C++ Fix the broken code, Fix the function that is being used It does not charge the full hour for fractional portions. If you enter 4.5 it will return 27.50 as opposed to 30. Instructions to fix the code Consider this…. Charge will never be less than 20 so…… double charge = 20; for hours above 3…. If (hours >3) charge = 20 + ceil(hours -3)*5; where ceil brings it up to the next full hour value…. The charge will never be greater than 50 so….. If (charge > 50) charge = 50; now all you need to do is return charge Here is the code: #include #include using namespace std; /* This is a function that calculates the charge of parking for the given amount of time (in hours) */ double calculateCharges(double hours) { if (hours <= 3.0) { // If the time is less than 3 hours. return 20.0; // Charge a fixed amount of $20 } else { double charge = 20.0 + (hours - 3.0) * 5; // Calculate the charge, which is $20 + ($5/hour for excess of 3 hours) if (charge >= 50.0) { // If the charge is greater than $50 return 50.0; // Charge the maximum amount of $50 } return charge; } } int main() { // Declare the variables double hoursParked[3], // For storing the number of hours parked parkingCharges[3], // For storing the parking charges totalHours = 0, // For storing the total hours all cars were parked totalCharges = 0; // For storing the total charges of all cars for (int i = 1; i <= 3; i++) { // Prompt the user for input of hours a car was parked cout << "Enter the hours Car " << i << " was parked: "; cin >> hoursParked[i - 1]; parkingCharges[i - 1] = calculateCharges(hoursParked[i - 1]); // Calculate the parking charges totalCharges += parkingCharges[i - 1]; // Add charges to total totalHours += hoursParked[i - 1]; // Add hours to total } // Print the information header // Use setw() to set the width of the text. We have used a width of 10 in our case cout << setw(5) << "Car" << setw(10) << "Hours" << setw(10) << "Charge" << endl; for (int i = 1; i <= 3; i++) { // Display the values for each car cout << fixed << setw(5) << i << setw(10) << setprecision(1) << hoursParked[i - 1] << setw(10) << setprecision(2) << parkingCharges[i - 1] << "\n"; } // Display the total values cout << fixed << setw(5) << "TOTAL" << setw(10) << setprecision(1) << totalHours << setw(10) << setprecision(2) << totalCharges << "\n"; }
C++ Fix the broken code, Fix the function that is being used It does not charge the full hour for fractional portions. If you enter 4.5 it will return 27.50 as opposed to 30.
Instructions to fix the code
#include<iostream>
#include<iomanip>
using namespace std;
/* This is a function that calculates the charge of parking for the given amount of time (in hours) */
double calculateCharges(double hours) {
if (hours <= 3.0) { // If the time is less than 3 hours.
return 20.0; // Charge a fixed amount of $20
}
else {
double charge = 20.0 + (hours - 3.0) * 5; // Calculate the charge, which is $20 + ($5/hour for excess of 3 hours)
if (charge >= 50.0) { // If the charge is greater than $50
return 50.0; // Charge the maximum amount of $50
}
return charge;
}
}
int main() {
// Declare the variables
double hoursParked[3], // For storing the number of hours parked
parkingCharges[3], // For storing the parking charges
totalHours = 0, // For storing the total hours all cars were parked
totalCharges = 0; // For storing the total charges of all cars
for (int i = 1; i <= 3; i++) { // Prompt the user for input of hours a car was parked
cout << "Enter the hours Car " << i << " was parked: ";
cin >> hoursParked[i - 1];
parkingCharges[i - 1] = calculateCharges(hoursParked[i - 1]); // Calculate the parking charges
totalCharges += parkingCharges[i - 1]; // Add charges to total
totalHours += hoursParked[i - 1]; // Add hours to total
}
// Print the information header
// Use setw() to set the width of the text. We have used a width of 10 in our case
cout << setw(5) << "Car" << setw(10) << "Hours" << setw(10) << "Charge" << endl;
for (int i = 1; i <= 3; i++) {
// Display the values for each car
cout << fixed << setw(5) << i << setw(10) << setprecision(1) << hoursParked[i - 1] << setw(10) << setprecision(2) << parkingCharges[i - 1] << "\n";
}
// Display the total values
cout << fixed << setw(5) << "TOTAL" << setw(10) << setprecision(1) << totalHours << setw(10) << setprecision(2) << totalCharges << "\n";
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

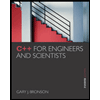
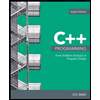
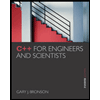
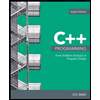