// Only few methods are mentioned here //Do remaining methods to pass the test methods /** * Removes the specified (num) of the given element from the internal ArrayList of elements. * If num <= 0, don't remove anything. * If num is too large, remove all instances of the given element from the internal ArrayList of elements. * Example: * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1 * - Calling remove(2, 1) would remove the first 2 instances of 1 * - The CustomIntegerArrayList will then contain the integers: 2, 2, 1 * - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100 * - Calling remove(4, 100) would remove all instances of 100 * - The CustomIntegerArrayList will then be empty * - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5 * - Calling remove(0, 5) would remove nothing * * @param num number of instances of element to remove * @param element to remove */ public void remove(int num, int element) { // TODO } /** * Removes the specified number (num) of elements from the internal ArrayList of elements, starting at the given index. * If index < 0, don't remove anything and return an empty ArrayList. * If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything and return an empty ArrayList. * if num == 0, don't remove anything and return an empty ArrayList. * If the number of elements after the given index is less than the given num, * just remove the rest of the elements in the internal ArrayList. * Example: * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - Calling splice(1, 2) would remove 2 and 3 (the 2nd and 3rd items) * - The CustomIntegerArrayList will then contain the integers: 1, 4, 5 * and this method would return a new ArrayList containing the removed elements: 2 and 3 * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - In a call to splice(3, 4), the number of elements after the given index 3 is less than the given num 4 * - This would remove 4 and 5 (the 4th and 5th items) * - The CustomIntegerArrayList will then contain the integers: 1, 2, 3 * and this method would return a new ArrayList containing the removed elements: 4 and 5 * - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500 * - Calling splice(1, 0) would remove nothing * and this method would return a new empty ArrayList * @param index to start on * @param num of items to remove * @return ArrayList of removed elements */ public ArrayList splice(int index, int num) { // TODO return null; } /** * Removes the specified number (num) of elements from the internal ArrayList of elements, * starting at the given index, and inserts the elements in the given otherArray at the given index. * Uses the splice(int index, int num) method. * If index < 0, don't remove anything or insert anything, and return an empty ArrayList. * If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything or insert anything, * and return an empty ArrayList. * if num == 0, don't remove anything or insert anything, and return an empty ArrayList. * If the number of elements after the given index is less than the given num, * just remove the rest of the elements in the internal ArrayList. * Example: * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - Calling splice(1, 2, [6, 7]) would remove 2 and 3 (the 2nd and 3rd items), * and insert 6 and 7 at index 1. * - The CustomIntegerArrayList will then contain the integers: 1, 6, 7, 4, 5 * and this method would return a new ArrayList containing the removed elements: 2 and 3 * - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5 * - In a call to splice(3, 4, [1000, 1001]), the number of elements after the given index 3 is less than the given num 4 * - This would remove 4 and 5 (the 4th and 5th items) and insert 1000 and 1001 at index 3. * - The CustomIntegerArrayList will then contain the integers: 1, 2, 3, 1000, 1001 * and this method would return a new ArrayList containing the removed elements: 4 and 5 * - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500 * - Calling splice(1, 0, [700]) would remove nothing and insert nothing * and this method would return a new empty ArrayList * - For a defined CustomIntegerArrayList containing the integers: 5, 2, 7, 3, 7, 8 * - Calling splice(6, 3, [9]) would remove nothing and insert nothing * and this method would return a new empty ArrayList * @param index at which to remove and add the elements * @param num of elements to remove * @param otherArray of elements to add * @return ArrayList of removed elements */ public ArrayList splice(int index, int num, int[] otherArray) { // TODO return null; }
// Only few methods are mentioned here
//Do remaining methods to pass the test methods
/**
* Removes the specified (num) of the given element from the internal ArrayList of elements.
* If num <= 0, don't remove anything.
* If num is too large, remove all instances of the given element from the internal ArrayList of elements.
* Example:
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1
* - Calling remove(2, 1) would remove the first 2 instances of 1
* - The CustomIntegerArrayList will then contain the integers: 2, 2, 1
* - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100
* - Calling remove(4, 100) would remove all instances of 100
* - The CustomIntegerArrayList will then be empty
* - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5
* - Calling remove(0, 5) would remove nothing
*
* @param num number of instances of element to remove
* @param element to remove
*/
public void remove(int num, int element) {
// TODO
}
/**
* Removes the specified number (num) of elements from the internal ArrayList of elements, starting at the given index.
* If index < 0, don't remove anything and return an empty ArrayList.
* If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything and return an empty ArrayList.
* if num == 0, don't remove anything and return an empty ArrayList.
* If the number of elements after the given index is less than the given num,
* just remove the rest of the elements in the internal ArrayList.
* Example:
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - Calling splice(1, 2) would remove 2 and 3 (the 2nd and 3rd items)
* - The CustomIntegerArrayList will then contain the integers: 1, 4, 5
* and this method would return a new ArrayList containing the removed elements: 2 and 3
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - In a call to splice(3, 4), the number of elements after the given index 3 is less than the given num 4
* - This would remove 4 and 5 (the 4th and 5th items)
* - The CustomIntegerArrayList will then contain the integers: 1, 2, 3
* and this method would return a new ArrayList containing the removed elements: 4 and 5
* - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500
* - Calling splice(1, 0) would remove nothing
* and this method would return a new empty ArrayList
* @param index to start on
* @param num of items to remove
* @return ArrayList of removed elements
*/
public ArrayList<Integer> splice(int index, int num) {
// TODO
return null;
}
/**
* Removes the specified number (num) of elements from the internal ArrayList of elements,
* starting at the given index, and inserts the elements in the given otherArray at the given index.
* Uses the splice(int index, int num) method.
* If index < 0, don't remove anything or insert anything, and return an empty ArrayList.
* If index is too large (>= to the size of this CustomIntegerArrayList), don't remove anything or insert anything,
* and return an empty ArrayList.
* if num == 0, don't remove anything or insert anything, and return an empty ArrayList.
* If the number of elements after the given index is less than the given num,
* just remove the rest of the elements in the internal ArrayList.
* Example:
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - Calling splice(1, 2, [6, 7]) would remove 2 and 3 (the 2nd and 3rd items),
* and insert 6 and 7 at index 1.
* - The CustomIntegerArrayList will then contain the integers: 1, 6, 7, 4, 5
* and this method would return a new ArrayList containing the removed elements: 2 and 3
* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 3, 4, 5
* - In a call to splice(3, 4, [1000, 1001]), the number of elements after the given index 3 is less than the given num 4
* - This would remove 4 and 5 (the 4th and 5th items) and insert 1000 and 1001 at index 3.
* - The CustomIntegerArrayList will then contain the integers: 1, 2, 3, 1000, 1001
* and this method would return a new ArrayList containing the removed elements: 4 and 5
* - For a defined CustomIntegerArrayList containing the integers: 100, 200, 500
* - Calling splice(1, 0, [700]) would remove nothing and insert nothing
* and this method would return a new empty ArrayList
* - For a defined CustomIntegerArrayList containing the integers: 5, 2, 7, 3, 7, 8
* - Calling splice(6, 3, [9]) would remove nothing and insert nothing
* and this method would return a new empty ArrayList
* @param index at which to remove and add the elements
* @param num of elements to remove
* @param otherArray of elements to add
* @return ArrayList of removed elements
*/
public ArrayList<Integer> splice(int index, int num, int[] otherArray) {
// TODO
return null;
}
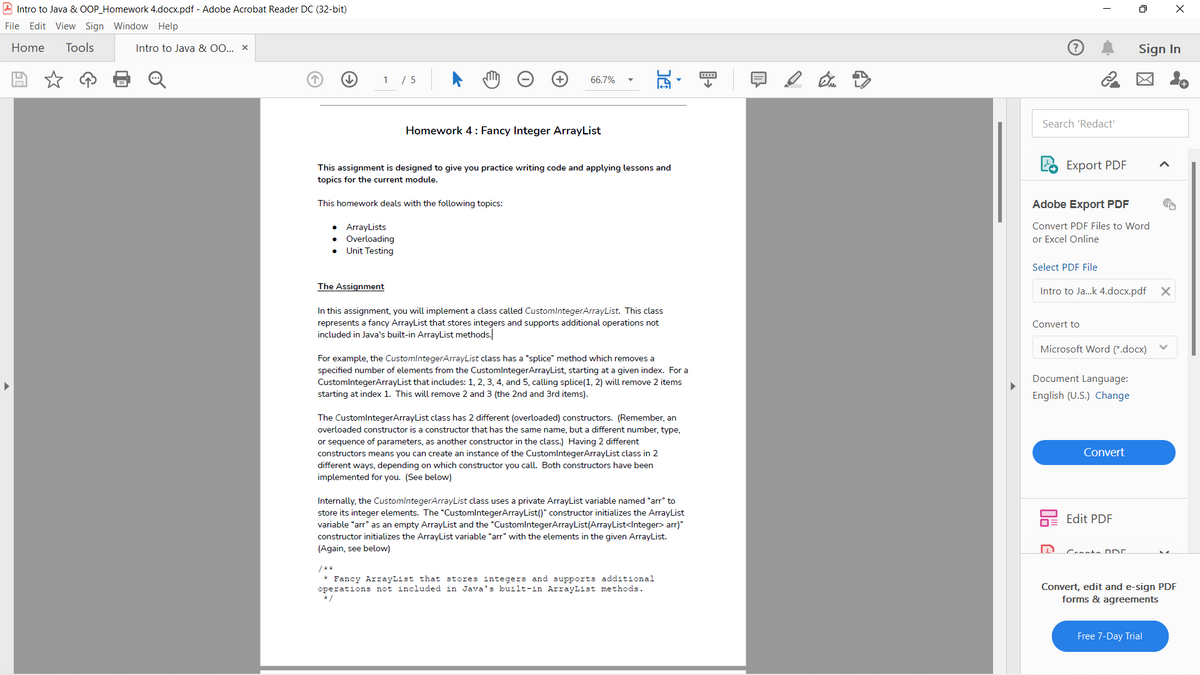
![A Intro to Java & OOP_Homework 4.docx.pdf - Adobe Acrobat Reader DC (32-bit)
File Edit View Sign Window Help
Home
Tools
Sign In
Intro to Java & 00... x
2 / 5
区 。
66.7%
Search 'Redact'
public class CustomIntegerArrayList {
// instance variables
Export PDF
/**
* Internal ArrayList of elements.
* /
private ArrayList<Integer> arr;
Adobe Export PDF
// constructors
Convert PDF Files to Word
or Excel Online
/**
* Creates a new empty CustomIntegerArrayList.
*/
Select PDF File
public CustomIntegerArrayList () {
this.arr = new ArrayList<Integer> (0;
Intro to Ja.k 4.docx.pdf X
}
/**
Convert to
a new CustomIntegerArrayList with the elements in
Creates
the given ArrayList.
Microsoft Word (*.docx)
@param arr with elements for the CustomIntegerArrayList
*
public CustomIntegerArrayList (ArrayList<Integer> arr) {
this.arr = new ArrayList<Integer> (arr);
Document Language:
English (U.S.) Change
There are 8 (mostly overloaded) methods that need to be implemented in the
CustomlntegerArrayList class. (Again, an overloaded method is a method that has the same
name, but a different number, type, or sequence of parameters, as another method in the same
class.)
Convert
getArrayList() - Returns the elements.
• get(int index) - Returns the element at the specified index from the elements.
add(int element) - Appends the given element to the end of the elements.
add(int index, int element) - Inserts the given element at the specified index.
remove(int index) - Removes the element at the specified index.
remove(int num, int element) - Removes the specified number of the given element
Edit PDF
from all elements.
Crante DDE.
splice(int index, int num) - Removes the specified number of elements from all
elements, starting at the given index.
splice(int index, int num, int[] otherArray) - Removes the specified number of elements
Convert, edit and e-sign PDF
forms & agreements
from all elements, starting at the given index, and inserts new elements in the given
array at the given index.
Free 7-Day Trial](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F86373622-039c-4af7-a380-0c111fcdd9a7%2Fad32a929-19b0-4543-b79e-8d2f533c4e2e%2Frsewali_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

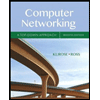
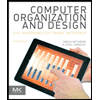
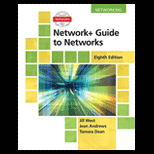
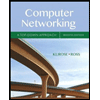
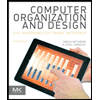
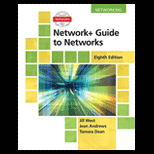
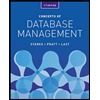
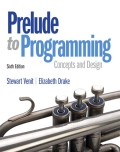
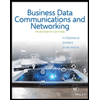