C++ code A simple version of snakes and ladder Implement a game that initially asks how many players will play the game. The game will then ask the name of each player. The game is played as follows: 1. The game is played iteratively. In every iteration, every player takes turn to roll a dice. The face value of dice is added to the total score of the player. 2. The first player to reach 100 score gets 1st position. The second player to reach 100 score gets 2 nd position. 3. When a player reaches 100, he no longer remains part of the play. If current score + face value is greater than 100, then the player will retry in the next iteration, i.e., the score must exactly become 100. 4. There are ladders at 20 and 60. If a player’s score becomes exactly 20, the score becomes 40. If a player’s score is exactly 60, the score becomes 75. Similarly, there are snakes at 50 and 90. If a player’s score is exactly 50, the score become 40. If a player’s score is exactly 90, the score becomes 79. 5. When n-1 players have reached 100, the game ends and the position of all players that participated in the game is displayed in ascending order. 6. During game play, some useful messages should be displayed, so that one can know what is going on in the game, such as: Please enter the number of players: 2 Please enter the name of Player 1: ABC Please enter the name of Player 2: XYZ It is ABC’s turn, please enter any key to continue. ABC got 5. The total score of ABC is now 5. It is XYZ’s turn, please enter any key to continue. XYZ got 6. The total score of XYZ is now 6. It is ABC’s turn, please enter any key to continue. ABC got 3. The total score of ABC is now 8. . . . It is XYZ’s turn, please enter any key to continue. XYZ got 4. The total score of XYZ is 50. There is a snake at 50, so total score is now 40. Use queue for this problem. You can use the queue class available in STL. You can only use push, pop, front, and empty functions of STL queue. Apart from queues, you cannot use any other data structures including arrays in any way (not even for storing the record of players that have already finished the game).
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++ code
A simple version of snakes and ladder
Implement a game that initially asks how many players will play the game. The game will then ask the
name of each player. The game is played as follows:
1. The game is played iteratively. In every iteration, every player takes turn to roll a dice. The face
value of dice is added to the total score of the player.
2. The first player to reach 100 score gets 1st position. The second player to reach 100 score gets
2
nd position.
3. When a player reaches 100, he no longer remains part of the play. If current score + face value is
greater than 100, then the player will retry in the next iteration, i.e., the score must exactly
become 100.
4. There are ladders at 20 and 60. If a player’s score becomes exactly 20, the score becomes 40. If
a player’s score is exactly 60, the score becomes 75. Similarly, there are snakes at 50 and 90. If a
player’s score is exactly 50, the score become 40. If a player’s score is exactly 90, the score
becomes 79.
5. When n-1 players have reached 100, the game ends and the position of all players that
participated in the game is displayed in ascending order.
6. During game play, some useful messages should be displayed, so that one can know what is
going on in the game, such as:
Please enter the number of players: 2
Please enter the name of Player 1: ABC
Please enter the name of Player 2: XYZ
It is ABC’s turn, please enter any key to continue.
ABC got 5. The total score of ABC is now 5.
It is XYZ’s turn, please enter any key to continue.
XYZ got 6. The total score of XYZ is now 6.
It is ABC’s turn, please enter any key to continue.
ABC got 3. The total score of ABC is now 8.
.
.
.
It is XYZ’s turn, please enter any key to continue.
XYZ got 4. The total score of XYZ is 50. There is a snake at 50, so total score is now 40.
Use queue for this problem. You can use the queue class available in STL. You can only use push,
pop, front, and empty functions of STL queue. Apart from queues, you cannot use any other data
structures including arrays in any way (not even for storing the record of players that have already
finished the game).

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

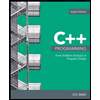
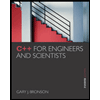
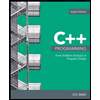
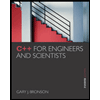