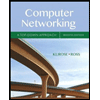
on a C++ program and using loops code this problem,
The game of "23" is a two-player game that begins with a pile of 23 toothpicks. Players take turns, withdrawing either 1, 2 or 3 toothpicks at a time. The player to withdraw the last toothpick loses the game. Write a human vs. computer program that plays "23". The human should always move first. When it is the computer's turn it should play according to the following rules:
-
-
- If there are more than 7 toothpicks left, then the computer should withdraw a random number of toothpicks (from 1 to 3). Use the rand() function, as demonstrated in class to produce this number..
- If there are 2, 3 or 4 toothpicks left, then the computer should withdraw enough toothpicks to leave 1.
- If there is 1 toothpick left, then the computer has to take it and loses.
-
When the human player enters the number of toothpicks to withdraw, the program should perform input validation. Make sure that the entered number is between 1 and 3 (inclusive) and that the player is not trying to withdraw more toothpicks than exist in the pile.Also while it runs it should look like,
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 3
20 toothpicks left
The computer picks 1
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 1
14 toothpicks left
The computer picks 3
11 toothpicks left
How many will you choose? (1, 2 or 3): 2
9 toothpicks left
The computer picks 2
7 toothpicks left
How many will you choose? (1, 2 or 3): 3
4 toothpicks left
The computer picks 3
1 toothpicks left
How many will you choose? (1, 2 or 3): 3
THE COMPUTER WINS!!!
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 2
21 toothpicks left
The computer picks 2
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 2
13 toothpicks left
The computer picks 2
11 toothpicks left
How many will you choose? (1, 2 or 3): 2
9 toothpicks left
The computer picks 2
7 toothpicks left
How many will you choose? (1, 2 or 3): 2
5 toothpicks left
The computer picks 2
3 toothpicks left
How many will you choose? (1, 2 or 3): 2
1 toothpicks left
THE HUMAN WINS!!!
Would you like to play 23? (y/n) y
23 toothpicks left
How many will you choose? (1, 2 or 3): 5
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 4
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 6
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): -3
Your pick was not valid
23 toothpicks left
How many will you choose? (1, 2 or 3): 3
20 toothpicks left
The computer picks 1
19 toothpicks left
How many will you choose? (1, 2 or 3): 2
17 toothpicks left
The computer picks 2
15 toothpicks left
How many will you choose? (1, 2 or 3): 2
13 toothpicks left
The computer picks 2
11 toothpicks left
How many will you choose? (1, 2 or 3): 1
10 toothpicks left
The computer picks 3
7 toothpicks left
How many will you choose? (1, 2 or 3): 1
6 toothpicks left
The computer picks 3
3 toothpicks left
How many will you choose? (1, 2 or 3): 3
THE COMPUTER WINS!!!
Would you like to play 23? (y/n) n

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a C# program that plays a guessing game with the user. Your program should select a random number between 1 and 50 and then let the user try to guess it. The program should continue asking the user for a number until he guesses correctly. (See below for some tips on random numbers). CHALLENGE #1: Modify your program so that it only allows the user 10 guesses, and then declares them to be an inadequate guesser if they haven’t gotten it correct. Your program should output the random number chosen. CHALLENGE #2: Modify your program so that after they guess a number (or get declared inadequate, if you do Challenge #1) that it asks them if they want to play again, and responds accordingly. Some Random Number Generation HintsRandom rndNumber = new Random();Console.WriteLine(rndNumber.Next()); //random integerConsole.WriteLine(rndNumber.Next(101)); //random integer between 0 and 100Console.WriteLine(rndNumber.Next(10, 43)); //random integer between 10 and…arrow_forwardin Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forwardStarting Out with Java From Control Structures through Objects 6th Edition Paint Job Estimatorarrow_forward
- Code in C++ Language As a cashier, you would always hate those times when customers choose to take back their order since you'd always summon your manager and yell, "Ma'am pa-void!" No matter how you hate it, you always have to do it since you have no other choice. Given (1) an array of prices of the items, (2) another array of the items the customer wishes to take back, and (3) the customer's payment, print the change of the customer. Input: The first number indicates the number of items "n" brought to the cashier. The next "n" numbers are the prices of the items. The next number indicates the number of items "m" that the customer wants to take back and not proceed. It is assured that 0 <= m <= n. The next "m" numbers are the items to be void that ranges from item 1 to item "n". The final number is the customer's payment. It is also assured that the given payment >= final price of items. INPUT: 5 103.65 650.95 10.25 1067.30 65.18 2 1 5 2000.00 Output: The change…arrow_forwardDesign a program that simulates the slot machine game. When the program starts, it should greet user and then start the game. For each game, the program asks user for the bet amount, and then randomly picks three words/images. If all the three words/images are same, user won three times of the bet amount, if any two of them are same, user won twice of the bet amount, otherwise, user won 0. The program should allow user to play as many games as wanted at one run. The program should display number of games user played, total amount bet and won at the end the program. It should also display each game's information, including game number, bet amount, the three words/images, and won amount. Here is the list of words you may use: Cherries, Oranges, Plums, Bells, Melons, and Bars. i need to do this in raptor flowchartarrow_forwardWrite a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forward
- Hello, This is part of my hangman simulation in C++. If you compile and run it and type "Easy," the code should run. If you run it though, the body of the hangman doesn't align when you guess wrong. Could you help me with that and implement an if statement to repeat the program if user wants to play again? #include <iostream> #include <cstdlib> #include <ctime> #include <string> #include <iomanip>using namespace std; const int MAX_TRIES = 5;char answer; int letterFill(char, string, string&); int main() { string name; char letter; int num_of_wrong_guesses = 0; string word; srand(time(NULL)); // ONLY NEED THIS ONCE! // welcome the user cout << "\n\nWelcome to hangman!! Guess a fruit that comes into your mind."; // Ask user for for Easy, Average, Hard string level; cout << "\nChoose a LEVEL(E - Easy, A - Average, H - Hard):" << endl; cin >> level; // compare level if (level == "Easy") {//put all the string inside…arrow_forwardUnderstanding ifStatements Summary In this lab, you complete a prewritten Java program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is made of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.java is open. 2. You need to declare variables for the following, and initialize them where specified: A variable for the cost of the sign initialized to 0.00 (charge). A variable for the number of characters initialized to 8 (numChars). A variable for the color of the characters initialized to "gold" (color). A variable for the…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
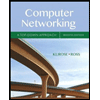
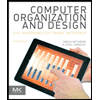
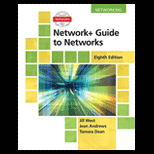
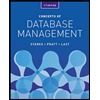
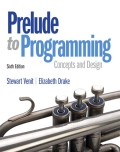
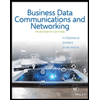