C++ assigment: It's a Number Guesser assignment. It asks to write a derived class of the NumberGuesser class named RandomNumberGuesser. The derived class should override the behavior of the getCurrentGuess method. It may also add member data and its own constructor. It may also override the higher(), lower() and reset() methods as you see fit. This is the code for NumberGuesser class. It is defined entirely in-line in the following file: NumberGuesser.h // NumberGuesser.h // #ifndef NUMBERGUESSER_H #define NUMBERGUESSER_H #include class NumberGuesser { protected: int low; int originalLow; int high; int originalHigh; public: NumberGuesser(int l, int h) { low = originalLow = l; high = originalHigh = h; } virtual int getCurrentGuess() { return (high + low) / 2; } virtual void higher() { low = getCurrentGuess() + 1; } virtual void lower() { high = getCurrentGuess() - 1; } virtual void reset() { low = originalLow; high = originalHigh; } }; #endif In the current NumberGuesser class the getCurrentGuess() method returns the midpoint of the range of possible values. In your RandomNumberGuesser class the getCurrentGuess() method should return a randomly generated number in the range of possible values. Note that repeated calls to getCurrentGuess() should always return the same value for both classes if neither the higher() or the lower() functions are called. Consider the following example: NumberGuesser *ng = new NumberGuesser(1, 10); cout << ng->getCurrentGuess(); cout << ng->getCurrentGuess(); cout << ng->getCurrentGuess(); Each invocation of getCurrentGuess should return 5. This value does not change until the higher() or lower() function is called. Now consider a RandomNumberGuesser example. The first call to getCurrentGuess() should return a random number between 1 and 10, inclusive. Each subsequent call to getCurrentGuess() should return the same number, until higher() or lower() is called, at which point a new random number within the new range should be generated. For example: NumberGuesser *ng = new RandomNumberGuesser(1, 10); // picks a random number between 1 and 10, let’s say it is 3, and outputs it. cout << ng->getCurrentGuess(); // this line prints out 3 again, because it is still the current guess cout << ng->getCurrentGuess(); // this line changes low to 4 because we now know the number is higher than 3. // high remains unchanged at 10. ng->higher(); // this line picks a random number between 4 and 10 and prints it out, // let’s say it is 9 cout << ng->getCurrentGuess(); // this line prints out 9 again, because it is still the current guess cout << ng->getCurrentGuess(); Make careful note of how the word “virtual” is used in NumberGuesser.h. There should be a RandomNumberGuesser.h and RandomNumberGuesser.cpp file at the end. Thanks
C++ assigment:
It's a Number Guesser assignment. It asks to write a derived class of the NumberGuesser class named RandomNumberGuesser. The derived class should override the behavior of the getCurrentGuess method. It may also add member data and its own constructor. It may also override the higher(), lower() and reset() methods as you see fit.
This is the code for NumberGuesser class. It is defined entirely in-line in the following file: NumberGuesser.h
// NumberGuesser.h
// #ifndef NUMBERGUESSER_H
#define NUMBERGUESSER_H
#include <iostream>
class NumberGuesser
{
protected:
int low;
int originalLow;
int high;
int originalHigh;
public:
NumberGuesser(int l, int h) {
low = originalLow = l; high = originalHigh = h;
}
virtual int getCurrentGuess() {
return (high + low) / 2;
}
virtual void higher() {
low = getCurrentGuess() + 1;
}
virtual void lower() {
high = getCurrentGuess() - 1;
}
virtual void reset() { low = originalLow; high = originalHigh;
}
};
#endif
In the current NumberGuesser class the getCurrentGuess() method returns the midpoint of the range of possible values. In your RandomNumberGuesser class the getCurrentGuess() method should return a randomly generated number in the range of possible values.
Note that repeated calls to getCurrentGuess() should always return the same value for both classes if neither the higher() or the lower() functions are called. Consider the following example:
NumberGuesser *ng = new NumberGuesser(1, 10);
cout << ng->getCurrentGuess();
cout << ng->getCurrentGuess();
cout << ng->getCurrentGuess();
Each invocation of getCurrentGuess should return 5. This value does not change until the higher() or lower() function is called.
Now consider a RandomNumberGuesser example. The first call to getCurrentGuess() should return a random number between 1 and 10, inclusive. Each subsequent call to getCurrentGuess() should return the same number, until higher() or lower() is called, at which point a new random number within the new range should be generated. For example:
NumberGuesser *ng = new RandomNumberGuesser(1, 10);
// picks a random number between 1 and 10, let’s say it is 3, and outputs it.
cout << ng->getCurrentGuess();
// this line prints out 3 again, because it is still the current guess
cout << ng->getCurrentGuess();
// this line changes low to 4 because we now know the number is higher than 3.
// high remains unchanged at 10.
ng->higher();
// this line picks a random number between 4 and 10 and prints it out,
// let’s say it is 9
cout << ng->getCurrentGuess();
// this line prints out 9 again, because it is still the current guess
cout << ng->getCurrentGuess();
Make careful note of how the word “virtual” is used in NumberGuesser.h.
There should be a RandomNumberGuesser.h and RandomNumberGuesser.cpp file at the end. Thanks

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

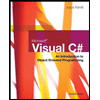
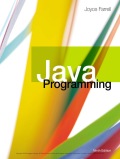
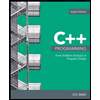
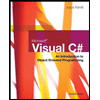
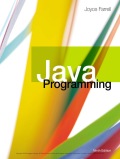
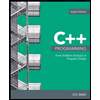