Building a Set Steps for Completion 1. Define the function list_to_set to take an argument of a list (the_list). 2. Within the list_to_set function, use another function to convert the list into a set. 3. Return the set. Snippet 6.90 shows an example of how the function list_to_set would work using the correct function: the_list = [2, 2, 4, 4, 4, 6, 8, 12, 10, 10] the_set=list_to_set(the_list) print (the_set) {2, 4, 6, 8, 10, 12} Snippet 6.90 Note: The example above is only showing the logic to what the correct function used within list_to_set will do. main.py + 1 # Write your code here 2 3 4 # Test your code with the following 5 if __name__ == '__main__': 6 print(list_to_set([2, 2, 4, 4, 4, 6, 8, 12, 10, 10])) 7
Scenario
Imagine that you have some data in a list that contains a lot of duplicates that you want to remove, but for simplicity’s sake, you want to avoid writing a for loop and building a new list.
Aim
The aim of this activity is to build a set out of a random set of items. Write a function called list_to_set that takes a list and turns it into a set. Do not use a for loop or while loop to do so.
![Building a Set
Steps for Completion
1. Define the function list_to_set to take an argument of a list (the_list).
2. Within the list_to_set function, use another function to convert the list into a set.
3. Return the set.
Snippet 6.90 shows an example of how the function list_to_set would work using the correct
function:
the_list = [2, 2, 4, 4, 4, 6, 8, 12, 10, 10]
the_set=list_to_set(the_list)
print (the_set)
{2, 4, 6, 8, 10, 12}
Snippet 6.90
Note: The example above is only showing the logic to what the correct function used within
list_to_set will do.
main.py
+
1 # Write your code here
2
3
4 # Test your code with the following
5 if __name__
== '__main__':
6
print (list_to_set([2, 2, 4, 4, 4,
6, 8, 12, 10, 10]))
7
↓](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5b13c2f-d38b-4c7e-8927-0b5918885d4c%2Fff209c98-0a7a-48f9-9595-cdd82517bcd2%2Fyxfx1ld_processed.png&w=3840&q=75)

Algorithm of the code:
1. Start
2. Initialize an empty dictionary
3. Iterate through the list of elements
4. For each element in the list, set it as the key in the dictionary
5. If the key already exists in the dictionary, skip it
6. Otherwise, add the key to the dictionary
7. Once the iteration has been completed, convert the dictionary to a set and return the set
8. end
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

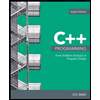
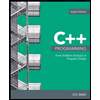