C++, How can I modify this code to have up to 6 players (can be 2,3,4,5,6 player depending on user)? Thank you #include #include #include using namespace std; #define DESTINATION 30 // define 30 as the winning position int dice() { srand(time(NULL)); // used for seeding in order to get // different random values each time return (rand()%6 +1); // since %6 gives 0 to 5, so add 1. } int snake(int n) { if (n == 17) return 4; else if (n == 19) return 7; else if (n == 21) return 9; else if (n == 27) return 1; } int ladder(int n) { if (n == 3) return 22; else if (n == 5) return 8; else if (n == 11) return 26; else if (n == 20) return 29; } int main () { int currentpos = 1; // user starts at 1 int value; // value on dice int count = 0; // to keep track of the number of dice rolls char choice; choice = 'N'; // by default consider choice as NO do { if (choice == 'Y' || choice == 'y') { value = dice(); count++; // increment the number of dice rolls cout << "The dice reads " << value <<".\n"; currentpos += value; if (currentpos > DESTINATION) { // check if current position is going beyond the destination cout << "Sorry! It\'s a very high number, Roll the dice again!\n"; currentpos -= value; // substract the previous value } else if (currentpos == 3 || currentpos == 5 || currentpos == 11 || currentpos == 20) { // check for ladder currentpos = ladder(currentpos); cout << "You are on a ladder to " << currentpos << "!! Yaay!\n"; } else if (currentpos == 17 || currentpos == 19 || currentpos == 21 || currentpos == 27) { // check for snake currentpos = snake(currentpos); cout << "Oops the snake just bit you!! Sorry, you are now at " << currentpos << ".\n"; } else if (currentpos == DESTINATION) { // check if destination has been reached cout << "Yaay!! You have successfully reached your destination and you took " << count << " tries to reach there.\nCongratulations.\nDo you want to play again? Enter ‘y’ or ‘Y’ to continue.\n"; currentpos = 0; // this ensures that if Y is entered, then game starts from place 0. cin >> choice; if (choice == 'N' || choice == 'n') // if user enters N, then exit break; } else cout << "You are now at " << currentpos << "!!!\n"; // this is used if ladder, snake and destination not encountered } cout << "Rolling the dice! Enter 'Y' or 'y' to roll!: "; cin >> choice; } while (choice == 'Y' || choice == 'y'); cout << "Goodbye!\n"; return 0;
C++,
How can I modify this code to have up to 6 players (can be 2,3,4,5,6 player depending on user)? Thank you
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
#define DESTINATION 30 // define 30 as the winning position
int dice() {
srand(time(NULL)); // used for seeding in order to get
// different random values each time
return (rand()%6 +1); // since %6 gives 0 to 5, so add 1.
}
int snake(int n) {
if (n == 17)
return 4;
else if (n == 19)
return 7;
else if (n == 21)
return 9;
else if (n == 27)
return 1;
}
int ladder(int n) {
if (n == 3)
return 22;
else if (n == 5)
return 8;
else if (n == 11)
return 26;
else if (n == 20)
return 29;
}
int main () {
int currentpos = 1; // user starts at 1
int value; // value on dice
int count = 0; // to keep track of the number of dice rolls
char choice;
choice = 'N'; // by default consider choice as NO
do {
if (choice == 'Y' || choice == 'y') {
value = dice();
count++; // increment the number of dice rolls
cout << "The dice reads " << value <<".\n";
currentpos += value;
if (currentpos > DESTINATION) { // check if current position is going beyond the destination
cout << "Sorry! It\'s a very high number, Roll the dice again!\n";
currentpos -= value; // substract the previous value
}
else if (currentpos == 3 || currentpos == 5 || currentpos == 11 || currentpos == 20) { // check for ladder
currentpos = ladder(currentpos);
cout << "You are on a ladder to " << currentpos << "!! Yaay!\n";
}
else if (currentpos == 17 || currentpos == 19 || currentpos == 21 || currentpos == 27) { // check for snake
currentpos = snake(currentpos);
cout << "Oops the snake just bit you!! Sorry, you are now at " << currentpos << ".\n";
}
else if (currentpos == DESTINATION) { // check if destination has been reached
cout << "Yaay!! You have successfully reached your destination and you took " << count << " tries to reach there.\nCongratulations.\nDo you want to play again? Enter ‘y’ or ‘Y’ to continue.\n";
currentpos = 0; // this ensures that if Y is entered, then game starts from place 0.
cin >> choice;
if (choice == 'N' || choice == 'n') // if user enters N, then exit
break;
}
else
cout << "You are now at " << currentpos << "!!!\n"; // this is used if ladder, snake and destination not encountered
}
cout << "Rolling the dice! Enter 'Y' or 'y' to roll!: ";
cin >> choice;
} while (choice == 'Y' || choice == 'y');
cout << "Goodbye!\n";
return 0;
}

Step by step
Solved in 2 steps with 1 images

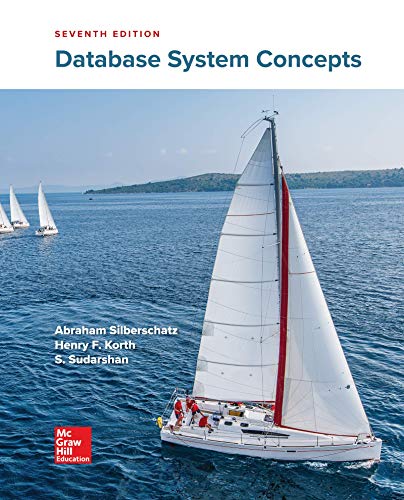
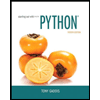
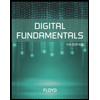
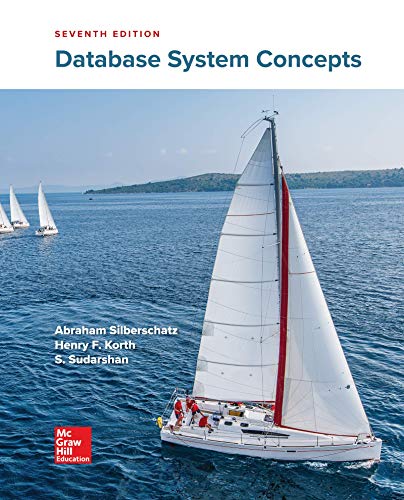
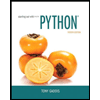
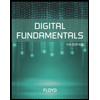
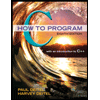
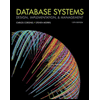
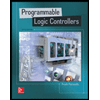