (arrl.getArrayList ()): //12]
Submission
You have been provided with CustomintegerArrayListjava and
CustomintegerArrayListTest java. To complete the assignment, implement the methods in
CustomintegerArrayList.java, making sure you pass all the tests in
CustomintegerArrayListTest java. Then write at least 3 additional and distinct test cases for
each unit test method in CustomintegerArrayListTestjava. Do not modify the name of the
methods in CustomintegerArral.ist.iava or the automated testina will not recognize it.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4946c40-85e7-4bdb-afce-1b8e9a9b2811%2F1158387a-d9b7-4761-952f-793c4701c939%2Ftdsmpkv_processed.jpeg&w=3840&q=75)
Transcribed Image Text:ONLINE LEARNING
Introduction to Java & Object Oriented Programming
Each method has been defined for you, but without the code. See the javadoc for each method
for instructions on what the method is supposed to do and how to write the code. It should be
clear enough. In some cases, we have provided hints and example method calls to help you
get started.
For example, we have defined a "remove" method for you (see below) which removes a
specified number of a given element from the CustomlntegerArrayList. Read the javadoc,
which explains what the method is supposed to do. Then write your code where it says ""
TODO" to implement the method. You'll do this for each method in the program.
/**
* Removes the specified number (num) of the given element from the
internal ArrayList of elements.
* If num <= 0, don't remove anything.
* If num is too large, remove all instances of the given element
from the internal ArrayList of elements.
* @param num number of instances of element to remove
* @param element to remove
*/
public void remove (int num, int element) {
// TODO Implement method
In addition, you will write unit tests to test your method implementations. Each unit test
method has been defined for you, including some test cases. First make sure you pass all of
the provided tests, then write additional and distinct test cases for each unit test method.
For example, we have defined a "testRemovelnt" method for you (see below) which tests the
"remove" method. Pass the tests provided then write additional tests where it says "// TODO".
You'll do this for each unit test method in the program.
eTest
void testRemoveInt () {
CustomIntegerArrayList arrl - new CustomIntegerArrayList ();
arrl.add (0, 2):
arrl.add (0, 3):
arrl.add (0, 4);
arrl.remove (0):
arrl.remove (1):
Penn
Engineering
TONLINE LEARNING
Introduction to Java & Object Oriented Programming
ArrayList<Integer> 1stl = new ArrayList<Integer> () :
1stl.add (0, 2):
1st1.add (0, 3):
1st1.add (0, 4):
1stl.remove (0) :
1st1.remove (1):
assertEquals (arrl.get (0), 1stl.get (0)):
// TODO write at least 3 additional test cases
The main method calls have been provided for you (see example below). They are designed
purely to call the other methods in the program, and to help you run the program. Nothing
needs to be completed in the main method.
public static void main (String args(]) {
//create new empty CustomIntegerArrayList
CustomIntegerArrayList arrl ="new CustomIntegerArrayList ();
//add element
arrl.add (2):
//get internal arraylist of elements
System.out.printin (arrl.getArrayList ()): //12]
Submission
You have been provided with CustomintegerArrayListjava and
CustomintegerArrayListTest java. To complete the assignment, implement the methods in
CustomintegerArrayList.java, making sure you pass all the tests in
CustomintegerArrayListTest java. Then write at least 3 additional and distinct test cases for
each unit test method in CustomintegerArrayListTestjava. Do not modify the name of the
methods in CustomintegerArral.ist.iava or the automated testina will not recognize it.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
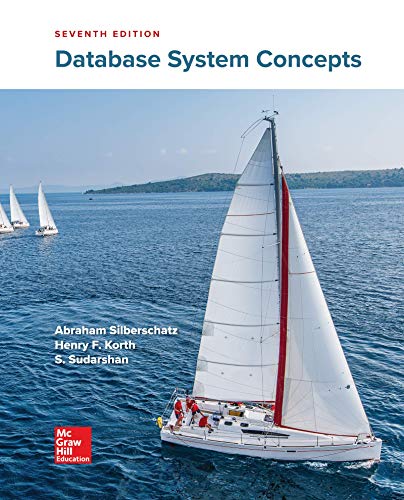
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
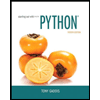
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
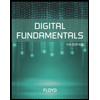
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
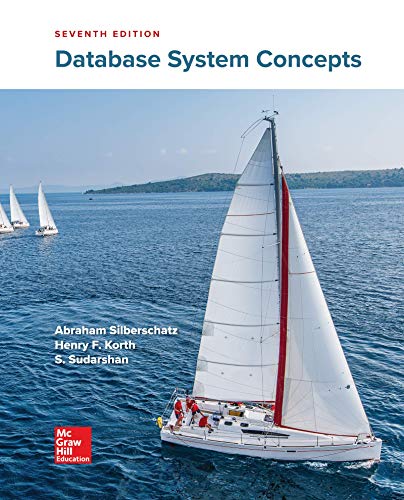
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
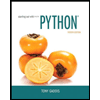
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
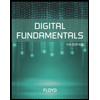
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
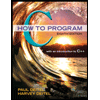
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
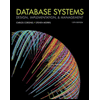
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
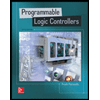
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education