C++ Pet Class You are going to organize and show information about pets in a Pet Store using a Pet class. The Pet class You need to make a Pet class that holds the following information as private data members: the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters) the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters) level of hungriness of the pet (2 means hungry, 1 means content, 0 means full) Your class also needs to have the following functions: a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content” a parameterized constructor to allow having any type of pet the order of the parameters is: name of the pet, type of pet, hungriness level a PrintInfo function that prints the info for the pet according to the sample output below a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry). a FeedPet function that changes the hungry level of the pet to 0 (Full) You must write your class in separate .h and .cpp files. Sample Output For a default pet (dog, named “Buddy”, content): A dog named Buddy who is hungry level 1. For a pet cat named Fluffy, who is content:: A cat named Fluffy who is hungry level 1. For a hamster named Sparky who is full: A hamster named Sparky who is hungry level 0. I need to provide Main.cpp, Pet.h, and Pet.Cpp. I am given Main.cpp, constants for Pet.h. No information given for Pet.cpp. Main.cpp (no changes needed here!)- #include "Pet.h" int main() {Pet firstPet;Pet secondPet("Fluffy", "cat", CONTENT_HUNGRY); firstPet.PrintInfo(); secondPet.PrintInfo();secondPet.TimePasses();secondPet.PrintInfo();secondPet.FeedPet();secondPet.PrintInfo(); Pet thirdPet("Sparky", "hamster", NOT_HUNGRY);thirdPet.PrintInfo();return 0;} Pet.h: I must add to it but use these constants- const int NOT_HUNGRY = 0;const int VERY_HUNGRY = 2;const int CONTENT_HUNGRY = 1; Pet.cpp: No given information.
C++
Pet Class
You are going to organize and show information about pets in a Pet Store using a Pet class.
The Pet class
You need to make a Pet class that holds the following information as private data members:
- the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters)
- the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters)
- level of hungriness of the pet (2 means hungry, 1 means content, 0 means full)
Your class also needs to have the following functions:
- a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content”
- a parameterized constructor to allow having any type of pet
- the order of the parameters is: name of the pet, type of pet, hungriness level
- a PrintInfo function that prints the info for the pet according to the sample output below
- a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry).
- a FeedPet function that changes the hungry level of the pet to 0 (Full)
You must write your class in separate .h and .cpp files.
Sample Output
For a default pet (dog, named “Buddy”, content):
A dog named Buddy who is hungry level 1.
For a pet cat named Fluffy, who is content::
A cat named Fluffy who is hungry level 1.
For a hamster named Sparky who is full:
A hamster named Sparky who is hungry level 0.
I need to provide Main.cpp, Pet.h, and Pet.Cpp. I am given Main.cpp, constants for Pet.h. No information given for Pet.cpp.
Main.cpp (no changes needed here!)-
#include "Pet.h"
int main() {
Pet firstPet;
Pet secondPet("Fluffy", "cat", CONTENT_HUNGRY);
firstPet.PrintInfo();
secondPet.PrintInfo();
secondPet.TimePasses();
secondPet.PrintInfo();
secondPet.FeedPet();
secondPet.PrintInfo();
Pet thirdPet("Sparky", "hamster", NOT_HUNGRY);
thirdPet.PrintInfo();
return 0;
}
Pet.h:
I must add to it but use these constants-
const int NOT_HUNGRY = 0;
const int VERY_HUNGRY = 2;
const int CONTENT_HUNGRY = 1;
Pet.cpp:
No given information.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

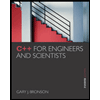
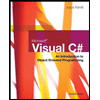
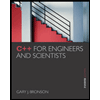
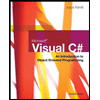